要爬取天猫旗舰店的数据,主要步骤包括使用Web爬虫工具、解析网页、模拟用户行为等。可以使用Python的第三方库如Requests、BeautifulSoup、Selenium等工具实现。本文将详细介绍如何通过这些工具爬取天猫旗舰店数据,并在实际应用中需要注意的事项。
一、了解目标网页
要爬取天猫旗舰店的数据,首先需要了解目标网页的结构和数据位置。可以使用浏览器的开发者工具(F12)查看网页的HTML代码结构,找到需要爬取的数据所在的标签和属性。
二、使用Requests库获取网页内容
Requests库是Python中常用的HTTP请求库,可以轻松地发送HTTP请求并获取网页内容。
import requests
url = 'https://www.tmall.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
print(response.text)
在上述代码中,通过指定请求头中的User-Agent
来模拟浏览器行为,防止被网站识别为爬虫工具。
三、使用BeautifulSoup解析网页内容
BeautifulSoup是一个非常强大的HTML和XML解析库,可以轻松地从网页中提取所需的数据。
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
假设我们要获取商品标题
titles = soup.find_all('div', class_='product-title')
for title in titles:
print(title.get_text(strip=True))
通过BeautifulSoup可以方便地找到HTML中的特定标签,并提取其中的文本内容。
四、处理动态加载内容
有些网页内容是通过JavaScript动态加载的,使用Requests库无法直接获取。这种情况下,可以使用Selenium模拟浏览器操作。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
import time
配置Chrome浏览器
chrome_options = Options()
chrome_options.add_argument('--headless') # 无头模式,不打开浏览器窗口
chrome_options.add_argument('--disable-gpu')
chrome_options.add_argument('--no-sandbox')
service = Service('/path/to/chromedriver') # chromedriver的路径
启动浏览器
driver = webdriver.Chrome(service=service, options=chrome_options)
driver.get(url)
等待页面加载完成
time.sleep(5)
获取动态加载的内容
titles = driver.find_elements(By.CLASS_NAME, 'product-title')
for title in titles:
print(title.text)
关闭浏览器
driver.quit()
使用Selenium可以模拟用户操作,如点击、滚动等,从而获取动态加载的网页内容。
五、处理反爬虫机制
天猫等电商平台通常有反爬虫机制,如IP封禁、验证码等。以下是一些常用的反爬虫对策:
- 使用代理IP:通过代理IP池切换IP地址,避免单个IP频繁访问被封禁。
- 模拟人类行为:随机延时、模拟鼠标移动和点击等操作。
- 设置请求头:添加常见的请求头,如
User-Agent
、Referer
等,模拟正常的浏览器请求。 - 使用分布式爬虫:使用Scrapy等框架结合分布式架构,提升爬取效率,同时降低被封禁风险。
六、数据存储
获取数据后,需要将其存储到合适的存储介质中,如数据库、CSV文件等。
import csv
假设已获取商品数据
data = [
{'title': '商品1', 'price': '100'},
{'title': '商品2', 'price': '200'},
]
保存到CSV文件
with open('products.csv', 'w', newline='', encoding='utf-8') as csvfile:
fieldnames = ['title', 'price']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in data:
writer.writerow(item)
通过以上步骤,我们可以实现从天猫旗舰店爬取商品数据并存储。需要注意的是,爬取数据应遵守网站的Robots协议和相关法律法规,合理使用爬虫工具,避免对网站造成负担或侵权。
七、实战案例:爬取天猫某旗舰店商品信息
以下是一个完整的爬取天猫某旗舰店商品信息的实战案例,结合Requests、BeautifulSoup和Selenium进行综合使用。
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
import time
import csv
def get_static_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
return response.text
def get_dynamic_content(url):
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
chrome_options.add_argument('--no-sandbox')
service = Service('/path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=chrome_options)
driver.get(url)
time.sleep(5)
titles = driver.find_elements(By.CLASS_NAME, 'product-title')
prices = driver.find_elements(By.CLASS_NAME, 'product-price')
data = []
for title, price in zip(titles, prices):
data.append({'title': title.text, 'price': price.text})
driver.quit()
return data
def save_to_csv(data, filename):
with open(filename, 'w', newline='', encoding='utf-8') as csvfile:
fieldnames = ['title', 'price']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in data:
writer.writerow(item)
def main():
url = 'https://www.tmall.com'
static_content = get_static_content(url)
soup = BeautifulSoup(static_content, 'html.parser')
# 假设我们从静态内容中提取某些信息
static_data = []
for div in soup.find_all('div', class_='some-static-class'):
static_data.append({'title': div.get_text(strip=True), 'price': 'N/A'})
dynamic_data = get_dynamic_content(url)
# 合并静态和动态数据
all_data = static_data + dynamic_data
save_to_csv(all_data, 'products.csv')
if __name__ == '__main__':
main()
以上代码展示了如何结合使用Requests、BeautifulSoup和Selenium,从天猫某旗舰店获取商品信息并保存到CSV文件中。通过这种方式,可以有效地爬取天猫上的商品数据,并应用于数据分析、市场调研等领域。
相关问答FAQs:
如何使用Python爬取天猫旗舰店的商品信息?
使用Python爬取天猫旗舰店的商品信息,通常需要使用第三方库,如Requests和BeautifulSoup。首先,通过Requests库获取网页的HTML内容,然后利用BeautifulSoup解析HTML,提取需要的商品信息,比如标题、价格和销量等。此外,注意天猫对爬虫的限制,可能需要设置合适的请求头或使用代理IP来避免被封禁。
在爬取天猫数据时,如何处理反爬虫机制?
天猫作为电商平台,具有较强的反爬虫机制。为应对这一挑战,建议随机设置User-Agent,模拟真实用户的浏览行为。此外,可以通过控制请求频率,避免短时间内发送大量请求。同时,使用代理IP来分散请求来源,增加成功爬取的几率。对于动态加载的内容,可以考虑使用Selenium等工具进行模拟浏览器操作。
爬取天猫旗舰店的商品数据是否合法?
在进行数据爬取时,法律和道德问题需高度重视。爬取天猫旗舰店的商品数据可能涉及到知识产权和数据使用政策,因此在进行爬取之前,建议仔细阅读并遵循网站的使用条款。此外,尽量避免对网站造成负担,合理控制爬取频率和数据量,尊重网站的robots.txt文件中的爬虫协议。
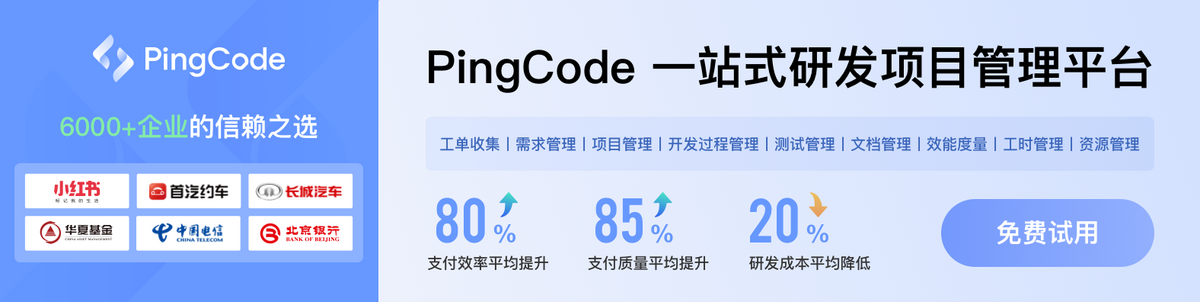