在Python中创建一个围棋板的步骤包括:使用二维数组表示棋盘、绘制棋盘、实现基本棋子操作、实现棋盘显示。 下面详细介绍如何使用Python创建一个围棋板,并涉及到每个步骤的具体实现。
一、使用二维数组表示棋盘
围棋板是一个19×19的网格,我们可以使用一个二维数组来表示。每个数组元素可以用来存储棋子的状态,例如0表示空,1表示黑子,2表示白子。
import numpy as np
class GoBoard:
def __init__(self, size=19):
self.size = size
self.board = np.zeros((size, size), dtype=int)
def display_board(self):
for row in self.board:
print(' '.join(str(cell) for cell in row))
二、绘制棋盘
绘制棋盘可以使用Python的matplotlib库来实现。matplotlib是一个强大的绘图库,可以很方便地绘制出围棋的棋盘和棋子。
import matplotlib.pyplot as plt
class GoBoard:
def __init__(self, size=19):
self.size = size
self.board = np.zeros((size, size), dtype=int)
def display_board(self):
fig, ax = plt.subplots()
ax.set_xlim(0, self.size)
ax.set_ylim(0, self.size)
for i in range(self.size):
ax.plot([0, self.size - 1], [i, i], color='black')
ax.plot([i, i], [0, self.size - 1], color='black')
for x in range(self.size):
for y in range(self.size):
if self.board[x, y] == 1:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='black')
ax.add_artist(circle)
elif self.board[x, y] == 2:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='white')
ax.add_artist(circle)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
三、实现基本棋子操作
为了让围棋板具有基本的功能,我们需要实现一些基本的棋子操作,比如放置棋子、判断合法性等。
class GoBoard:
def __init__(self, size=19):
self.size = size
self.board = np.zeros((size, size), dtype=int)
def place_stone(self, x, y, color):
if self.board[x, y] == 0:
self.board[x, y] = color
return True
return False
def is_legal_move(self, x, y):
return self.board[x, y] == 0
def display_board(self):
fig, ax = plt.subplots()
ax.set_xlim(0, self.size)
ax.set_ylim(0, self.size)
for i in range(self.size):
ax.plot([0, self.size - 1], [i, i], color='black')
ax.plot([i, i], [0, self.size - 1], color='black')
for x in range(self.size):
for y in range(self.size):
if self.board[x, y] == 1:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='black')
ax.add_artist(circle)
elif self.board[x, y] == 2:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='white')
ax.add_artist(circle)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
四、实现棋盘显示
在实现了基本的棋盘绘制和棋子操作之后,我们可以完善棋盘显示功能,使得用户可以更直观地看到棋盘的状态。
class GoBoard:
def __init__(self, size=19):
self.size = size
self.board = np.zeros((size, size), dtype=int)
def place_stone(self, x, y, color):
if self.board[x, y] == 0:
self.board[x, y] = color
return True
return False
def is_legal_move(self, x, y):
return self.board[x, y] == 0
def display_board(self):
fig, ax = plt.subplots()
ax.set_xlim(0, self.size)
ax.set_ylim(0, self.size)
for i in range(self.size):
ax.plot([0, self.size - 1], [i, i], color='black')
ax.plot([i, i], [0, self.size - 1], color='black')
for x in range(self.size):
for y in range(self.size):
if self.board[x, y] == 1:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='black')
ax.add_artist(circle)
elif self.board[x, y] == 2:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='white')
ax.add_artist(circle)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
五、增加高级功能
为了让围棋板更加实用,可以增加一些高级功能,比如检测气、判断胜负等。
class GoBoard:
def __init__(self, size=19):
self.size = size
self.board = np.zeros((size, size), dtype=int)
def place_stone(self, x, y, color):
if self.board[x, y] == 0:
self.board[x, y] = color
return True
return False
def is_legal_move(self, x, y):
return self.board[x, y] == 0
def display_board(self):
fig, ax = plt.subplots()
ax.set_xlim(0, self.size)
ax.set_ylim(0, self.size)
for i in range(self.size):
ax.plot([0, self.size - 1], [i, i], color='black')
ax.plot([i, i], [0, self.size - 1], color='black')
for x in range(self.size):
for y in range(self.size):
if self.board[x, y] == 1:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='black')
ax.add_artist(circle)
elif self.board[x, y] == 2:
circle = plt.Circle((y, self.size - x - 1), 0.4, color='white')
ax.add_artist(circle)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
def get_liberties(self, x, y):
color = self.board[x, y]
if color == 0:
return 0
visited = set()
liberties = 0
def dfs(x, y):
nonlocal liberties
if (x, y) in visited:
return
visited.add((x, y))
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
nx, ny = x + dx, y + dy
if 0 <= nx < self.size and 0 <= ny < self.size:
if self.board[nx, ny] == 0:
liberties += 1
elif self.board[nx, ny] == color:
dfs(nx, ny)
dfs(x, y)
return liberties
def remove_dead_stones(self):
for x in range(self.size):
for y in range(self.size):
if self.board[x, y] != 0 and self.get_liberties(x, y) == 0:
self.board[x, y] = 0
通过以上的步骤,我们可以使用Python创建一个基本的围棋板,具备棋盘显示、棋子操作和基本的规则检测功能。这个围棋板可以作为进一步开发的基础,添加更多的功能和优化。
相关问答FAQs:
如何用Python创建一个围棋板的基本步骤是什么?
要用Python创建一个围棋板,首先需要确定围棋板的尺寸,通常是19×19的格子。可以使用Python中的图形库,例如Pygame或Tkinter,来绘制棋盘和棋子。创建一个二维数组来表示棋盘状态,使用循环来绘制每个格子,并在适当的位置绘制棋子。
我可以使用哪些Python库来帮助创建围棋板?
推荐使用Pygame和Tkinter这两个库。Pygame适合创建游戏界面,提供丰富的图形和声音支持,非常适合围棋这样的棋类游戏。而Tkinter是Python内置的GUI库,简单易用,可以快速搭建围棋板的界面。选择合适的库可以大大简化开发过程。
在围棋板中如何实现棋子的落子功能?
为了实现棋子的落子功能,需要监听鼠标事件。当用户点击棋盘的某个位置时,可以通过计算点击位置对应的格子坐标,更新棋盘的状态,并在对应位置绘制棋子。此外,要确保落子的合法性,例如检查是否可以在该位置放置棋子,以及是否有气等规则,可以通过定义相关函数来处理这些逻辑。
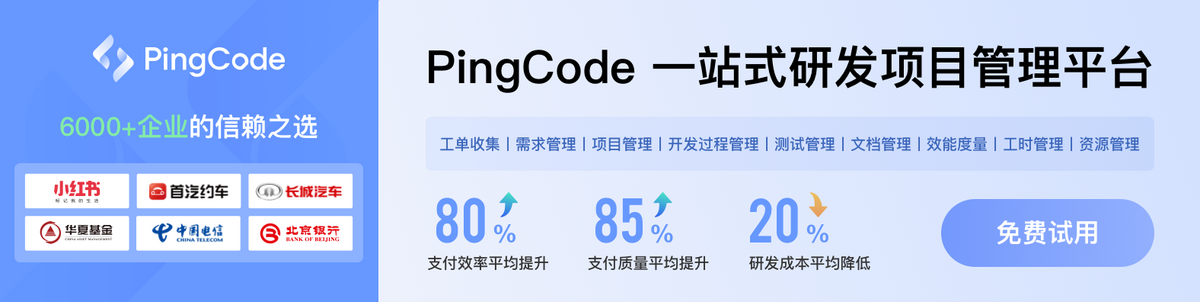