在Python中引用变量到字符串的方式有多种,包括使用格式化字符串(f-strings)、百分号格式化、和str.format()方法。这些方法都可以帮助你在字符串中插入变量。其中,f-strings 是Python 3.6及以后版本中推荐使用的方法,因为它简洁且易读。本文将详细介绍这几种方法,并给出实际应用中的示例代码。
一、f-strings(格式化字符串)
f-strings是一种在Python 3.6及以后版本中引入的字符串格式化方法,使用起来非常简洁和直观。通过在字符串前面加上字母f
或F
,然后在字符串内部使用大括号{}
包裹变量名或表达式,即可将变量插入到字符串中。
name = "Alice"
age = 30
message = f"Hello, my name is {name} and I am {age} years old."
print(message)
在上面的示例中,name
和age
变量被直接插入到了字符串中,得到的输出是:
Hello, my name is Alice and I am 30 years old.
f-strings不仅可以插入变量,还可以插入表达式,例如:
number = 10
message = f"The square of {number} is {number 2}."
print(message)
输出结果为:
The square of 10 is 100.
二、百分号格式化
在早期的Python版本中,百分号%
格式化是一种常见的字符串插入方法。这种方法使用%
符号和格式说明符来表示需要插入的变量位置。
name = "Bob"
age = 25
message = "Hello, my name is %s and I am %d years old." % (name, age)
print(message)
在这个示例中,%s
和%d
分别是字符串和整数的格式说明符,它们将被name
和age
变量的值替换,最终输出结果为:
Hello, my name is Bob and I am 25 years old.
虽然百分号格式化方法比较老旧,但在某些场景下仍然会用到。
三、str.format()方法
str.format()方法是Python 2.7及以后版本中引入的一种字符串格式化方法,它通过调用字符串的format()
方法,并在字符串中使用大括号{}
来插入变量。
name = "Charlie"
age = 35
message = "Hello, my name is {} and I am {} years old.".format(name, age)
print(message)
在这个示例中,format()
方法将name
和age
变量的值插入到字符串中的大括号位置,最终输出结果为:
Hello, my name is Charlie and I am 35 years old.
此外,str.format()
方法还支持带有索引和命名的占位符:
name = "David"
age = 40
message = "Hello, my name is {0} and I am {1} years old.".format(name, age)
print(message)
message = "Hello, my name is {name} and I am {age} years old.".format(name=name, age=age)
print(message)
这两种方式的输出结果分别为:
Hello, my name is David and I am 40 years old.
Hello, my name is David and I am 40 years old.
四、模板字符串(Template Strings)
模板字符串是Python标准库中的string
模块提供的一种字符串格式化方法,适用于需要更复杂字符串模板的情况。模板字符串使用$
符号作为占位符,并通过Template
类的substitute()
方法来插入变量。
from string import Template
name = "Eve"
age = 28
template = Template("Hello, my name is $name and I am $age years old.")
message = template.substitute(name=name, age=age)
print(message)
在这个示例中,Template
类的substitute()
方法将name
和age
变量的值插入到字符串中的占位符位置,最终输出结果为:
Hello, my name is Eve and I am 28 years old.
五、小结
在Python中引用变量到字符串的方式主要有以下几种:f-strings、百分号格式化、str.format()方法、模板字符串。其中,f-strings是Python 3.6及以后版本中推荐使用的方法,因为它简洁且易读。每种方法都有其优缺点,选择适合自己需求的方式即可。了解这些方法可以帮助你在不同场景中更灵活地处理字符串格式化问题。
六、实战案例
案例一:动态生成文件名
在许多应用场景中,需要动态生成文件名,例如根据日期时间生成日志文件名。使用字符串插入方法,可以轻松实现这一需求。
from datetime import datetime
current_time = datetime.now().strftime("%Y%m%d_%H%M%S")
file_name = f"log_{current_time}.txt"
print(file_name)
在这个示例中,使用datetime
模块获取当前日期时间,并通过strftime()
方法将其格式化为YYYYMMDD_HHMMSS
格式,然后使用f-strings将其插入到文件名字符串中,最终生成的文件名类似于:
log_20230401_153045.txt
案例二:生成SQL查询语句
在数据库操作中,常常需要动态生成SQL查询语句。例如,根据用户输入的条件生成相应的查询语句。使用字符串插入方法,可以方便地构建SQL查询语句。
table = "users"
columns = ["id", "name", "email"]
conditions = "age > 30"
query = f"SELECT {', '.join(columns)} FROM {table} WHERE {conditions};"
print(query)
在这个示例中,通过f-strings将表名、列名和条件插入到SQL查询字符串中,最终生成的查询语句为:
SELECT id, name, email FROM users WHERE age > 30;
案例三:生成HTML内容
在Web开发中,常常需要动态生成HTML内容。例如,根据用户输入的数据生成一个HTML表格。使用字符串插入方法,可以方便地构建HTML内容。
data = [
{"name": "Alice", "age": 30},
{"name": "Bob", "age": 25},
{"name": "Charlie", "age": 35}
]
rows = ""
for item in data:
rows += f"<tr><td>{item['name']}</td><td>{item['age']}</td></tr>"
html = f"""
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
{rows}
</table>
"""
print(html)
在这个示例中,通过f-strings将数据插入到HTML表格字符串中,最终生成的HTML内容为:
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr><td>Alice</td><td>30</td></tr><tr><td>Bob</td><td>25</td></tr><tr><td>Charlie</td><td>35</td></tr>
</table>
七、注意事项
- 安全性:在处理用户输入的数据时,特别是在生成SQL查询语句时,要特别注意防止SQL注入攻击。应使用参数化查询或ORM工具来生成安全的SQL查询语句。
- 性能:在需要高性能的场景中,选择合适的字符串插入方法。例如,f-strings在大多数情况下性能较好,但在大量字符串拼接时,
join()
方法可能更高效。 - 兼容性:在选择字符串插入方法时,要考虑Python版本的兼容性。例如,f-strings仅适用于Python 3.6及以后版本。
八、总结
本文介绍了Python中引用变量到字符串的几种常用方法,包括f-strings、百分号格式化、str.format()方法和模板字符串。通过对比这些方法的优缺点,以及实际应用中的示例代码,帮助你更好地理解和选择适合自己需求的字符串插入方法。在实际开发中,根据具体需求和场景选择合适的方法,能够提高代码的可读性和维护性。
相关问答FAQs:
如何在Python字符串中插入变量?
在Python中,可以通过多种方式在字符串中插入变量。最常用的方法包括使用格式化字符串(f-strings)、str.format()
方法以及百分号格式化(%)。例如,使用f-strings的方式是name = "Alice"; greeting = f"Hello, {name}!"
,这样可以直接在字符串中插入变量的值。
在Python中使用字符串拼接时需要注意什么?
使用字符串拼接时,确保所有要拼接的内容都是字符串类型。如果变量是其他数据类型,可以使用str()
函数将其转换为字符串。例如,age = 25; message = "I am " + str(age) + " years old."
。这样可以避免类型错误。
Python中使用模板字符串有什么优势?
模板字符串(Template Strings)提供了一种更加安全和可读的方式来插入变量。使用string
模块中的Template
类,可以通过$
符号来引用变量,例如:from string import Template; t = Template("Hello, $name"); result = t.substitute(name="Bob")
。这种方法非常适合需要处理用户输入的场景,能有效避免注入攻击。
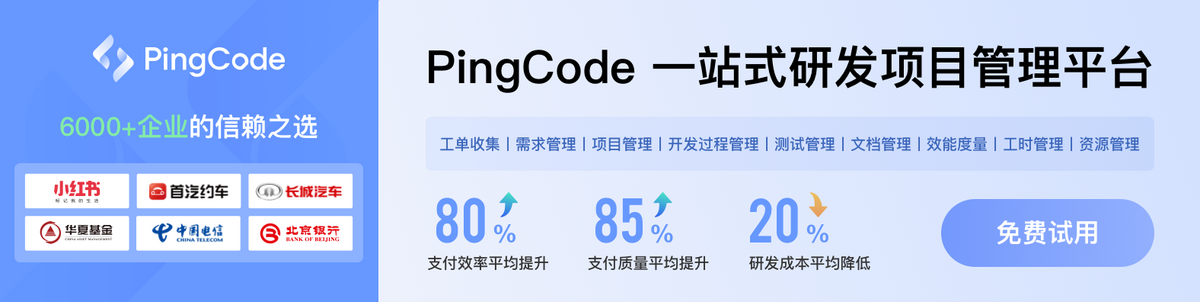