Python可以通过多种方式来将一段文字整体左移,包括使用字符串操作方法、正则表达式、以及文本处理库。具体方法有:逐行去除前导空格、使用正则表达式匹配空格、利用文本处理库。 其中,逐行去除前导空格的方法最为简单和直观,可以通过读取文件内容后逐行去除每行的前导空格来实现。
逐行去除前导空格是一种简单且有效的方法。首先,我们读取文件内容并将其存储在一个列表中,每个元素代表一行。然后,我们遍历列表,使用字符串的 lstrip()
方法去除每行的前导空格。最后,我们将处理后的内容重新写入文件或者输出到控制台。下面是一个示例代码:
def remove_leading_spaces(text):
lines = text.split('\n')
return '\n'.join(line.lstrip() for line in lines)
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
一、字符串操作方法
Python 提供了多种字符串操作方法,可以轻松地处理和修改文本内容。以下是一些常用的方法:
1、使用 lstrip()
方法
lstrip()
方法用于去除字符串左侧的空白字符。它可以有效地去除每行开头的空格或制表符,从而实现整体左移。
def remove_leading_spaces(text):
lines = text.split('\n')
return '\n'.join(line.lstrip() for line in lines)
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们首先将输入文本按行分割成一个列表,然后对每行应用 lstrip()
方法,最后将处理后的行重新组合成一个字符串。
2、使用 replace()
方法
replace()
方法用于替换字符串中的指定子字符串。我们可以使用它来替换每行开头的空格。
def remove_leading_spaces(text):
lines = text.split('\n')
return '\n'.join(line.replace(' ', '', 1) for line in lines)
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们使用 replace()
方法将每行开头的四个空格替换为空字符串。
二、正则表达式
正则表达式是一种强大的文本处理工具,可以用于复杂的字符串匹配和替换操作。Python 提供了 re
模块,用于处理正则表达式。
1、使用 re.sub()
方法
re.sub()
方法用于替换字符串中匹配正则表达式的部分。我们可以使用它来去除每行开头的空格。
import re
def remove_leading_spaces(text):
return re.sub(r'^\s+', '', text, flags=re.MULTILINE)
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们使用正则表达式 r'^\s+'
来匹配每行开头的空格,并将其替换为空字符串。flags=re.MULTILINE
参数用于在多行模式下匹配每行的开头。
三、文本处理库
Python 有许多强大的文本处理库,可以用于处理和修改文本内容。以下是一些常用的库:
1、使用 textwrap.dedent()
方法
textwrap
模块提供了多种文本处理功能,其中 dedent()
方法用于去除多行字符串的共同前导空格。
import textwrap
def remove_leading_spaces(text):
return textwrap.dedent(text)
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们使用 textwrap.dedent()
方法来去除每行开头的空格。
2、使用 pandas
库
pandas
是一个强大的数据处理库,通常用于处理结构化数据。我们可以使用它来处理文本数据。
import pandas as pd
def remove_leading_spaces(text):
df = pd.DataFrame(text.split('\n'), columns=['line'])
df['line'] = df['line'].str.lstrip()
return '\n'.join(df['line'])
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们首先将文本按行分割并存储在 pandas
DataFrame 中,然后使用 str.lstrip()
方法去除每行的前导空格,最后将处理后的行重新组合成一个字符串。
四、综合应用
在实际应用中,我们可以根据具体需求选择合适的方法,甚至可以结合多种方法来处理文本。以下是一个综合应用的示例:
import re
import textwrap
import pandas as pd
def remove_leading_spaces(text):
# 使用正则表达式去除每行开头的空格
text = re.sub(r'^\s+', '', text, flags=re.MULTILINE)
# 使用 textwrap.dedent() 方法去除多行字符串的共同前导空格
text = textwrap.dedent(text)
# 使用 pandas 库进一步处理文本
df = pd.DataFrame(text.split('\n'), columns=['line'])
df['line'] = df['line'].str.lstrip()
return '\n'.join(df['line'])
text = """ This is a line with leading spaces.
Another line with leading spaces.
Yet another line with leading spaces."""
print(remove_leading_spaces(text))
在这个示例中,我们首先使用正则表达式去除每行开头的空格,然后使用 textwrap.dedent()
方法去除多行字符串的共同前导空格,最后使用 pandas
库进一步处理文本。
总结
通过本文的介绍,我们了解了如何使用 Python 将一段文字整体左移,包括使用字符串操作方法、正则表达式、以及文本处理库。每种方法都有其优缺点和适用场景,我们可以根据具体需求选择合适的方法。逐行去除前导空格是最为简单和直观的方法,而正则表达式和文本处理库则提供了更为强大和灵活的处理能力。在实际应用中,我们可以结合多种方法来实现复杂的文本处理任务。
相关问答FAQs:
如何在Python中实现文本的左移操作?
在Python中,您可以使用字符串切片和拼接来实现文本的左移。举个例子,假设您有一段文字“Hello, World!”,如果想把它左移一个字符,可以这样做:
text = "Hello, World!"
left_shifted_text = text[1:] + text[0]
print(left_shifted_text) # 输出: ello, World!H
通过调整切片的起始和结束位置,可以实现不同的左移效果。
有没有现成的库可以实现文本的左移?
虽然Python标准库中没有专门的文本左移函数,但可以使用字符串操作和列表推导等方法实现。如果您希望使用更高级的功能,可以考虑第三方库如numpy
来处理大规模文本数据,尽管其主要用于数值计算。
在处理多行文本时,如何左移每一行?
在处理多行文本时,可以将每一行视作一个字符串,然后对每一行进行左移操作。可以使用循环遍历每一行,示例代码如下:
multi_line_text = """Hello, World!
Welcome to Python.
Enjoy coding!"""
left_shifted_lines = [line[1:] + line[0] for line in multi_line_text.splitlines()]
result = "\n".join(left_shifted_lines)
print(result)
这样可以确保每一行都被正确地左移。
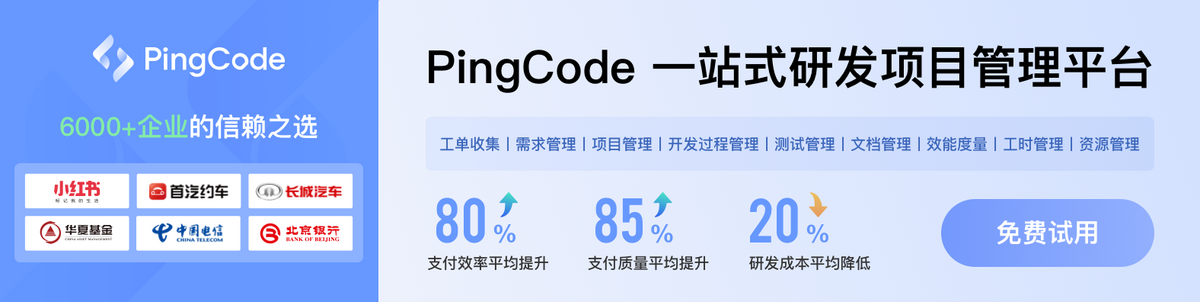