在Python的类中实现多线程,可以通过使用threading
模块来创建和管理线程。创建线程类、继承Thread类、实现run方法、使用锁机制来避免线程间竞争是实现多线程的重要步骤。下面将详细介绍如何在Python的类中实现多线程,并对其中一个核心点——使用锁机制来避免线程间竞争进行详细描述。
使用锁机制来避免线程间竞争是多线程编程中的一个关键点。在多线程环境下,多个线程可能会同时访问和修改共享资源,这可能会导致数据不一致或冲突。通过使用锁机制,可以确保在同一时刻只有一个线程可以访问共享资源,从而避免竞争条件。Python提供了threading.Lock
类来实现锁机制。我们可以在线程执行的关键代码段前后加上锁的获取和释放操作,从而确保线程的安全性。
一、创建线程类
为了在Python的类中实现多线程,我们首先需要创建一个线程类。这个线程类可以继承自threading.Thread
类,并重写其run
方法。run
方法是线程的入口点,当线程启动时,run
方法中的代码将被执行。以下是一个简单的线程类示例:
import threading
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
def run(self):
print(f"Starting thread: {self.name}")
# 在此处添加线程执行的代码
print(f"Exiting thread: {self.name}")
在上述代码中,我们创建了一个名为MyThread
的类,它继承自threading.Thread
类,并重写了run
方法。run
方法中包含了线程执行的代码。
二、创建线程并启动
一旦创建了线程类,我们就可以创建线程对象并启动线程。以下是一个示例:
# 创建新线程
thread1 = MyThread(1, "Thread-1", 1)
thread2 = MyThread(2, "Thread-2", 2)
启动线程
thread1.start()
thread2.start()
在上述代码中,我们创建了两个线程对象thread1
和thread2
,并调用它们的start
方法来启动线程。当线程启动时,它们将执行run
方法中的代码。
三、使用锁机制来避免线程间竞争
在多线程编程中,多个线程可能会同时访问和修改共享资源,导致数据不一致或冲突。为了避免这种情况,我们可以使用锁机制。以下是一个示例,演示如何使用锁机制来保护共享资源:
import threading
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter, lock):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
self.lock = lock
def run(self):
print(f"Starting thread: {self.name}")
# 获取锁
self.lock.acquire()
try:
# 在此处添加线程执行的代码
print(f"Thread {self.name} is running")
finally:
# 释放锁
self.lock.release()
print(f"Exiting thread: {self.name}")
创建锁对象
lock = threading.Lock()
创建新线程
thread1 = MyThread(1, "Thread-1", 1, lock)
thread2 = MyThread(2, "Thread-2", 2, lock)
启动线程
thread1.start()
thread2.start()
在上述代码中,我们创建了一个锁对象lock
,并将其传递给线程类。在run
方法中,我们在访问共享资源之前获取锁,并在访问完成后释放锁。通过这种方式,我们可以确保在同一时刻只有一个线程可以访问共享资源,从而避免竞争条件。
四、线程同步
在某些情况下,我们可能需要确保多个线程按照特定的顺序执行。为此,我们可以使用线程同步机制,例如事件、条件变量和信号量。以下是一个示例,演示如何使用事件来实现线程同步:
import threading
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter, event):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
self.event = event
def run(self):
print(f"Starting thread: {self.name}")
# 等待事件
self.event.wait()
# 在此处添加线程执行的代码
print(f"Thread {self.name} is running")
print(f"Exiting thread: {self.name}")
创建事件对象
event = threading.Event()
创建新线程
thread1 = MyThread(1, "Thread-1", 1, event)
thread2 = MyThread(2, "Thread-2", 2, event)
启动线程
thread1.start()
thread2.start()
设置事件
event.set()
在上述代码中,我们创建了一个事件对象event
,并将其传递给线程类。在run
方法中,我们调用event.wait
方法来等待事件被设置。通过调用event.set
方法,我们可以通知等待的线程继续执行。通过这种方式,我们可以实现线程同步,确保线程按照特定的顺序执行。
五、线程池
在多线程编程中,我们可能需要创建和管理大量的线程。为了简化这种情况,我们可以使用线程池。Python提供了concurrent.futures.ThreadPoolExecutor
类来创建和管理线程池。以下是一个示例,演示如何使用线程池:
import concurrent.futures
def task(name):
print(f"Task {name} is running")
创建线程池
with concurrent.futures.ThreadPoolExecutor(max_workers=2) as executor:
# 提交任务
future1 = executor.submit(task, "Task-1")
future2 = executor.submit(task, "Task-2")
# 等待任务完成
concurrent.futures.wait([future1, future2])
在上述代码中,我们创建了一个线程池对象executor
,并使用submit
方法提交任务。通过调用concurrent.futures.wait
方法,我们可以等待所有任务完成。线程池可以帮助我们有效地管理和调度线程,避免创建和销毁大量线程的开销。
六、守护线程
守护线程是一种特殊的线程,它会在主线程结束时自动结束。为了创建守护线程,我们可以在启动线程之前调用setDaemon
方法或将daemon
属性设置为True
。以下是一个示例:
import threading
import time
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
def run(self):
print(f"Starting thread: {self.name}")
while True:
print(f"Thread {self.name} is running")
time.sleep(1)
创建新线程
thread1 = MyThread(1, "Thread-1", 1)
thread1.setDaemon(True)
启动线程
thread1.start()
主线程执行其他操作
time.sleep(5)
print("Main thread exiting")
在上述代码中,我们创建了一个守护线程thread1
,并调用setDaemon
方法将其设置为守护线程。守护线程将在主线程结束时自动结束。
七、线程安全的数据结构
在多线程编程中,我们可能需要使用线程安全的数据结构来管理共享数据。Python提供了一些线程安全的数据结构,例如queue.Queue
。以下是一个示例,演示如何使用queue.Queue
来管理线程之间的通信:
import threading
import queue
class Producer(threading.Thread):
def __init__(self, thread_id, name, data_queue):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.data_queue = data_queue
def run(self):
print(f"Starting producer: {self.name}")
for i in range(5):
item = f"Item-{i}"
self.data_queue.put(item)
print(f"Producer {self.name} produced {item}")
print(f"Exiting producer: {self.name}")
class Consumer(threading.Thread):
def __init__(self, thread_id, name, data_queue):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.data_queue = data_queue
def run(self):
print(f"Starting consumer: {self.name}")
while True:
item = self.data_queue.get()
if item is None:
break
print(f"Consumer {self.name} consumed {item}")
print(f"Exiting consumer: {self.name}")
创建队列对象
data_queue = queue.Queue()
创建生产者和消费者线程
producer = Producer(1, "Producer-1", data_queue)
consumer = Consumer(2, "Consumer-1", data_queue)
启动线程
producer.start()
consumer.start()
等待生产者线程完成
producer.join()
向队列添加None,以通知消费者线程退出
data_queue.put(None)
等待消费者线程完成
consumer.join()
在上述代码中,我们创建了一个线程安全的队列对象data_queue
,并将其传递给生产者和消费者线程。生产者线程将数据添加到队列中,消费者线程从队列中获取数据。通过使用线程安全的数据结构,我们可以简化多线程编程中的数据管理和通信。
八、线程的状态管理
在多线程编程中,我们可能需要管理线程的状态,例如启动、暂停、恢复和终止线程。以下是一个示例,演示如何管理线程的状态:
import threading
import time
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
self.paused = threading.Event()
self.paused.set()
self.stopped = threading.Event()
def run(self):
print(f"Starting thread: {self.name}")
while not self.stopped.is_set():
self.paused.wait()
print(f"Thread {self.name} is running")
time.sleep(1)
print(f"Exiting thread: {self.name}")
def pause(self):
self.paused.clear()
def resume(self):
self.paused.set()
def stop(self):
self.stopped.set()
创建新线程
thread1 = MyThread(1, "Thread-1", 1)
启动线程
thread1.start()
主线程执行其他操作
time.sleep(3)
thread1.pause()
print("Thread paused")
time.sleep(3)
thread1.resume()
print("Thread resumed")
time.sleep(3)
thread1.stop()
print("Thread stopped")
在上述代码中,我们创建了一个名为MyThread
的类,并添加了pause
、resume
和stop
方法来管理线程的状态。通过使用事件对象paused
和stopped
,我们可以控制线程的执行、暂停和终止。
九、线程异常处理
在多线程编程中,我们可能需要处理线程中的异常。以下是一个示例,演示如何在线程中处理异常:
import threading
class MyThread(threading.Thread):
def __init__(self, thread_id, name, counter):
threading.Thread.__init__(self)
self.thread_id = thread_id
self.name = name
self.counter = counter
def run(self):
print(f"Starting thread: {self.name}")
try:
# 在此处添加线程执行的代码
raise ValueError("An error occurred")
except Exception as e:
print(f"Exception in thread {self.name}: {e}")
print(f"Exiting thread: {self.name}")
创建新线程
thread1 = MyThread(1, "Thread-1", 1)
启动线程
thread1.start()
在上述代码中,我们在run
方法中添加了异常处理代码,通过使用try
和except
块,我们可以捕获并处理线程中的异常。
十、总结
在Python的类中实现多线程可以通过使用threading
模块来创建和管理线程。创建线程类、继承Thread
类、实现run
方法、使用锁机制来避免线程间竞争是实现多线程的重要步骤。此外,我们还可以使用线程同步机制、线程池、守护线程、线程安全的数据结构、线程状态管理和线程异常处理来实现更加复杂和健壮的多线程应用。
通过这些方法,我们可以充分利用多核处理器的优势,提高程序的并发性能和响应速度。在实际应用中,我们需要根据具体需求选择合适的多线程编程技术,并注意线程安全和竞争条件的处理。
相关问答FAQs:
在Python的类中使用多线程的好处是什么?
在Python的类中实现多线程可以有效提升程序的效率,尤其是在处理I/O密集型任务时。多线程允许程序同时执行多个操作,减少等待时间,从而提高响应速度。此外,使用类结构可以使代码更加模块化,易于维护和扩展。
在Python中实现多线程时需要注意哪些问题?
实现多线程时,需关注线程安全问题。多个线程可能会同时访问共享资源,导致数据不一致或程序崩溃。因此,使用锁(如threading.Lock
)来保护共享资源是一个重要的做法。此外,还需考虑线程的生命周期管理,确保线程在完成任务后被正确关闭,以避免资源泄露。
如何确保在多线程环境中数据的一致性?
为了确保数据一致性,可以使用锁机制或其他同步工具(如Semaphore
和Event
)。使用锁可以在某一时刻只允许一个线程访问特定的共享资源,从而避免竞争条件。此外,使用队列(如queue.Queue
)来在线程之间安全地传递数据也是一种有效的方法,可以确保数据的有序处理和一致性。
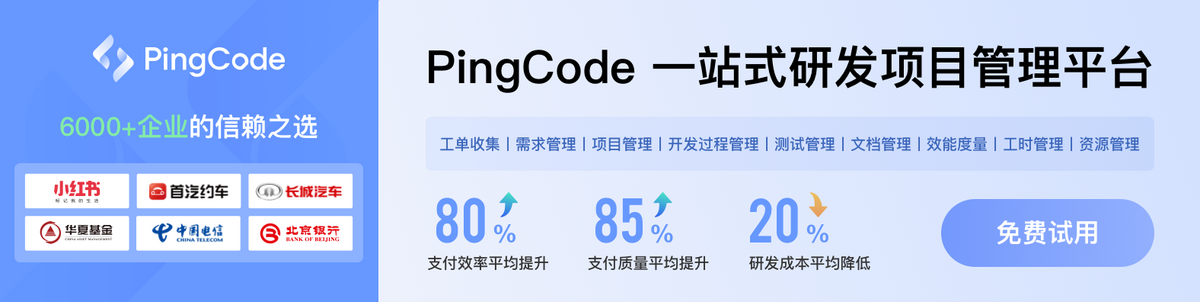