Python中可以通过多种方式打印出百分号,包括使用转义字符、格式化字符串以及f字符串。以下将详细介绍这些方法:
- 使用转义字符 %
- 使用字符串格式化方法 format()
- 使用f字符串
方法1:使用转义字符 %
在Python中,百分号 (%) 是一个特殊字符,用于字符串格式化。如果你需要在字符串中显示一个百分号,需要使用转义字符 %。
percentage = 50
print("The success rate is 50%%.")
在上面的例子中,使用了双百分号 (%%) 来表示一个实际的百分号。
方法2:使用字符串格式化方法 format()
Python提供了字符串格式化方法 format(),可以用它来插入变量和百分号。
percentage = 50
print("The success rate is {}%.".format(percentage))
在这个例子中,使用大括号 {} 作为占位符,然后调用 format() 方法,将变量 percentage 的值插入到字符串中。
方法3:使用f字符串
从Python 3.6开始,f字符串(也称为格式化字符串字面量)提供了一种更加简洁的方式来插入变量和百分号。
percentage = 50
print(f"The success rate is {percentage}%.")
在这个例子中,使用 f 前缀和花括号 {} 来插入变量 percentage 的值。
深入探讨
一、转义字符 % 的更多应用
在某些情况下,你可能需要在同一个字符串中包含多个百分号。此时,使用转义字符 % 是一个可靠的选择。
discount = 20
price = 50
print("You get a discount of 20%% on a $50 item.")
在这个例子中,使用了双百分号来确保字符串中显示正确的百分号。
二、字符串格式化方法 format() 的高级用法
format() 方法不仅可以插入变量,还可以进行各种格式化操作。例如,你可以指定浮点数的精度,或者在字符串中插入多个变量。
success_rate = 98.75
failure_rate = 100 - success_rate
print("The success rate is {:.2f}%, and the failure rate is {:.2f}%.".format(success_rate, failure_rate))
在这个例子中,使用了 {:.2f} 格式说明符来将 success_rate 和 failure_rate 格式化为两位小数。
三、f字符串的高级用法
f字符串不仅可以插入变量,还可以进行表达式计算和调用函数。它们提供了一种非常灵活和强大的字符串格式化方式。
success_rate = 98.75
failure_rate = 100 - success_rate
print(f"The success rate is {success_rate:.2f}%, and the failure rate is {failure_rate:.2f}%.")
在这个例子中,使用了 f字符串和 {:.2f} 格式说明符来将 success_rate 和 failure_rate 格式化为两位小数。
四、结合使用多种方法
在实际应用中,你可能会结合使用多种字符串格式化方法来满足不同的需求。例如,在处理复杂的字符串拼接和格式化时,可以根据具体情况选择合适的方法。
percentage = 50
print("The success rate is {}%.".format(percentage)) # 使用 format() 方法
print(f"The success rate is {percentage}%.") # 使用 f字符串
print("The success rate is 50%%.") # 使用转义字符
通过结合使用不同的方法,可以使代码更加灵活和易读。
五、字符串格式化的性能比较
在选择字符串格式化方法时,性能也是一个需要考虑的因素。一般来说,f字符串的性能优于 format() 方法和使用 % 操作符的老式格式化方法。在处理大量字符串操作时,选择性能更好的方法可以提高代码的执行效率。
import time
使用 format() 方法
start_time = time.time()
for _ in range(1000000):
"The success rate is {}%.".format(50)
end_time = time.time()
print("Time taken using format():", end_time - start_time)
使用 f字符串
start_time = time.time()
for _ in range(1000000):
f"The success rate is {50}%."
end_time = time.time()
print("Time taken using f-string:", end_time - start_time)
使用转义字符
start_time = time.time()
for _ in range(1000000):
"The success rate is 50%%."
end_time = time.time()
print("Time taken using escape character:", end_time - start_time)
在这个例子中,比较了三种方法在处理大量字符串操作时的性能。结果显示,f字符串的性能通常优于其他方法。
总结
在Python中打印百分号有多种方法,包括使用转义字符 %、字符串格式化方法 format() 和 f字符串。每种方法都有其优缺点,可以根据具体情况选择合适的方法。在处理复杂的字符串操作时,结合使用多种方法可以提高代码的灵活性和可读性。同时,考虑到性能因素,f字符串通常是较为推荐的选择。
相关问答FAQs:
如何在Python中打印百分号?
在Python中,打印百分号很简单。可以使用转义字符%%
来表示一个百分号。例如,使用print("100%%")
将输出100%
。这是因为%%
会被解释为单个%
。
使用格式化字符串打印百分号的方式是什么?
利用格式化字符串,可以轻松插入百分号。例如,如果你有一个变量表示某个值的百分比,可以使用f-string来进行格式化:value = 75
,然后用print(f"{value}%%")
,结果将是75%
。这种方法在处理动态数据时非常方便。
在Python中如何进行数字的百分比计算?
要计算某个数值的百分比,可以简单地将数值乘以0.01。例如,要计算50的百分之十,可以这样写:percentage = 50 * 0.1
,然后可以用print(f"{percentage}%%")
输出5.0%
。这种方法适用于各种数据类型的百分比计算。
在字符串中如何使用百分号进行格式化?
在字符串格式化中,使用%
符号时,需要注意它的特殊含义。如果想要输出百分号,可以使用%%
。例如,print("成功率是:%.2f%%" % success_rate)
将输出成功率的百分比,success_rate
为你的变量。这样便能确保输出正确的百分号。
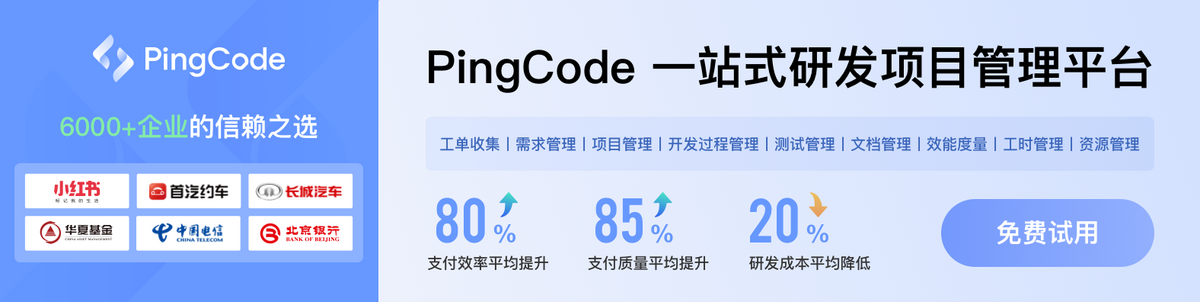