在Python中,写入一个TeX文件夹可以通过os模块来创建目录,并使用open()函数来写入文件。使用os模块创建目录、使用open()函数写入文件。以下是详细描述其中一个步骤:在写入文件之前,可以检查目录是否存在,如果不存在,可以使用os.makedirs()函数来创建。
一、创建目录
要写入TeX文件,首先需要确保目标目录存在。如果目录不存在,可以使用os.makedirs()函数创建目录。以下是一个示例代码:
import os
def create_directory(path):
if not os.path.exists(path):
os.makedirs(path)
else:
print("Directory already exists")
示例:创建一个名为'tex_files'的文件夹
create_directory('tex_files')
在上述代码中,create_directory()函数用于检查目录是否存在。如果目录不存在,则创建该目录。
二、写入文件
在创建目录之后,可以使用Python的内置open()函数来写入TeX文件。以下是一个示例代码:
def write_to_tex_file(directory, filename, content):
file_path = os.path.join(directory, filename)
with open(file_path, 'w') as file:
file.write(content)
示例:写入一个名为'sample.tex'的文件
tex_content = r"""
\documentclass{article}
\begin{document}
Hello, TeX!
\end{document}
"""
write_to_tex_file('tex_files', 'sample.tex', tex_content)
在上述代码中,write_to_tex_file()函数接受目录、文件名和内容作为参数,并将内容写入指定目录中的文件。
三、检查文件内容
写入文件之后,可以检查文件是否已经正确写入。以下是一个示例代码:
def read_tex_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
示例:读取'sample.tex'文件的内容
file_path = 'tex_files/sample.tex'
tex_content = read_tex_file(file_path)
print(tex_content)
在上述代码中,read_tex_file()函数读取指定文件的内容并返回。
四、附加内容到现有文件
有时候可能需要向现有TeX文件中附加内容。可以使用open()函数的'a'模式来附加内容。以下是一个示例代码:
def append_to_tex_file(directory, filename, content):
file_path = os.path.join(directory, filename)
with open(file_path, 'a') as file:
file.write(content)
示例:附加内容到'sample.tex'文件
additional_content = r"""
\section{Introduction}
This is an introduction section.
"""
append_to_tex_file('tex_files', 'sample.tex', additional_content)
在上述代码中,append_to_tex_file()函数附加指定内容到现有的文件中。
五、使用模板生成TeX文件
在生成TeX文件时,使用模板可以使代码更加整洁和易于维护。以下是一个示例代码:
from string import Template
def generate_tex_file_from_template(directory, filename, template_content, substitutions):
file_path = os.path.join(directory, filename)
template = Template(template_content)
tex_content = template.substitute(substitutions)
with open(file_path, 'w') as file:
file.write(tex_content)
示例:使用模板生成'sample.tex'文件
template_content = r"""
\documentclass{article}
\begin{document}
Hello, $name!
\end{document}
"""
substitutions = {'name': 'TeX'}
generate_tex_file_from_template('tex_files', 'sample.tex', template_content, substitutions)
在上述代码中,generate_tex_file_from_template()函数使用字符串模板和替换字典生成TeX文件。
六、处理异常情况
在实际应用中,处理异常情况是非常重要的。以下是一个示例代码:
def safe_write_to_tex_file(directory, filename, content):
try:
create_directory(directory)
write_to_tex_file(directory, filename, content)
print("File written successfully")
except Exception as e:
print(f"An error occurred: {e}")
示例:安全地写入'sample.tex'文件
safe_write_to_tex_file('tex_files', 'sample.tex', tex_content)
在上述代码中,safe_write_to_tex_file()函数在写入文件时处理可能的异常情况,并打印错误信息。
七、总结
通过以上步骤,可以在Python中轻松地创建TeX文件夹并写入TeX文件。使用os模块创建目录、使用open()函数写入文件、使用模板生成TeX文件、处理异常情况,这些技巧可以帮助你在处理TeX文件时更加高效和可靠。希望以上内容对你有所帮助。
相关问答FAQs:
如何在Python中创建新的tex文件?
在Python中,可以使用内置的open()
函数来创建一个新的.tex文件。只需指定文件名和模式为'w'
(写入模式),例如:
with open('example.tex', 'w') as file:
file.write('\\documentclass{article}\n')
file.write('\\begin{document}\n')
file.write('Hello, LaTeX!\n')
file.write('\\end{document}\n')
上述代码将创建一个名为example.tex
的文件,并写入基本的LaTeX文档结构。
如何在已有的tex文件中追加内容?
如果需要在已有的.tex文件中追加内容,可以将打开模式设为'a'
(追加模式)。这样可以在文件末尾添加新内容,而不会覆盖原有内容。例如:
with open('example.tex', 'a') as file:
file.write('\\section{Introduction}\n')
file.write('This is the introduction section.\n')
这段代码将向example.tex
文件中添加一个新的部分而不删除之前的内容。
如何确保写入tex文件时格式正确?
为了确保.tex文件的格式正确,可以使用Python的多行字符串(triple quotes)来编写LaTeX代码,这样可以提高可读性。还可以利用Python的字符串格式化功能,将变量动态插入到文档中。示例如下:
title = "Sample Document"
content = "This is a sample document with Python and LaTeX."
latex_code = f"""\documentclass{{article}}
\\title{{{title}}}
\\begin{{document}}
\\maketitle
{content}
\\end{{document}}"""
with open('sample.tex', 'w') as file:
file.write(latex_code)
这种方法可以使生成的LaTeX文档更清晰且易于管理。
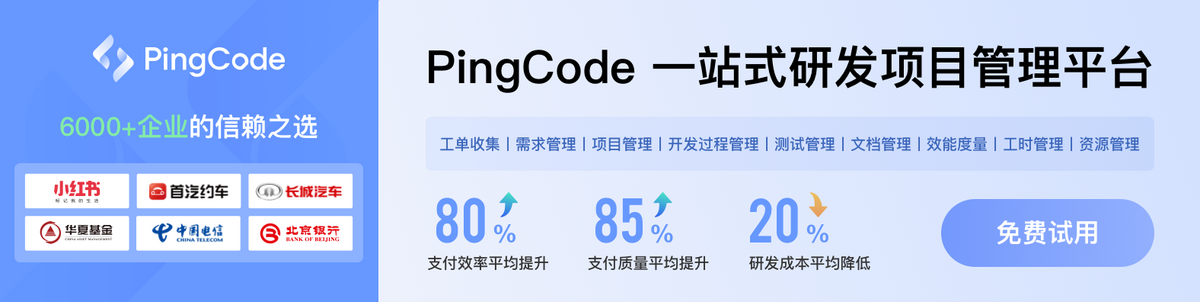