Python 读取 7z 文件的方法有多种,包括使用 py7zr
库、pyunpack
和 patool
库等。我们将重点介绍使用 py7zr
库的方法。
首先,使用 py7zr
库是读取 7z 文件的推荐方式,因为它是专门用于处理 7z 文件的库。使用 py7zr
库,可以方便地读取、解压缩和操作 7z 文件。
一、安装和导入必要的库
要使用 py7zr
库,首先需要安装它,可以使用以下命令安装:
pip install py7zr
安装完成后,在 Python 脚本中导入该库:
import py7zr
二、读取 7z 文件内容
读取 7z 文件的基本步骤包括打开文件、解压缩文件和读取文件内容。以下是详细步骤:
- 打开 7z 文件:使用
py7zr.SevenZipFile
类打开 7z 文件。 - 解压缩文件:使用
extractall
方法将文件解压到指定目录。 - 读取文件内容:可以通过标准的文件读取方法读取解压后的文件内容。
三、代码示例
以下是一个完整的示例,展示如何使用 py7zr
库读取 7z 文件中的内容:
import py7zr
import os
def extract_7z_file(archive_path, extract_to):
with py7zr.SevenZipFile(archive_path, mode='r') as archive:
archive.extractall(path=extract_to)
def read_file_content(file_path):
with open(file_path, 'r') as file:
return file.read()
def main():
archive_path = 'path/to/your/archive.7z'
extract_to = 'path/to/extracted/files'
# 创建解压目录(如果不存在)
os.makedirs(extract_to, exist_ok=True)
# 解压 7z 文件
extract_7z_file(archive_path, extract_to)
# 假设 7z 文件中有一个名为 'example.txt' 的文件
extracted_file_path = os.path.join(extract_to, 'example.txt')
# 读取解压后的文件内容
content = read_file_content(extracted_file_path)
print(content)
if __name__ == '__main__':
main()
四、处理多个文件
如果 7z 文件中包含多个文件,可以使用 py7zr
库的 getnames
方法获取文件名列表,然后逐个读取文件内容:
def extract_7z_file(archive_path, extract_to):
with py7zr.SevenZipFile(archive_path, mode='r') as archive:
archive.extractall(path=extract_to)
def read_file_content(file_path):
with open(file_path, 'r') as file:
return file.read()
def main():
archive_path = 'path/to/your/archive.7z'
extract_to = 'path/to/extracted/files'
# 创建解压目录(如果不存在)
os.makedirs(extract_to, exist_ok=True)
# 解压 7z 文件
with py7zr.SevenZipFile(archive_path, mode='r') as archive:
archive.extractall(path=extract_to)
file_names = archive.getnames()
# 逐个读取文件内容
for file_name in file_names:
extracted_file_path = os.path.join(extract_to, file_name)
if os.path.isfile(extracted_file_path):
content = read_file_content(extracted_file_path)
print(f'Content of {file_name}:\n{content}\n')
if __name__ == '__main__':
main()
五、处理大文件和内存优化
在处理大文件时,解压和读取文件的过程可能会消耗大量内存。可以使用流式读取的方法来优化内存使用:
import py7zr
import os
def extract_7z_file(archive_path, extract_to):
with py7zr.SevenZipFile(archive_path, mode='r') as archive:
archive.extractall(path=extract_to)
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
print(line.strip())
def main():
archive_path = 'path/to/your/archive.7z'
extract_to = 'path/to/extracted/files'
# 创建解压目录(如果不存在)
os.makedirs(extract_to, exist_ok=True)
# 解压 7z 文件
with py7zr.SevenZipFile(archive_path, mode='r') as archive:
archive.extractall(path=extract_to)
file_names = archive.getnames()
# 逐个读取文件内容(流式读取大文件)
for file_name in file_names:
extracted_file_path = os.path.join(extract_to, file_name)
if os.path.isfile(extracted_file_path):
print(f'Reading content of {file_name}:')
read_large_file(extracted_file_path)
if __name__ == '__main__':
main()
六、处理压缩加密的 7z 文件
如果 7z 文件是加密的,您需要提供密码才能解压缩文件。 py7zr
库支持处理加密文件:
import py7zr
import os
def extract_7z_file(archive_path, extract_to, password):
with py7zr.SevenZipFile(archive_path, mode='r', password=password) as archive:
archive.extractall(path=extract_to)
def read_file_content(file_path):
with open(file_path, 'r') as file:
return file.read()
def main():
archive_path = 'path/to/your/encrypted_archive.7z'
extract_to = 'path/to/extracted/files'
password = 'your_password'
# 创建解压目录(如果不存在)
os.makedirs(extract_to, exist_ok=True)
# 解压加密的 7z 文件
extract_7z_file(archive_path, extract_to, password)
# 假设 7z 文件中有一个名为 'example.txt' 的文件
extracted_file_path = os.path.join(extract_to, 'example.txt')
# 读取解压后的文件内容
content = read_file_content(extracted_file_path)
print(content)
if __name__ == '__main__':
main()
七、总结
通过上述方法,您可以使用 Python 轻松读取和处理 7z 文件。使用 py7zr
库,您可以方便地打开、解压和读取 7z 文件中的内容。此外,处理大文件和加密文件时,您可以使用流式读取和提供密码的方法。希望这些示例代码对您有所帮助。如果您需要处理其他类型的压缩文件,可以考虑使用 pyunpack
和 patool
库,它们支持更多的压缩格式。
相关问答FAQs:
如何在Python中安装处理7z文件所需的库?
要在Python中读取7z文件,首先需要安装py7zr
库。你可以使用pip进行安装,运行命令pip install py7zr
。安装后,便可以使用此库来解压和读取7z文件内的内容。
读取7z文件时,是否需要解压缩整个文件?
不需要在读取7z文件时解压缩整个文件。使用py7zr
库,你可以直接读取文件中的特定条目,从而节省时间和存储空间。例如,你可以指定要提取的文件名,而不是解压缩整个归档。
在Python中读取7z文件时如何处理编码问题?
在处理7z文件的内容时,可能会遇到编码问题。确保在读取文件时使用正确的编码格式,例如UTF-8。如果你在读取文本文件时遇到乱码,可以尝试指定其他编码方式,如utf-16
或latin1
,以确保文本能够正确解码。
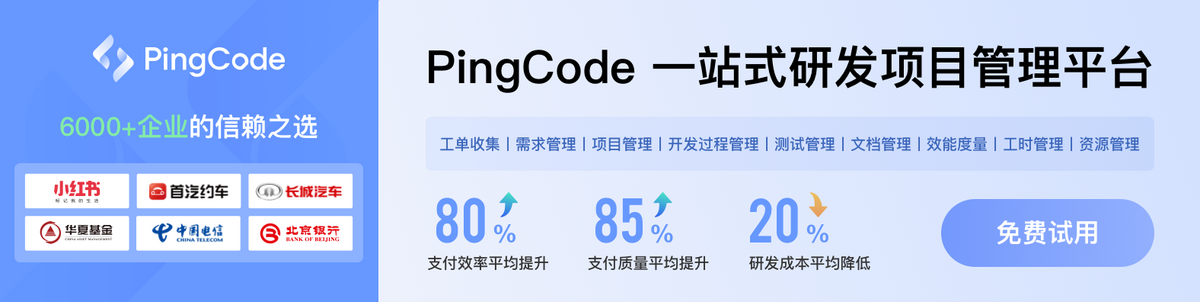