使用Python查找代码中的关键字可以通过多种方法来实现,包括使用正则表达式、字符串操作和内置的模块等方法。最常用的方法是使用正则表达式来精确匹配关键字、使用字符串操作来遍历和匹配关键字、使用内置的're'模块来处理复杂的模式匹配。 其中,正则表达式是最强大和灵活的方法之一。下面将详细介绍如何使用这些方法来查找代码中的关键字,并提供一些实际的代码示例。
一、使用字符串操作查找关键字
字符串操作是最简单直接的方法。Python提供了一些内置的字符串方法,可以轻松查找关键字。
1.1 使用'str.find()'方法
str.find()
方法返回字符串中第一次出现的子字符串的索引,如果找不到子字符串则返回-1。
code = "def my_function():\n print('Hello, World!')\n"
keyword = "print"
index = code.find(keyword)
if index != -1:
print(f"Keyword '{keyword}' found at index {index}")
else:
print(f"Keyword '{keyword}' not found")
1.2 使用'str.count()'方法
str.count()
方法返回子字符串在字符串中出现的次数。
code = "def my_function():\n print('Hello, World!')\n"
keyword = "print"
count = code.count(keyword)
print(f"Keyword '{keyword}' found {count} times")
1.3 使用'in'操作符
in
操作符可以用来检查子字符串是否存在于字符串中。
code = "def my_function():\n print('Hello, World!')\n"
keyword = "print"
if keyword in code:
print(f"Keyword '{keyword}' found in the code")
else:
print(f"Keyword '{keyword}' not found in the code")
二、使用正则表达式查找关键字
正则表达式是处理字符串匹配和查找的强大工具。Python的're'模块提供了对正则表达式的支持。
2.1 使用're.search()'方法
re.search()
方法用于搜索字符串中的模式。如果找到匹配项,则返回匹配对象;否则,返回None。
import re
code = "def my_function():\n print('Hello, World!')\n"
pattern = r"print"
match = re.search(pattern, code)
if match:
print(f"Keyword '{pattern}' found at index {match.start()}")
else:
print(f"Keyword '{pattern}' not found")
2.2 使用're.findall()'方法
re.findall()
方法返回字符串中所有非重叠匹配项的列表。
import re
code = "def my_function():\n print('Hello, World!')\n"
pattern = r"print"
matches = re.findall(pattern, code)
print(f"Keyword '{pattern}' found {len(matches)} times")
2.3 使用're.finditer()'方法
re.finditer()
方法返回一个迭代器,生成匹配对象。
import re
code = "def my_function():\n print('Hello, World!')\n"
pattern = r"print"
matches = re.finditer(pattern, code)
for match in matches:
print(f"Keyword '{pattern}' found at index {match.start()}")
三、使用内置模块查找关键字
Python有一些内置模块可以用来查找代码中的关键字。例如,ast
模块可以将Python代码解析为抽象语法树,从而更精确地查找关键字。
3.1 使用'ast'模块
ast
模块可以解析Python代码并生成抽象语法树,可以用来查找特定类型的节点。
import ast
code = """
def my_function():
print('Hello, World!')
"""
class KeywordVisitor(ast.NodeVisitor):
def __init__(self, keyword):
self.keyword = keyword
self.found = False
def visit_Name(self, node):
if node.id == self.keyword:
self.found = True
self.generic_visit(node)
tree = ast.parse(code)
visitor = KeywordVisitor('print')
visitor.visit(tree)
if visitor.found:
print(f"Keyword 'print' found in the code")
else:
print(f"Keyword 'print' not found in the code")
3.2 使用'tokenize'模块
tokenize
模块提供了一个生成器函数,可以将Python代码分解成标记(token)。
import tokenize
from io import StringIO
code = """
def my_function():
print('Hello, World!')
"""
keyword = 'print'
found = False
tokens = tokenize.generate_tokens(StringIO(code).readline)
for token in tokens:
if token.type == tokenize.NAME and token.string == keyword:
found = True
break
if found:
print(f"Keyword '{keyword}' found in the code")
else:
print(f"Keyword '{keyword}' not found in the code")
四、综合应用
在实际应用中,可能需要结合多种方法来查找代码中的关键字。以下是一个综合应用的示例:
4.1 使用多种方法查找关键字
import re
import ast
import tokenize
from io import StringIO
def find_keyword_in_code(code, keyword):
# Using string operations
if keyword in code:
print(f"(String operations) Keyword '{keyword}' found in the code")
else:
print(f"(String operations) Keyword '{keyword}' not found in the code")
# Using regular expressions
pattern = re.compile(rf"\b{keyword}\b")
matches = pattern.findall(code)
if matches:
print(f"(Regular expressions) Keyword '{keyword}' found {len(matches)} times in the code")
else:
print(f"(Regular expressions) Keyword '{keyword}' not found in the code")
# Using AST module
class KeywordVisitor(ast.NodeVisitor):
def __init__(self, keyword):
self.keyword = keyword
self.found = False
def visit_Name(self, node):
if node.id == self.keyword:
self.found = True
self.generic_visit(node)
tree = ast.parse(code)
visitor = KeywordVisitor(keyword)
visitor.visit(tree)
if visitor.found:
print(f"(AST module) Keyword '{keyword}' found in the code")
else:
print(f"(AST module) Keyword '{keyword}' not found in the code")
# Using tokenize module
found = False
tokens = tokenize.generate_tokens(StringIO(code).readline)
for token in tokens:
if token.type == tokenize.NAME and token.string == keyword:
found = True
break
if found:
print(f"(Tokenize module) Keyword '{keyword}' found in the code")
else:
print(f"(Tokenize module) Keyword '{keyword}' not found in the code")
Example usage
code = """
def my_function():
print('Hello, World!')
"""
keyword = "print"
find_keyword_in_code(code, keyword)
通过结合使用字符串操作、正则表达式、ast
模块和tokenize
模块,可以更全面地查找代码中的关键字。每种方法都有其优势,可以根据具体需求选择合适的方法或组合使用多种方法以确保结果的准确性和全面性。
总结:使用Python查找代码中的关键字有多种方法,包括字符串操作、正则表达式和内置模块(如ast
和tokenize
)。这些方法各有优缺点,可以根据具体需求选择合适的方法,或结合多种方法以确保查找结果的全面性和准确性。
相关问答FAQs:
如何在Python代码中查找特定的关键字?
您可以使用Python的内置功能和库来查找代码中的关键字。最常用的方法是使用in
关键字来检查字符串中是否包含特定的子字符串。此外,使用正则表达式(re
模块)可以提供更复杂的匹配模式。如果您需要在多个文件中查找关键字,可以考虑使用os
模块来遍历文件系统,并在每个文件中应用上述查找方法。
是否有工具可以自动查找Python代码中的关键字?
是的,有许多工具可以帮助您自动查找代码中的关键字。例如,使用文本编辑器(如VSCode或PyCharm)内置的搜索功能,可以快速查找整个项目中的关键字。此外,命令行工具如grep
也非常强大,可以在终端中进行快速查找。
如何优化Python代码查找性能?
优化查找性能的方法包括限制搜索的文件范围,例如只在特定目录或文件类型中进行搜索。使用索引工具(如Whoosh
或Elasticsearch
)可以加快搜索速度,尤其是在处理大型代码库时。此外,避免在每次查找时都读取整个文件,而是将文件内容缓存,以减少IO操作的开销。
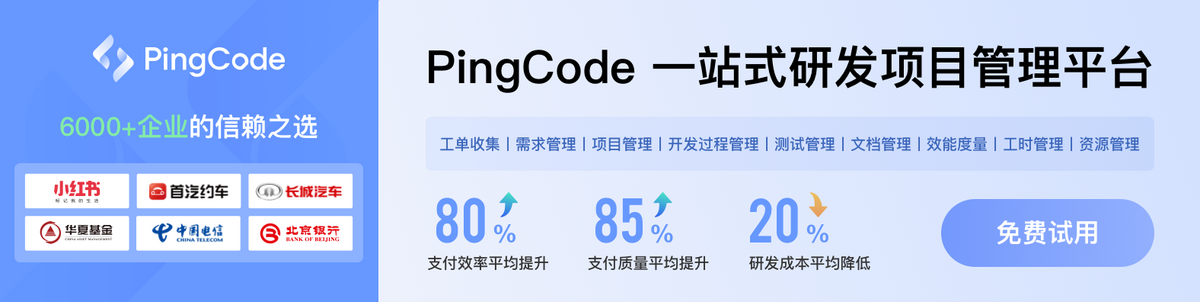