要复制一个文件夹(包括其所有内容)在Python中,通常使用shutil
模块。使用shutil.copytree()函数、处理文件夹不存在的情况、保持文件权限等是实现这一目标的关键点。下面是详细介绍这些方面的内容。
一、使用shutil.copytree()函数
shutil
模块是Python标准库的一部分,提供了许多用于文件操作的实用函数。要复制一个文件夹及其内容,可以使用shutil.copytree()
函数。这个函数接受源文件夹路径和目标文件夹路径作为参数,并递归复制所有内容。
import shutil
def copy_folder(src, dst):
try:
shutil.copytree(src, dst)
print(f"Folder {src} copied to {dst} successfully.")
except Exception as e:
print(f"Error: {e}")
示例用法
copy_folder('path/to/source_folder', 'path/to/destination_folder')
二、处理文件夹不存在的情况
当源文件夹或目标文件夹不存在时,代码会报错。为了使代码更加健壮,我们可以在复制前检查这些文件夹是否存在。
import os
import shutil
def copy_folder(src, dst):
if not os.path.exists(src):
print(f"Source folder {src} does not exist.")
return
if os.path.exists(dst):
print(f"Destination folder {dst} already exists.")
return
try:
shutil.copytree(src, dst)
print(f"Folder {src} copied to {dst} successfully.")
except Exception as e:
print(f"Error: {e}")
示例用法
copy_folder('path/to/source_folder', 'path/to/destination_folder')
三、保持文件权限
在某些情况下,您可能需要保持源文件夹及其内容的文件权限。这可以通过传递copy_function
参数给shutil.copytree()
函数来实现。默认情况下,shutil.copy2
会保留文件的元数据(如权限)。
import os
import shutil
def copy_folder(src, dst):
if not os.path.exists(src):
print(f"Source folder {src} does not exist.")
return
if os.path.exists(dst):
print(f"Destination folder {dst} already exists.")
return
try:
shutil.copytree(src, dst, copy_function=shutil.copy2)
print(f"Folder {src} copied to {dst} successfully.")
except Exception as e:
print(f"Error: {e}")
示例用法
copy_folder('path/to/source_folder', 'path/to/destination_folder')
四、忽略特定文件或文件夹
有时,您可能不想复制源文件夹中的所有文件。例如,您可能希望忽略某些临时文件或不需要的子文件夹。可以使用ignore
参数来实现这一点。
import os
import shutil
def ignore_files(dir, files):
return [f for f in files if os.path.splitext(f)[1] in ['.tmp', '.log']]
def copy_folder(src, dst):
if not os.path.exists(src):
print(f"Source folder {src} does not exist.")
return
if os.path.exists(dst):
print(f"Destination folder {dst} already exists.")
return
try:
shutil.copytree(src, dst, ignore=ignore_files)
print(f"Folder {src} copied to {dst} successfully.")
except Exception as e:
print(f"Error: {e}")
示例用法
copy_folder('path/to/source_folder', 'path/to/destination_folder')
五、处理大文件夹
对于包含大量文件或非常大的文件夹,复制操作可能需要很长时间。在这种情况下,您可能希望提供进度反馈或使用多线程来加快复制过程。以下是一个简单的示例,显示如何使用tqdm
库来显示进度条。
import os
import shutil
from tqdm import tqdm
def copy_with_progress(src, dst):
total_size = sum(os.path.getsize(os.path.join(root, file)) for root, _, files in os.walk(src) for file in files)
copied_size = 0
def copy_function(src, dst, *, follow_symlinks=True):
nonlocal copied_size
shutil.copy2(src, dst, follow_symlinks=follow_symlinks)
copied_size += os.path.getsize(src)
progress_bar.update(copied_size - progress_bar.n)
with tqdm(total=total_size, unit='B', unit_scale=True) as progress_bar:
shutil.copytree(src, dst, copy_function=copy_function)
示例用法
copy_with_progress('path/to/source_folder', 'path/to/destination_folder')
六、总结
通过使用shutil.copytree()
函数,我们可以方便地复制文件夹及其所有内容。为了处理各种情况,我们可以检查文件夹是否存在、保留文件权限、忽略特定文件以及处理大文件夹的复制过程。以下是完整示例:
import os
import shutil
from tqdm import tqdm
def ignore_files(dir, files):
return [f for f in files if os.path.splitext(f)[1] in ['.tmp', '.log']]
def copy_with_progress(src, dst):
if not os.path.exists(src):
print(f"Source folder {src} does not exist.")
return
if os.path.exists(dst):
print(f"Destination folder {dst} already exists.")
return
total_size = sum(os.path.getsize(os.path.join(root, file)) for root, _, files in os.walk(src) for file in files)
copied_size = 0
def copy_function(src, dst, *, follow_symlinks=True):
nonlocal copied_size
shutil.copy2(src, dst, follow_symlinks=follow_symlinks)
copied_size += os.path.getsize(src)
progress_bar.update(copied_size - progress_bar.n)
with tqdm(total=total_size, unit='B', unit_scale=True) as progress_bar:
shutil.copytree(src, dst, copy_function=copy_function, ignore=ignore_files)
示例用法
copy_with_progress('path/to/source_folder', 'path/to/destination_folder')
通过这种方法,您可以有效地复制文件夹及其内容,并处理各种特殊情况。
相关问答FAQs:
如何使用Python复制文件夹?
在Python中,可以使用shutil
模块中的copytree
函数来复制整个文件夹。这个函数不仅可以复制文件夹的结构,还能复制其中的所有文件和子文件夹。以下是一个简单的示例:
import shutil
shutil.copytree('源文件夹路径', '目标文件夹路径')
确保路径正确且目标文件夹不存在,否则会引发异常。
是否可以选择性地复制文件夹中的特定文件?
是的,shutil
模块的copytree
函数允许您通过提供ignore
参数来选择性地忽略某些文件。例如,如果您只想复制特定类型的文件,可以使用如下代码:
import shutil
import os
def ignore_patterns(path, names):
return [name for name in names if not name.endswith('.txt')] # 忽略所有非文本文件
shutil.copytree('源文件夹路径', '目标文件夹路径', ignore=ignore_patterns)
这种方式使您能够灵活控制复制的内容。
在复制文件夹时,如何处理文件冲突?
在使用shutil.copytree
时,如果目标文件夹已经存在,会引发FileExistsError
。为了避免这种情况,可以在复制之前检查目标文件夹是否存在,或者使用dirs_exist_ok
参数(Python 3.8及以上版本)来允许在目标文件夹中合并内容。例如:
shutil.copytree('源文件夹路径', '目标文件夹路径', dirs_exist_ok=True)
这样,目标文件夹中的文件将被保留,而源文件夹中的文件将被添加。
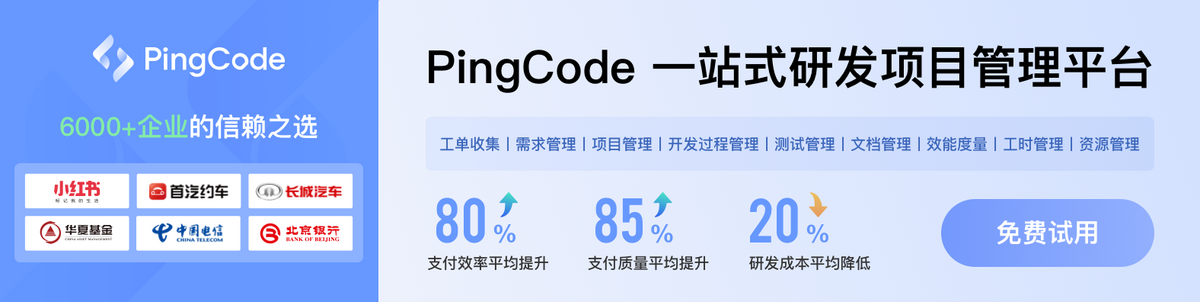