Python爬取金考典的题
要使用Python爬取金考典的题,关键步骤包括:选择合适的爬虫库、处理网页请求、解析网页内容、存储抓取的数据。 其中,选择合适的爬虫库是最重要的一步,可以选择如requests、BeautifulSoup、Scrapy等工具,本文将详细解释如何使用这些工具来实现爬虫任务。
一、选择合适的爬虫库
1.1 requests库
requests库是一个简单易用的HTTP请求库,可以用于发送HTTP请求,获取网页内容。其优点是语法简洁,易于上手。安装requests库可以使用以下命令:
pip install requests
使用requests库发送GET请求,获取网页内容的基本代码如下:
import requests
url = 'http://www.example.com'
response = requests.get(url)
print(response.text)
1.2 BeautifulSoup库
BeautifulSoup库是一个用于解析HTML和XML文档的库,可以方便地提取网页中的数据。安装BeautifulSoup库可以使用以下命令:
pip install beautifulsoup4
使用BeautifulSoup库解析网页内容的基本代码如下:
from bs4 import BeautifulSoup
html_doc = '<html><head><title>The Dormouse's story</title></head><body><p class="title"><b>The Dormouse's story</b></p></body></html>'
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title.string)
1.3 Scrapy库
Scrapy库是一个功能强大的爬虫框架,适用于大型爬虫项目。安装Scrapy库可以使用以下命令:
pip install scrapy
使用Scrapy库创建一个爬虫项目的基本步骤如下:
scrapy startproject myproject
cd myproject
scrapy genspider myspider example.com
二、处理网页请求
2.1 发送HTTP请求
使用requests库发送HTTP请求,获取网页内容的基本代码如下:
import requests
url = 'http://www.example.com'
response = requests.get(url)
if response.status_code == 200:
print('Request successful')
print(response.text)
else:
print('Request failed')
2.2 处理响应数据
获取网页内容后,可以使用BeautifulSoup库解析网页内容,提取所需数据。基本代码如下:
from bs4 import BeautifulSoup
url = 'http://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.string)
三、解析网页内容
3.1 提取网页元素
使用BeautifulSoup库解析网页内容,提取所需的网页元素,如标题、段落、链接等。基本代码如下:
from bs4 import BeautifulSoup
html_doc = '<html><head><title>The Dormouse's story</title></head><body><p class="title"><b>The Dormouse's story</b></p></body></html>'
soup = BeautifulSoup(html_doc, 'html.parser')
提取标题
title = soup.title.string
print('Title:', title)
提取段落
paragraph = soup.p.text
print('Paragraph:', paragraph)
提取链接
for link in soup.find_all('a'):
print('Link:', link.get('href'))
3.2 处理复杂网页结构
对于复杂的网页结构,可以使用BeautifulSoup库的各种查找方法,如find、find_all、select等,来提取所需的数据。基本代码如下:
from bs4 import BeautifulSoup
html_doc = '''<html>
<head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>'''
soup = BeautifulSoup(html_doc, 'html.parser')
使用find方法查找元素
title = soup.find('title').string
print('Title:', title)
使用find_all方法查找元素
links = soup.find_all('a')
for link in links:
print('Link:', link.get('href'))
使用select方法查找元素
paragraph = soup.select('p.story')[0].text
print('Paragraph:', paragraph)
四、存储抓取的数据
4.1 存储到文本文件
将抓取的数据存储到文本文件,可以使用Python内置的文件操作函数。基本代码如下:
data = 'Some data to save'
with open('data.txt', 'w') as file:
file.write(data)
4.2 存储到CSV文件
将抓取的数据存储到CSV文件,可以使用csv库。基本代码如下:
import csv
data = [['Name', 'Age'], ['Alice', 30], ['Bob', 25]]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
4.3 存储到数据库
将抓取的数据存储到数据库,可以使用SQLite数据库。基本代码如下:
import sqlite3
连接数据库
conn = sqlite3.connect('data.db')
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(name TEXT, age INTEGER)''')
插入数据
data = [('Alice', 30), ('Bob', 25)]
cursor.executemany('INSERT INTO users VALUES (?, ?)', data)
提交事务
conn.commit()
关闭连接
conn.close()
五、处理反爬虫机制
5.1 使用User-Agent
很多网站会通过检查请求头中的User-Agent来识别爬虫,可以通过设置User-Agent来绕过这种检查。基本代码如下:
import requests
url = 'http://www.example.com'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
print(response.text)
5.2 使用代理
使用代理可以隐藏爬虫的真实IP地址,防止被封禁。基本代码如下:
import requests
url = 'http://www.example.com'
proxies = {'http': 'http://10.10.1.10:3128', 'https': 'http://10.10.1.10:1080'}
response = requests.get(url, proxies=proxies)
print(response.text)
5.3 设置请求间隔
设置请求间隔可以避免频繁请求导致被封禁。基本代码如下:
import requests
import time
url = 'http://www.example.com'
for i in range(10):
response = requests.get(url)
print(response.text)
time.sleep(1) # 设置请求间隔为1秒
六、处理动态网页
6.1 使用Selenium库
对于动态网页,可以使用Selenium库来模拟浏览器操作,获取网页内容。安装Selenium库可以使用以下命令:
pip install selenium
使用Selenium库获取网页内容的基本代码如下:
from selenium import webdriver
url = 'http://www.example.com'
driver = webdriver.Chrome()
driver.get(url)
print(driver.page_source)
driver.quit()
6.2 处理JavaScript渲染内容
对于需要JavaScript渲染的内容,可以使用Selenium库来等待页面加载完成,然后再获取网页内容。基本代码如下:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
url = 'http://www.example.com'
driver = webdriver.Chrome()
driver.get(url)
等待页面加载完成
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'myElement'))
)
print(driver.page_source)
driver.quit()
七、处理验证码
7.1 手动输入验证码
对于需要手动输入验证码的网站,可以使用Selenium库来打开浏览器,手动输入验证码,然后继续爬取数据。基本代码如下:
from selenium import webdriver
url = 'http://www.example.com'
driver = webdriver.Chrome()
driver.get(url)
手动输入验证码
input('Please enter the captcha and press Enter')
print(driver.page_source)
driver.quit()
7.2 使用第三方验证码识别服务
对于需要自动识别验证码的网站,可以使用第三方验证码识别服务,如打码兔、超级鹰等。基本代码如下:
import requests
获取验证码图片
url = 'http://www.example.com/captcha'
response = requests.get(url)
with open('captcha.jpg', 'wb') as file:
file.write(response.content)
识别验证码
api_url = 'https://api.example.com/captcha'
files = {'file': open('captcha.jpg', 'rb')}
response = requests.post(api_url, files=files)
captcha = response.json()['captcha']
print('Captcha:', captcha)
通过以上步骤,可以使用Python爬取金考典的题,获取所需的数据。在实际操作中,还需要根据具体网站的结构和反爬虫机制,灵活调整代码和策略。
相关问答FAQs:
如何使用Python进行网页爬虫来获取金考典的题目?
要使用Python爬取金考典的题目,您需要掌握基本的网络请求和数据解析技术。可以使用requests
库发送HTTP请求获取网页内容,再利用BeautifulSoup
或lxml
等库来解析HTML结构,从中提取出题目信息。确保遵循网站的爬虫规则,避免过于频繁地请求服务器。
在爬取金考典题目时,是否需要处理登录验证?
很多在线学习平台包括金考典可能会要求用户登录才能访问题目。您需要使用requests
库处理登录请求,包括发送用户名和密码,获取会话cookie。成功登录后,您可以使用相同的会话对象请求题目页面,确保能够获取到需要的数据。
如何避免在爬取金考典时被网站封禁?
为了避免被网站封禁,建议您采取一些策略,例如设置请求间隔,使用代理IP、伪装请求头(如User-Agent)等,模拟正常用户的行为。此外,定期检查网站的爬虫政策,确保您的爬虫活动不违反其使用条款,以保护您的IP地址不被封禁。
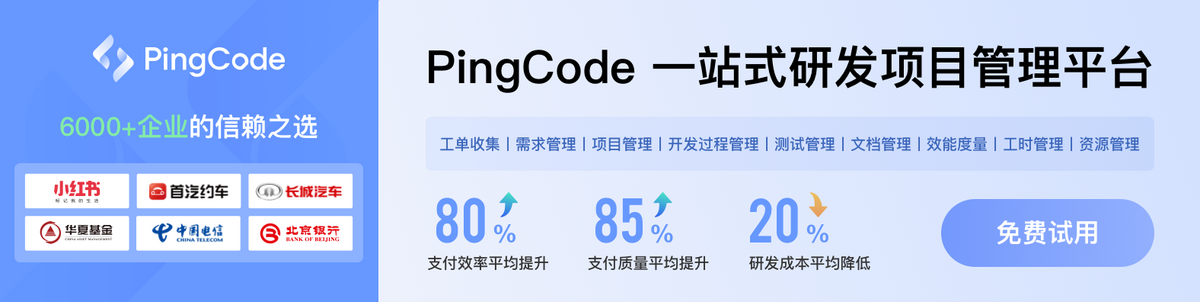