Python删除字符串中的标点符号可以通过多种方法实现,其中包括使用字符串方法、列表解析和正则表达式。常用的方法包括使用str.translate()方法、str.replace()方法和re模块。以下是详细的介绍和示例。
使用str.translate()方法
str.translate()方法是一个高效的删除字符的方法。我们需要创建一个翻译表,指明哪些字符需要删除。可以使用str.maketrans()方法创建这个翻译表。假设我们要删除所有的标点符号,可以使用以下步骤:
import string
def remove_punctuation(input_string):
translator = str.maketrans('', '', string.punctuation)
return input_string.translate(translator)
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们导入了string模块,该模块包含了一个标点符号列表。我们使用str.maketrans()方法创建一个翻译表,并将所有标点符号映射到None。然后,我们使用str.translate()方法应用这个翻译表,从而删除字符串中的所有标点符号。
使用str.replace()方法
str.replace()方法可以用于逐个替换字符串中的标点符号。虽然这种方法不如str.translate()方法高效,但它简单易懂,适合处理少量标点符号的情况。以下是使用str.replace()方法删除标点符号的示例:
def remove_punctuation(input_string):
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
for char in punctuation:
input_string = input_string.replace(char, "")
return input_string
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们定义了一个包含所有标点符号的字符串。然后,我们遍历这个字符串,并使用str.replace()方法将每个标点符号替换为空字符串,从而删除它们。
使用正则表达式(re模块)
正则表达式是一种强大的字符串处理工具,适合用于复杂的字符串处理任务。我们可以使用re.sub()方法通过正则表达式删除字符串中的标点符号。以下是使用正则表达式删除标点符号的示例:
import re
def remove_punctuation(input_string):
return re.sub(r'[^\w\s]', '', input_string)
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们使用re.sub()方法将正则表达式模式匹配的字符替换为空字符串。模式r'[^\w\s]'匹配所有非字母数字字符和非空白字符,从而删除所有标点符号。
一、str.translate()方法
str.translate()方法是一个高效的删除字符的方法。我们需要创建一个翻译表,指明哪些字符需要删除。可以使用str.maketrans()方法创建这个翻译表。假设我们要删除所有的标点符号,可以使用以下步骤:
import string
def remove_punctuation(input_string):
translator = str.maketrans('', '', string.punctuation)
return input_string.translate(translator)
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们导入了string模块,该模块包含了一个标点符号列表。我们使用str.maketrans()方法创建一个翻译表,并将所有标点符号映射到None。然后,我们使用str.translate()方法应用这个翻译表,从而删除字符串中的所有标点符号。
str.translate()方法的优势
str.translate()方法的一个显著优势是其高效性。相比于逐个替换标点符号的方法,str.translate()方法在处理大文本时性能更佳。特别是在需要删除大量标点符号的情况下,这种方法能够显著提升效率。
二、str.replace()方法
str.replace()方法可以用于逐个替换字符串中的标点符号。虽然这种方法不如str.translate()方法高效,但它简单易懂,适合处理少量标点符号的情况。以下是使用str.replace()方法删除标点符号的示例:
def remove_punctuation(input_string):
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
for char in punctuation:
input_string = input_string.replace(char, "")
return input_string
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们定义了一个包含所有标点符号的字符串。然后,我们遍历这个字符串,并使用str.replace()方法将每个标点符号替换为空字符串,从而删除它们。
str.replace()方法的优势
str.replace()方法的优势在于其简单易懂,适合处理少量标点符号的情况。对于初学者来说,这种方法容易理解和实现。此外,str.replace()方法在处理少量标点符号时性能也不错。
三、正则表达式(re模块)
正则表达式是一种强大的字符串处理工具,适合用于复杂的字符串处理任务。我们可以使用re.sub()方法通过正则表达式删除字符串中的标点符号。以下是使用正则表达式删除标点符号的示例:
import re
def remove_punctuation(input_string):
return re.sub(r'[^\w\s]', '', input_string)
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们使用re.sub()方法将正则表达式模式匹配的字符替换为空字符串。模式r'[^\w\s]'匹配所有非字母数字字符和非空白字符,从而删除所有标点符号。
正则表达式的优势
正则表达式的一个显著优势是其灵活性和强大功能。我们可以轻松地定义复杂的模式来匹配特定的字符或字符组合。此外,正则表达式在处理复杂字符串处理任务时非常高效。
四、其他方法
除了上述三种常用方法,还有一些其他方法可以用于删除字符串中的标点符号。例如,我们可以使用列表解析和filter()函数来实现这一任务。以下是使用列表解析删除标点符号的示例:
import string
def remove_punctuation(input_string):
return ''.join([char for char in input_string if char not in string.punctuation])
example_string = "Hello, world! This is a test string."
clean_string = remove_punctuation(example_string)
print(clean_string) # 输出 "Hello world This is a test string"
在这个例子中,我们使用列表解析遍历输入字符串,并将所有非标点符号字符加入到一个新的字符串中。最终,我们将这些字符连接成一个新的字符串,从而删除所有标点符号。
列表解析的优势
列表解析的一个显著优势是其简洁性和可读性。对于简单的字符串处理任务,列表解析是一种非常直观的实现方法。此外,列表解析在处理小规模数据时性能也不错。
五、总结
在Python中删除字符串中的标点符号有多种方法,包括str.translate()方法、str.replace()方法、正则表达式和列表解析等。每种方法都有其独特的优势和适用场景。
str.translate()方法适用于需要高效删除大量标点符号的情况,其高效性使其在处理大文本时表现出色。
str.replace()方法适用于处理少量标点符号的情况,其简单易懂的实现方式使其成为初学者的首选。
正则表达式适用于复杂的字符串处理任务,其灵活性和强大功能使其在处理复杂模式匹配时表现出色。
列表解析适用于简单的字符串处理任务,其简洁性和可读性使其成为处理小规模数据的理想选择。
选择适合的删除标点符号的方法取决于具体的需求和应用场景。在实际应用中,可以根据文本的规模、标点符号的数量和处理任务的复杂性,选择最合适的方法来删除字符串中的标点符号。
相关问答FAQs:
如何在Python中识别和处理字符串中的标点符号?
在Python中,可以使用内置的字符串方法和正则表达式来识别标点符号。使用string.punctuation
可以获取所有的标点字符,也可以利用re
模块中的正则表达式对字符串进行匹配和替换。这样可以有效地找出并处理字符串中的标点符号。
使用Python删除字符串中的标点符号是否会影响字符串的其他部分?
删除字符串中的标点符号只会影响标点本身,其他字符和空格不会受到影响。通过使用str.replace()
或正则表达式的re.sub()
方法,可以精确地移除指定的标点符号,而保留字母、数字和空格等内容,从而确保文本的其他部分保持完整。
有没有推荐的库或工具可以简化在Python中去除标点符号的操作?
可以使用string
模块和re
模块来简化这一过程。string
模块提供了一个便捷的string.punctuation
常量,列出了所有常见的标点符号。而re
模块则允许使用正则表达式快速找到并替换标点符号,极大地提高了操作的灵活性和效率。
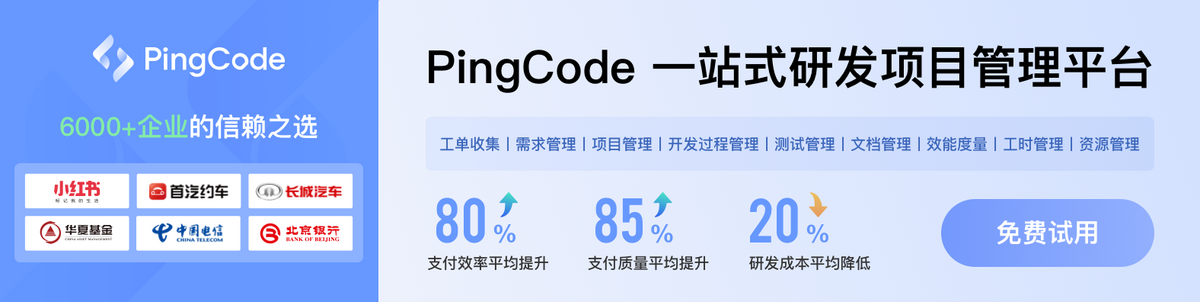