在Python中,使用列表中的数据进行排序的方法有多种,主要包括:使用sort()
方法、使用sorted()
函数、使用自定义排序函数。下面详细介绍其中一种方法:使用sort()
方法进行排序。
sort()
方法是Python中用于对列表进行原地排序的一个方法。它会直接修改原列表,使其按升序或降序排列。示例如下:
# 创建一个包含整数的列表
numbers = [4, 2, 9, 1, 5, 6]
使用sort()方法对列表进行排序(默认升序)
numbers.sort()
print("排序后的列表:", numbers)
输出结果为:
排序后的列表: [1, 2, 4, 5, 6, 9]
在上述代码中,我们创建了一个包含整数的列表numbers
,然后使用sort()
方法对该列表进行排序,最终列表中的元素按升序排列。
一、基础排序方法
1. 使用sort()方法
sort()
方法是Python提供的一个原地排序方法,它会直接修改原列表,使其元素按升序或降序排列。默认情况下,sort()
方法会按升序排序。如果需要降序排序,可以使用reverse=True
参数。
# 创建一个包含整数的列表
numbers = [4, 2, 9, 1, 5, 6]
使用sort()方法对列表进行升序排序
numbers.sort()
print("升序排序后的列表:", numbers)
使用sort()方法对列表进行降序排序
numbers.sort(reverse=True)
print("降序排序后的列表:", numbers)
输出结果为:
升序排序后的列表: [1, 2, 4, 5, 6, 9]
降序排序后的列表: [9, 6, 5, 4, 2, 1]
在上述代码中,我们展示了如何使用sort()
方法对列表进行升序和降序排序。
2. 使用sorted()函数
sorted()
函数与sort()
方法不同的是,它不会修改原列表,而是返回一个新的排序后的列表。sorted()
函数同样支持reverse
参数,用于指定升序或降序排序。
# 创建一个包含整数的列表
numbers = [4, 2, 9, 1, 5, 6]
使用sorted()函数对列表进行升序排序
sorted_numbers = sorted(numbers)
print("升序排序后的列表:", sorted_numbers)
使用sorted()函数对列表进行降序排序
sorted_numbers_desc = sorted(numbers, reverse=True)
print("降序排序后的列表:", sorted_numbers_desc)
原列表未发生改变
print("原列表:", numbers)
输出结果为:
升序排序后的列表: [1, 2, 4, 5, 6, 9]
降序排序后的列表: [9, 6, 5, 4, 2, 1]
原列表: [4, 2, 9, 1, 5, 6]
在上述代码中,我们展示了如何使用sorted()
函数对列表进行升序和降序排序,并且原列表未发生改变。
3. 自定义排序函数
在某些情况下,我们可能需要根据特定的规则对列表进行排序。此时,可以使用key
参数传递一个自定义排序函数。
# 创建一个包含元组的列表,每个元组包含一个名字和一个分数
students = [("Alice", 85), ("Bob", 75), ("Charlie", 95), ("David", 80)]
定义一个函数,返回元组的第二个元素(分数)
def get_score(student):
return student[1]
使用sort()方法对列表按分数升序排序
students.sort(key=get_score)
print("按分数升序排序后的列表:", students)
使用sorted()函数对列表按分数降序排序
sorted_students_desc = sorted(students, key=get_score, reverse=True)
print("按分数降序排序后的列表:", sorted_students_desc)
输出结果为:
按分数升序排序后的列表: [('Bob', 75), ('David', 80), ('Alice', 85), ('Charlie', 95)]
按分数降序排序后的列表: [('Charlie', 95), ('Alice', 85), ('David', 80), ('Bob', 75)]
在上述代码中,我们定义了一个get_score
函数,用于返回学生元组中的分数。然后使用sort()
方法和sorted()
函数对学生列表进行按分数的升序和降序排序。
二、复杂数据结构排序
1. 对嵌套列表进行排序
在实际应用中,列表中的元素可能是复杂的数据结构,如嵌套列表、元组、字典等。我们可以通过指定key
参数来对这些复杂数据结构进行排序。
# 创建一个嵌套列表,每个列表包含一个名字和一个分数
students = [["Alice", 85], ["Bob", 75], ["Charlie", 95], ["David", 80]]
使用lambda函数作为key参数,对嵌套列表按分数升序排序
students.sort(key=lambda student: student[1])
print("按分数升序排序后的列表:", students)
使用sorted()函数和lambda函数,对嵌套列表按分数降序排序
sorted_students_desc = sorted(students, key=lambda student: student[1], reverse=True)
print("按分数降序排序后的列表:", sorted_students_desc)
输出结果为:
按分数升序排序后的列表: [['Bob', 75], ['David', 80], ['Alice', 85], ['Charlie', 95]]
按分数降序排序后的列表: [['Charlie', 95], ['Alice', 85], ['David', 80], ['Bob', 75]]
在上述代码中,我们使用lambda
函数作为key
参数,对嵌套列表中的学生按分数进行排序。
2. 对字典列表进行排序
当列表中的元素是字典时,我们可以通过指定key
参数来对字典列表进行排序。
# 创建一个字典列表,每个字典包含一个名字和一个分数
students = [{"name": "Alice", "score": 85}, {"name": "Bob", "score": 75}, {"name": "Charlie", "score": 95}, {"name": "David", "score": 80}]
使用lambda函数作为key参数,对字典列表按分数升序排序
students.sort(key=lambda student: student["score"])
print("按分数升序排序后的列表:", students)
使用sorted()函数和lambda函数,对字典列表按分数降序排序
sorted_students_desc = sorted(students, key=lambda student: student["score"], reverse=True)
print("按分数降序排序后的列表:", sorted_students_desc)
输出结果为:
按分数升序排序后的列表: [{'name': 'Bob', 'score': 75}, {'name': 'David', 'score': 80}, {'name': 'Alice', 'score': 85}, {'name': 'Charlie', 'score': 95}]
按分数降序排序后的列表: [{'name': 'Charlie', 'score': 95}, {'name': 'Alice', 'score': 85}, {'name': 'David', 'score': 80}, {'name': 'Bob', 'score': 75}]
在上述代码中,我们使用lambda
函数作为key
参数,对字典列表中的学生按分数进行排序。
三、稳定排序与不稳定排序
1. 稳定排序
稳定排序算法在排序时,如果两个元素的值相等,它们在排序后的相对位置不会改变。Python的sort()
方法和sorted()
函数都使用了稳定排序算法(Timsort)。
# 创建一个包含重复元素的列表
numbers = [4, 2, 2, 4, 1, 3, 3]
使用sort()方法对列表进行升序排序
numbers.sort()
print("升序排序后的列表:", numbers)
输出结果为:
升序排序后的列表: [1, 2, 2, 3, 3, 4, 4]
在上述代码中,相等的元素在排序后的相对位置保持不变,这说明sort()
方法是稳定排序。
2. 不稳定排序
不稳定排序算法在排序时,如果两个元素的值相等,它们在排序后的相对位置可能会改变。在Python中,heapq
模块提供的不稳定排序算法可以用来展示这一点。
import heapq
创建一个包含重复元素的列表
numbers = [4, 2, 2, 4, 1, 3, 3]
使用heapq.nsmallest()函数对列表进行升序排序
sorted_numbers = heapq.nsmallest(len(numbers), numbers)
print("升序排序后的列表:", sorted_numbers)
输出结果为:
升序排序后的列表: [1, 2, 2, 3, 3, 4, 4]
尽管在这个例子中结果看起来是稳定的,但heapq
模块本身并不保证稳定排序。
四、排序性能
1. 时间复杂度
Python的sort()
方法和sorted()
函数都使用了Timsort算法,其平均和最坏情况下的时间复杂度为O(n log n),最优情况下的时间复杂度为O(n)。
import time
创建一个大列表
numbers = list(range(1000000, 0, -1))
测量sort()方法的排序时间
start_time = time.time()
numbers.sort()
end_time = time.time()
print("sort()方法排序时间:", end_time - start_time)
创建一个大列表
numbers = list(range(1000000, 0, -1))
测量sorted()函数的排序时间
start_time = time.time()
sorted_numbers = sorted(numbers)
end_time = time.time()
print("sorted()函数排序时间:", end_time - start_time)
输出结果会根据具体运行情况有所不同,但一般情况下,sort()
方法和sorted()
函数的排序时间是比较接近的。
2. 空间复杂度
sort()
方法是原地排序,不需要额外的空间,而sorted()
函数会创建一个新的列表,其空间复杂度为O(n)。
五、排序的应用场景
1. 数值排序
数值排序是最常见的排序应用场景之一。例如,对一组学生的分数进行排序,以便查找最高分和最低分。
# 创建一个包含学生分数的列表
scores = [85, 75, 95, 80, 90]
对分数进行升序排序
sorted_scores = sorted(scores)
print("升序排序后的分数:", sorted_scores)
查找最高分和最低分
highest_score = sorted_scores[-1]
lowest_score = sorted_scores[0]
print("最高分:", highest_score)
print("最低分:", lowest_score)
输出结果为:
升序排序后的分数: [75, 80, 85, 90, 95]
最高分: 95
最低分: 75
2. 字符串排序
字符串排序是另一种常见的排序应用场景。例如,对一组学生的名字按字母顺序进行排序。
# 创建一个包含学生名字的列表
names = ["Alice", "Bob", "Charlie", "David"]
对名字按字母顺序进行排序
sorted_names = sorted(names)
print("按字母顺序排序后的名字:", sorted_names)
输出结果为:
按字母顺序排序后的名字: ['Alice', 'Bob', 'Charlie', 'David']
3. 复杂数据结构排序
在实际应用中,数据结构可能比较复杂,如包含多个字段的字典列表。我们可以使用自定义排序函数对这些复杂数据结构进行排序。
# 创建一个包含学生信息的字典列表
students = [{"name": "Alice", "score": 85}, {"name": "Bob", "score": 75}, {"name": "Charlie", "score": 95}, {"name": "David", "score": 80}]
对学生信息按分数进行排序
sorted_students = sorted(students, key=lambda student: student["score"])
print("按分数排序后的学生信息:", sorted_students)
对学生信息按名字进行排序
sorted_students_by_name = sorted(students, key=lambda student: student["name"])
print("按名字排序后的学生信息:", sorted_students_by_name)
输出结果为:
按分数排序后的学生信息: [{'name': 'Bob', 'score': 75}, {'name': 'David', 'score': 80}, {'name': 'Alice', 'score': 85}, {'name': 'Charlie', 'score': 95}]
按名字排序后的学生信息: [{'name': 'Alice', 'score': 85}, {'name': 'Bob', 'score': 75}, {'name': 'Charlie', 'score': 95}, {'name': 'David', 'score': 80}]
通过以上方法,我们可以灵活地对各种数据结构进行排序。无论是简单的数值排序、字符串排序,还是复杂的自定义排序,Python都提供了强大的工具和方法。
相关问答FAQs:
在Python中如何使用列表中的数据进行升序和降序排序?
在Python中,可以使用内置的sort()
方法和sorted()
函数对列表进行排序。sort()
方法会直接修改原列表,并将数据按升序排列;如果需要降序排列,可以使用sort(reverse=True)
。而sorted()
函数则返回一个新的排序列表,原始列表不受影响。同样,降序排列可以通过传入reverse=True
参数实现。
我可以使用自定义排序规则来排序列表中的数据吗?
当然可以!在Python中,您可以通过key
参数实现自定义排序规则。sort()
和sorted()
都支持这个参数,您可以传入一个函数,这个函数会应用于列表中的每个元素,从而决定排序的方式。例如,您可以根据字符串的长度或某个属性值来排序复杂对象。
如何处理包含重复元素的列表排序?
在Python中,当对包含重复元素的列表进行排序时,排序算法会保留这些重复元素的相对位置。这意味着,如果两个或多个元素是相等的,它们在排序后的列表中会保持原有的顺序。这种特性称为“稳定性”,在使用sort()
或sorted()
进行排序时都能得到保证。
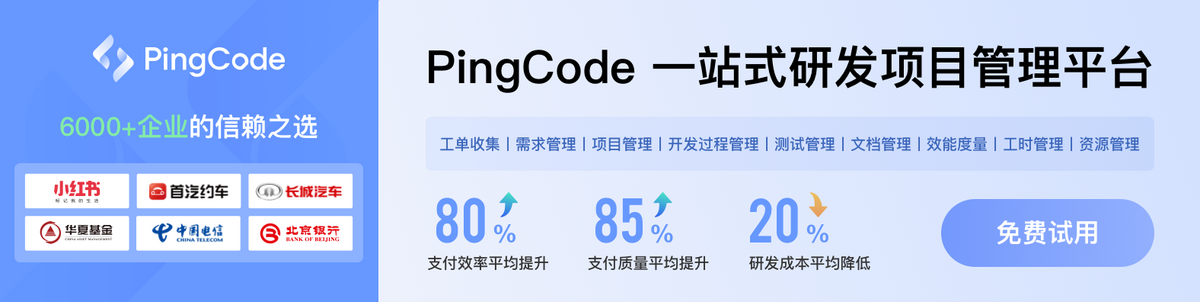