要获取图像的经纬度坐标,可以使用Python中的Pillow库和ExifRead库来读取图像的EXIF数据。主要方法包括:使用ExifRead库读取EXIF数据、解析GPS信息、转换GPS坐标格式。 其中,使用ExifRead库读取EXIF数据是最为核心的步骤。
一、安装必要的Python库
在开始处理图像之前,需要先安装一些必要的Python库。具体包括Pillow库和ExifRead库。可以使用pip命令进行安装:
pip install Pillow
pip install exifread
二、读取图像文件的EXIF数据
EXIF数据是存储在JPEG和TIFF图像文件中的元数据,它包含了图像的各种信息,包括拍摄时间、相机设置和GPS坐标。使用ExifRead库可以方便地读取这些数据。
以下是一个示例代码,展示了如何读取图像文件的EXIF数据:
import exifread
def read_exif_data(image_path):
with open(image_path, 'rb') as image_file:
tags = exifread.process_file(image_file)
return tags
image_path = 'your_image.jpg'
exif_data = read_exif_data(image_path)
for tag in exif_data.keys():
print(f"{tag}: {exif_data[tag]}")
上述代码会打印出图像文件的所有EXIF标签和对应的值。
三、解析GPS信息
EXIF数据中的GPS信息通常存储在特定的标签中,需要对其进行解析才能获得经纬度坐标。以下是解析GPS信息的示例代码:
def get_gps_info(exif_data):
gps_info = {}
gps_tags = ['GPS GPSLatitude', 'GPS GPSLatitudeRef', 'GPS GPSLongitude', 'GPS GPSLongitudeRef']
for tag in gps_tags:
if tag in exif_data:
gps_info[tag] = exif_data[tag]
return gps_info
gps_info = get_gps_info(exif_data)
for tag, value in gps_info.items():
print(f"{tag}: {value}")
四、转换GPS坐标格式
GPS坐标通常以度、分、秒的格式存储,需要将其转换为十进制格式,以便于进一步使用。以下是一个将度、分、秒格式的GPS坐标转换为十进制格式的函数:
def convert_to_decimal_degrees(value, ref):
degrees = value.values[0]
minutes = value.values[1]
seconds = value.values[2]
decimal_degrees = degrees + (minutes / 60.0) + (seconds / 3600.0)
if ref == 'S' or ref == 'W':
decimal_degrees = -decimal_degrees
return decimal_degrees
latitude = convert_to_decimal_degrees(gps_info['GPS GPSLatitude'], gps_info['GPS GPSLatitudeRef'])
longitude = convert_to_decimal_degrees(gps_info['GPS GPSLongitude'], gps_info['GPS GPSLongitudeRef'])
print(f"Latitude: {latitude}, Longitude: {longitude}")
五、综合示例
为了更清晰地展示整个过程,下面是一个完整的示例代码,展示了如何读取图像文件的EXIF数据、解析GPS信息并转换为十进制格式的经纬度坐标:
import exifread
def read_exif_data(image_path):
with open(image_path, 'rb') as image_file:
tags = exifread.process_file(image_file)
return tags
def get_gps_info(exif_data):
gps_info = {}
gps_tags = ['GPS GPSLatitude', 'GPS GPSLatitudeRef', 'GPS GPSLongitude', 'GPS GPSLongitudeRef']
for tag in gps_tags:
if tag in exif_data:
gps_info[tag] = exif_data[tag]
return gps_info
def convert_to_decimal_degrees(value, ref):
degrees = value.values[0]
minutes = value.values[1]
seconds = value.values[2]
decimal_degrees = degrees + (minutes / 60.0) + (seconds / 3600.0)
if ref == 'S' or ref == 'W':
decimal_degrees = -decimal_degrees
return decimal_degrees
image_path = 'your_image.jpg'
exif_data = read_exif_data(image_path)
gps_info = get_gps_info(exif_data)
if gps_info:
latitude = convert_to_decimal_degrees(gps_info['GPS GPSLatitude'], gps_info['GPS GPSLatitudeRef'])
longitude = convert_to_decimal_degrees(gps_info['GPS GPSLongitude'], gps_info['GPS GPSLongitudeRef'])
print(f"Latitude: {latitude}, Longitude: {longitude}")
else:
print("No GPS information found.")
六、处理其他图像格式
虽然JPEG和TIFF是最常见的包含EXIF数据的图像格式,但有些其他格式也可能包含类似的元数据。对于这些格式,可以使用Pillow库来读取图像并提取其元数据。
以下是一个示例代码,展示了如何使用Pillow库读取图像并提取其元数据:
from PIL import Image
from PIL.ExifTags import TAGS
def get_exif_data(image_path):
image = Image.open(image_path)
exif_data = image._getexif()
if exif_data is not None:
exif = {
TAGS.get(tag, tag): value
for tag, value in exif_data.items()
}
return exif
return {}
image_path = 'your_image.jpg'
exif_data = get_exif_data(image_path)
for tag, value in exif_data.items():
print(f"{tag}: {value}")
七、处理缺失的EXIF数据
在某些情况下,图像文件可能缺少EXIF数据,特别是GPS信息。这种情况下,需要处理这些缺失数据,并给予相应的提示。
以下是一个处理缺失EXIF数据的示例代码:
import exifread
def read_exif_data(image_path):
try:
with open(image_path, 'rb') as image_file:
tags = exifread.process_file(image_file)
return tags
except Exception as e:
print(f"Error reading EXIF data: {e}")
return {}
def get_gps_info(exif_data):
gps_info = {}
gps_tags = ['GPS GPSLatitude', 'GPS GPSLatitudeRef', 'GPS GPSLongitude', 'GPS GPSLongitudeRef']
for tag in gps_tags:
if tag in exif_data:
gps_info[tag] = exif_data[tag]
return gps_info
def convert_to_decimal_degrees(value, ref):
degrees = value.values[0]
minutes = value.values[1]
seconds = value.values[2]
decimal_degrees = degrees + (minutes / 60.0) + (seconds / 3600.0)
if ref == 'S' or ref == 'W':
decimal_degrees = -decimal_degrees
return decimal_degrees
image_path = 'your_image.jpg'
exif_data = read_exif_data(image_path)
gps_info = get_gps_info(exif_data)
if gps_info:
latitude = convert_to_decimal_degrees(gps_info['GPS GPSLatitude'], gps_info['GPS GPSLatitudeRef'])
longitude = convert_to_decimal_degrees(gps_info['GPS GPSLongitude'], gps_info['GPS GPSLongitudeRef'])
print(f"Latitude: {latitude}, Longitude: {longitude}")
else:
print("No GPS information found.")
八、处理大批量图像
如果需要处理大量图像文件,可以将上述代码封装到一个函数中,并遍历图像文件列表来处理每一个图像。以下是一个批量处理图像文件的示例代码:
import os
import exifread
def read_exif_data(image_path):
try:
with open(image_path, 'rb') as image_file:
tags = exifread.process_file(image_file)
return tags
except Exception as e:
print(f"Error reading EXIF data: {e}")
return {}
def get_gps_info(exif_data):
gps_info = {}
gps_tags = ['GPS GPSLatitude', 'GPS GPSLatitudeRef', 'GPS GPSLongitude', 'GPS GPSLongitudeRef']
for tag in gps_tags:
if tag in exif_data:
gps_info[tag] = exif_data[tag]
return gps_info
def convert_to_decimal_degrees(value, ref):
degrees = value.values[0]
minutes = value.values[1]
seconds = value.values[2]
decimal_degrees = degrees + (minutes / 60.0) + (seconds / 3600.0)
if ref == 'S' or ref == 'W':
decimal_degrees = -decimal_degrees
return decimal_degrees
def process_images(image_folder):
for root, dirs, files in os.walk(image_folder):
for file in files:
if file.lower().endswith(('.jpg', '.jpeg', '.tiff')):
image_path = os.path.join(root, file)
exif_data = read_exif_data(image_path)
gps_info = get_gps_info(exif_data)
if gps_info:
latitude = convert_to_decimal_degrees(gps_info['GPS GPSLatitude'], gps_info['GPS GPSLatitudeRef'])
longitude = convert_to_decimal_degrees(gps_info['GPS GPSLongitude'], gps_info['GPS GPSLongitudeRef'])
print(f"Image: {file}, Latitude: {latitude}, Longitude: {longitude}")
else:
print(f"Image: {file}, No GPS information found.")
image_folder = 'path_to_your_image_folder'
process_images(image_folder)
九、总结
通过以上步骤,可以使用Python读取图像文件的EXIF数据,并提取其中的GPS信息来获取图像的经纬度坐标。关键步骤包括:安装必要的库、读取EXIF数据、解析GPS信息、转换坐标格式。在处理大批量图像文件时,可以使用批处理方法来提高效率。希望这些示例代码能够帮助你更好地理解和实现图像经纬度坐标的获取。
相关问答FAQs:
如何在Python中读取图像的EXIF数据?
在Python中,可以使用PIL库(Pillow)和exifread库来读取图像的EXIF数据。EXIF数据通常包含拍摄时的经纬度信息。首先,你需要安装这些库,可以通过pip命令来实现。读取图像后,通过访问EXIF字典中的经纬度标签,可以获取到相应的坐标信息。
获取经纬度坐标时需要注意哪些图像格式?
并非所有图像文件都包含EXIF数据,常见的格式如JPEG和TIFF通常会包含这些信息,而PNG和GIF则不支持EXIF。因此,在处理图像之前,请确保你的图像格式支持EXIF数据,以便能够成功提取经纬度坐标。
如果图像没有经纬度信息,应该如何处理?
在某些情况下,图像可能不包含经纬度信息。在这种情况下,可以考虑使用其他方法来获取位置数据。例如,使用图像的文件名、路径信息或结合其他数据源(如地图API)来推断图像的地理位置。确保在处理这些图像时,记录下缺失位置的情况,以便于后续的数据整理和分析。
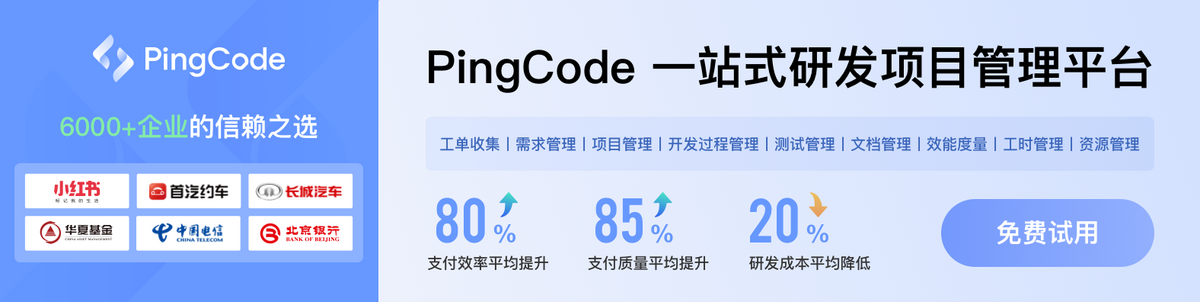