Python可以通过多种方式将txt文件打印出来,包括读取文件内容并在控制台输出、使用文件对象的read()方法、使用with语句管理文件资源等。以下将详细介绍这些方法及其使用场景。 在实际使用中,可以根据具体需求选择适合的方法,下面将详细介绍其中一种方法:使用with语句和文件对象的read()方法来读取txt文件内容并打印出来。
使用with语句管理文件资源:
使用with语句来管理文件资源是Python中一种非常推荐的做法,因为它能够确保文件在使用完毕后自动关闭,即使在出现异常的情况下。这样不仅能够提高代码的健壮性,还能防止文件句柄泄漏。
以下是一个示例代码,展示了如何使用with语句和文件对象的read()方法来读取txt文件内容并打印出来:
def print_txt_file(file_path):
# 使用with语句打开文件
with open(file_path, 'r', encoding='utf-8') as file:
# 读取文件内容
file_content = file.read()
# 打印文件内容
print(file_content)
调用函数并传入txt文件的路径
print_txt_file('example.txt')
在这个示例中,open()
函数用于打开txt文件,'r'
参数表示以只读模式打开文件,encoding='utf-8'
用于指定文件编码格式。with
语句确保文件在使用完毕后自动关闭,file.read()
方法读取文件的全部内容并存储在变量file_content
中,最后通过print()
函数将文件内容打印到控制台。
一、读取txt文件内容并打印
读取txt文件内容并打印的方法有多种,下面将逐一介绍。
1、使用read()方法读取文件内容
read()
方法用于读取文件的全部内容。使用这个方法可以一次性将文件内容加载到内存中,适用于文件较小的情况。
def print_file_using_read(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
示例调用
print_file_using_read('example.txt')
2、使用readlines()方法逐行读取文件内容
readlines()
方法用于将文件的每一行作为一个字符串元素存储在列表中。适用于需要逐行处理文件内容的情况。
def print_file_using_readlines(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
示例调用
print_file_using_readlines('example.txt')
3、使用for循环逐行读取文件内容
直接使用for循环遍历文件对象,可以逐行读取文件内容。这种方法在处理大文件时更加高效,因为它不会一次性将文件内容加载到内存中。
def print_file_using_for_loop(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
print(line, end='')
示例调用
print_file_using_for_loop('example.txt')
二、处理大文件的注意事项
在处理大文件时,需要特别注意内存的使用情况。一次性将整个文件内容加载到内存中可能会导致内存不足的问题。下面介绍几种处理大文件的方法。
1、逐行读取文件内容
逐行读取文件内容是处理大文件时常用的方法。可以通过for循环遍历文件对象来实现逐行读取。
def print_large_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
print(line, end='')
示例调用
print_large_file('large_file.txt')
2、使用生成器读取文件内容
生成器是一种惰性计算的迭代器,可以逐步生成数据而不是一次性将数据加载到内存中。使用生成器可以有效减少内存占用。
def read_file_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r', encoding='utf-8') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
yield chunk
def print_large_file_with_generator(file_path):
for chunk in read_file_in_chunks(file_path):
print(chunk, end='')
示例调用
print_large_file_with_generator('large_file.txt')
三、处理不同编码格式的txt文件
在处理txt文件时,需要注意文件的编码格式。常见的编码格式有UTF-8、UTF-16、GBK等。如果文件的编码格式不正确,可能会导致读取文件时出现乱码或异常。可以通过open()
函数的encoding
参数指定文件的编码格式。
1、读取UTF-8编码格式的文件
def print_utf8_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
示例调用
print_utf8_file('utf8_file.txt')
2、读取GBK编码格式的文件
def print_gbk_file(file_path):
with open(file_path, 'r', encoding='gbk') as file:
content = file.read()
print(content)
示例调用
print_gbk_file('gbk_file.txt')
四、写入txt文件
除了读取txt文件内容,Python也可以将内容写入txt文件。下面介绍几种写入txt文件的方法。
1、使用write()方法写入文件
write()
方法用于将字符串内容写入文件。如果文件不存在,会自动创建一个新文件。如果文件存在,默认会覆盖原有内容。
def write_to_file(file_path, content):
with open(file_path, 'w', encoding='utf-8') as file:
file.write(content)
示例调用
write_to_file('output.txt', 'Hello, world!')
2、使用writelines()方法写入文件
writelines()
方法用于将字符串列表写入文件。每个字符串元素将作为文件的一行。
def write_lines_to_file(file_path, lines):
with open(file_path, 'w', encoding='utf-8') as file:
file.writelines(lines)
示例调用
write_lines_to_file('output.txt', ['Hello, world!\n', 'Python is great!\n'])
3、追加写入文件
使用'a'
模式打开文件,可以在文件末尾追加内容,而不会覆盖原有内容。
def append_to_file(file_path, content):
with open(file_path, 'a', encoding='utf-8') as file:
file.write(content)
示例调用
append_to_file('output.txt', 'Appending new content.\n')
五、处理txt文件的常见问题
在处理txt文件时,可能会遇到一些常见问题,如文件不存在、权限不足、编码错误等。下面介绍几种常见问题的处理方法。
1、处理文件不存在的情况
在打开文件之前,可以使用os.path.exists()
函数检查文件是否存在。如果文件不存在,可以提前处理,避免程序异常。
import os
def read_file_if_exists(file_path):
if os.path.exists(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
else:
print(f"File '{file_path}' does not exist.")
示例调用
read_file_if_exists('example.txt')
2、处理权限不足的情况
在读取或写入文件时,如果文件权限不足,可能会引发权限异常。可以使用try-except语句捕获异常并进行处理。
def safe_read_file(file_path):
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
except PermissionError:
print(f"Permission denied: '{file_path}'")
示例调用
safe_read_file('example.txt')
3、处理编码错误的情况
在读取文件时,如果编码格式不正确,可能会引发编码错误。可以使用try-except语句捕获异常并进行处理。
def safe_read_file_with_encoding(file_path, encoding='utf-8'):
try:
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
print(content)
except UnicodeDecodeError:
print(f"Encoding error: '{file_path}'")
示例调用
safe_read_file_with_encoding('example.txt', 'utf-8')
六、总结
本文介绍了Python如何将txt文件打印出来的多种方法,包括使用read()方法、readlines()方法、for循环逐行读取、生成器逐块读取等。还介绍了处理大文件和不同编码格式文件的方法,以及写入txt文件的多种方式。最后,介绍了处理文件不存在、权限不足、编码错误等常见问题的方法。在实际应用中,可以根据具体需求选择适合的方法来处理txt文件。
通过掌握这些方法和技巧,可以更高效地处理和操作txt文件,提高Python编程的实用性和灵活性。希望本文对你在处理txt文件时有所帮助。
相关问答FAQs:
如何在Python中读取和打印txt文件的内容?
在Python中,读取和打印txt文件的内容非常简单。您可以使用内置的open()
函数打开文件,并结合read()
或readlines()
方法来读取内容。以下是一个基本示例:
with open('yourfile.txt', 'r') as file:
content = file.read()
print(content)
这种方法会将整个文件的内容读取到一个字符串中,并打印出来。
Python中如何逐行读取和打印txt文件?
如果您想逐行读取txt文件,可以使用readline()
或for
循环来遍历文件对象。这样可以节省内存,特别是对于大型文件。示例如下:
with open('yourfile.txt', 'r') as file:
for line in file:
print(line.strip()) # strip()用于去除行末的换行符
这个方法会逐行打印文件内容,使得输出更加整洁。
在Python中如何处理文件不存在或读取错误的情况?
在处理文件时,可能会遇到文件不存在或其他读取错误的情况。使用try-except
语句可以有效地捕捉这些异常。示例如下:
try:
with open('yourfile.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except Exception as e:
print(f"读取文件时发生错误:{e}")
这种方式可以帮助您在文件读取过程中处理潜在的问题,确保程序的健壮性。
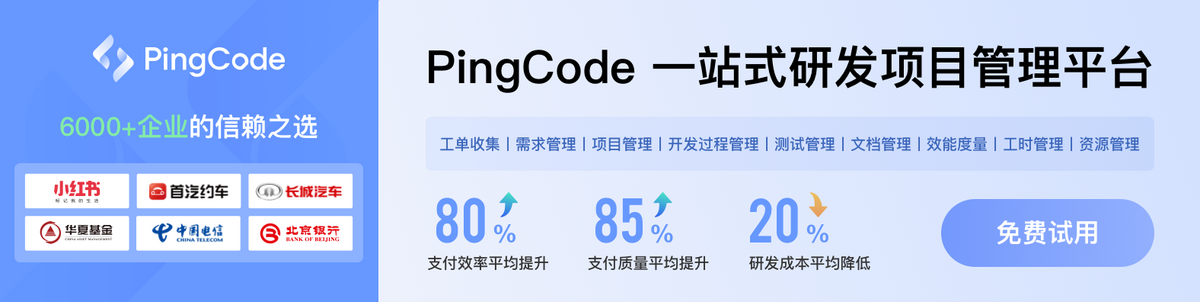