使用Python从网上获取数据库的方法包括使用Web scraping工具、REST API、数据库连接库等。我们将详细介绍如何使用Python的requests和BeautifulSoup库进行Web scraping、使用REST API获取数据库信息、以及通过数据库连接库(如SQLAlchemy)连接并获取数据。
Web scraping工具和REST API是获取数据库信息的常用方法。
一、使用Web scraping工具
Web scraping是指通过编程自动从网页提取信息的过程。Python有许多强大的库可以用于Web scraping,包括requests和BeautifulSoup。
1、安装和导入库
首先,我们需要安装并导入requests和BeautifulSoup库:
pip install requests
pip install beautifulsoup4
import requests
from bs4 import BeautifulSoup
2、发送HTTP请求
使用requests库发送HTTP请求以获取网页内容:
url = 'http://example.com'
response = requests.get(url)
3、解析HTML内容
使用BeautifulSoup解析网页内容:
soup = BeautifulSoup(response.content, 'html.parser')
4、提取数据
我们可以使用BeautifulSoup提供的各种方法来提取所需的数据。例如,提取所有标题标签:
titles = soup.find_all('h1')
for title in titles:
print(title.get_text())
二、使用REST API获取数据库信息
许多网站和服务提供REST API以供程序访问。使用Python的requests库可以很方便地与这些API进行交互。
1、发送请求
我们使用requests库发送HTTP请求:
url = 'http://api.example.com/data'
response = requests.get(url)
data = response.json()
2、处理响应数据
一旦我们获取到响应数据,我们就可以处理并提取我们需要的信息:
for item in data['items']:
print(item['name'], item['value'])
三、使用数据库连接库
Python有许多库可以用于连接和操作数据库,包括SQLAlchemy、pymysql、psycopg2等。
1、安装和导入库
首先,我们需要安装并导入相应的数据库连接库。例如,使用SQLAlchemy:
pip install sqlalchemy
from sqlalchemy import create_engine
2、创建数据库连接
创建一个数据库连接引擎:
engine = create_engine('mysql+pymysql://user:password@host:port/dbname')
3、执行SQL查询
使用连接引擎执行SQL查询并获取数据:
with engine.connect() as connection:
result = connection.execute("SELECT * FROM table_name")
for row in result:
print(row)
四、综合实例
让我们通过一个综合实例来展示如何使用这些方法获取数据库信息。
1、从网页获取数据
假设我们需要从一个包含产品信息的网页获取数据,并将其存储到数据库中。
import requests
from bs4 import BeautifulSoup
from sqlalchemy import create_engine
发送HTTP请求
url = 'http://example.com/products'
response = requests.get(url)
解析HTML内容
soup = BeautifulSoup(response.content, 'html.parser')
提取产品信息
products = []
for product in soup.find_all('div', class_='product'):
name = product.find('h2').get_text()
price = product.find('span', class_='price').get_text()
products.append({'name': name, 'price': price})
创建数据库连接
engine = create_engine('mysql+pymysql://user:password@host:port/dbname')
存储数据到数据库
with engine.connect() as connection:
for product in products:
connection.execute("INSERT INTO products (name, price) VALUES (%s, %s)", (product['name'], product['price']))
2、从API获取数据
假设我们需要从一个API获取用户信息,并将其存储到数据库中。
import requests
from sqlalchemy import create_engine
发送HTTP请求
url = 'http://api.example.com/users'
response = requests.get(url)
data = response.json()
创建数据库连接
engine = create_engine('mysql+pymysql://user:password@host:port/dbname')
存储数据到数据库
with engine.connect() as connection:
for user in data['users']:
connection.execute("INSERT INTO users (id, name, email) VALUES (%s, %s, %s)", (user['id'], user['name'], user['email']))
五、处理复杂数据
在实际应用中,数据可能会更加复杂,我们需要处理嵌套数据、分页数据等。
1、处理嵌套数据
假设我们需要处理一个包含嵌套数据的API响应:
import requests
from sqlalchemy import create_engine
发送HTTP请求
url = 'http://api.example.com/orders'
response = requests.get(url)
data = response.json()
创建数据库连接
engine = create_engine('mysql+pymysql://user:password@host:port/dbname')
存储数据到数据库
with engine.connect() as connection:
for order in data['orders']:
connection.execute("INSERT INTO orders (id, date, total) VALUES (%s, %s, %s)", (order['id'], order['date'], order['total']))
for item in order['items']:
connection.execute("INSERT INTO order_items (order_id, product, quantity) VALUES (%s, %s, %s)", (order['id'], item['product'], item['quantity']))
2、处理分页数据
假设我们需要处理一个包含分页数据的API响应:
import requests
from sqlalchemy import create_engine
创建数据库连接
engine = create_engine('mysql+pymysql://user:password@host:port/dbname')
处理分页数据
page = 1
while True:
url = f'http://api.example.com/users?page={page}'
response = requests.get(url)
data = response.json()
if not data['users']:
break
with engine.connect() as connection:
for user in data['users']:
connection.execute("INSERT INTO users (id, name, email) VALUES (%s, %s, %s)", (user['id'], user['name'], user['email']))
page += 1
六、总结
在这篇文章中,我们详细介绍了如何使用Python从网上获取数据库信息的方法,包括使用Web scraping工具、REST API、数据库连接库等。Web scraping工具和REST API是获取数据库信息的常用方法,而数据库连接库则可以用于直接连接和操作数据库。通过实际示例,我们展示了如何使用这些方法从网页和API获取数据,并将其存储到数据库中。希望这些方法和示例能帮助您更好地理解和应用Python进行数据获取和处理。
相关问答FAQs:
如何使用Python连接到网络数据库?
使用Python连接到网络数据库通常需要使用适当的库,例如SQLAlchemy
、psycopg2
(对于PostgreSQL)或pymysql
(对于MySQL)。首先,您需要安装所需的库。然后,使用数据库的连接字符串进行连接,确保提供正确的用户名、密码、主机地址和数据库名称。连接后,您可以执行SQL查询,获取所需的数据。
在Python中如何处理获取的数据库数据?
获取数据后,您可以使用pandas
库将数据加载到DataFrame中,这使得数据处理和分析变得更加简单。使用pandas.read_sql_query()
函数可以将SQL查询的结果直接转换为DataFrame。处理后,您可以利用pandas
提供的多种功能进行数据清洗、分析和可视化。
在获取数据库数据时如何处理异常和错误?
在进行数据库操作时,捕获异常是确保程序稳定运行的关键。可以使用try-except
块来捕获连接错误、查询错误等。确保在捕获异常时提供清晰的错误信息,并在必要时进行资源释放(例如关闭数据库连接),以避免潜在的内存泄漏或连接超时问题。
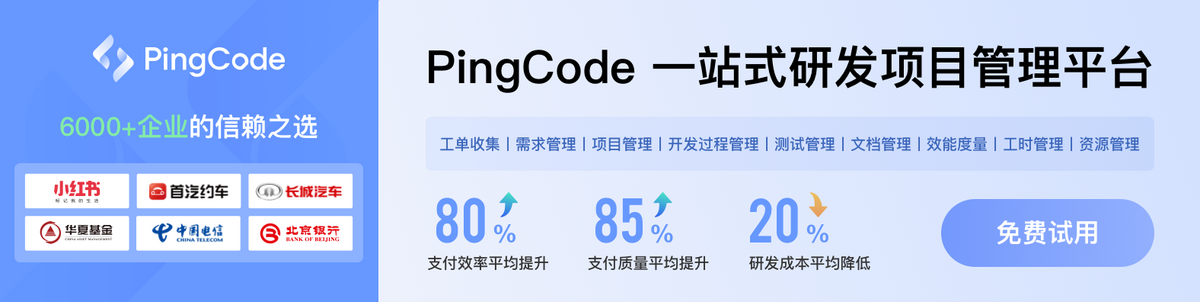