Python将代码转换成界面的步骤包括:使用图形用户界面(GUI)库、设计界面布局、编写事件处理函数。 其中,使用图形用户界面(GUI)库是实现界面的基础。Python提供了许多GUI库,最常用的有Tkinter、PyQt和Kivy等。下面我将详细介绍如何使用这些库来将代码转换成界面。
一、Tkinter库
Tkinter是Python的标准GUI库,随Python安装包一起分发。它是一个轻量级的库,适用于简单的GUI应用程序。
1. 导入Tkinter库
首先,我们需要导入Tkinter库。以下是导入Tkinter库的代码:
import tkinter as tk
from tkinter import ttk
2. 创建主窗口
创建主窗口是每个Tkinter应用程序的起点。以下是创建主窗口的代码:
root = tk.Tk()
root.title("My Tkinter Application")
root.geometry("400x300")
3. 添加控件
在主窗口中添加控件,如按钮、标签和文本框等。以下是添加控件的示例代码:
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
button = tk.Button(root, text="Click Me", command=lambda: print("Button clicked!"))
button.pack()
4. 运行主循环
最后,运行主循环来显示窗口并等待用户交互。以下是运行主循环的代码:
root.mainloop()
二、PyQt库
PyQt是一个功能强大的GUI库,适用于需要更多功能和复杂界面的应用程序。它基于Qt库,提供了丰富的控件和强大的布局管理。
1. 安装PyQt库
首先,需要安装PyQt库,可以使用pip命令安装:
pip install PyQt5
2. 导入PyQt库
导入PyQt库的代码如下:
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QVBoxLayout, QWidget
3. 创建主窗口
创建主窗口的代码如下:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My PyQt Application")
self.setGeometry(100, 100, 400, 300)
layout = QVBoxLayout()
label = QLabel("Hello, PyQt!")
layout.addWidget(label)
button = QPushButton("Click Me")
button.clicked.connect(lambda: print("Button clicked!"))
layout.addWidget(button)
container = QWidget()
container.setLayout(layout)
self.setCentralWidget(container)
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
三、Kivy库
Kivy是一个开源的Python库,专为多点触控界面设计,适用于跨平台应用程序(Windows、Linux、macOS、Android和iOS)。
1. 安装Kivy库
首先,需要安装Kivy库,可以使用pip命令安装:
pip install kivy
2. 导入Kivy库
导入Kivy库的代码如下:
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
3. 创建主窗口
创建主窗口的代码如下:
class MyApp(App):
def build(self):
layout = BoxLayout(orientation='vertical')
label = Label(text="Hello, Kivy!")
layout.add_widget(label)
button = Button(text="Click Me")
button.bind(on_press=lambda instance: print("Button clicked!"))
layout.add_widget(button)
return layout
if __name__ == "__main__":
MyApp().run()
四、设计界面布局
设计界面布局是将代码转换成界面的关键步骤。无论使用哪种GUI库,都需要合理安排控件的位置和大小,以确保用户体验良好。
1. 布局管理器
大多数GUI库提供了布局管理器,用于自动排列控件。常见的布局管理器有垂直布局、水平布局和网格布局等。以下是Tkinter中使用布局管理器的示例代码:
import tkinter as tk
root = tk.Tk()
root.title("My Tkinter Application")
root.geometry("400x300")
frame = tk.Frame(root)
frame.pack(fill=tk.BOTH, expand=True)
label = tk.Label(frame, text="Hello, Tkinter!")
label.pack(side=tk.TOP, padx=10, pady=10)
button = tk.Button(frame, text="Click Me", command=lambda: print("Button clicked!"))
button.pack(side=tk.BOTTOM, padx=10, pady=10)
root.mainloop()
2. 自定义布局
有时,默认的布局管理器无法满足需求,这时可以自定义布局。例如,在Kivy中,可以使用相对布局来精确控制控件的位置和大小:
from kivy.app import App
from kivy.uix.floatlayout import FloatLayout
from kivy.uix.label import Label
from kivy.uix.button import Button
class MyApp(App):
def build(self):
layout = FloatLayout()
label = Label(text="Hello, Kivy!", size_hint=(0.2, 0.1), pos_hint={'x': 0.4, 'y': 0.8})
layout.add_widget(label)
button = Button(text="Click Me", size_hint=(0.2, 0.1), pos_hint={'x': 0.4, 'y': 0.6})
button.bind(on_press=lambda instance: print("Button clicked!"))
layout.add_widget(button)
return layout
if __name__ == "__main__":
MyApp().run()
五、编写事件处理函数
事件处理函数是响应用户交互的核心。例如,当用户点击按钮时,程序应执行相应的操作。在GUI库中,可以通过绑定事件处理函数来实现这一点。
1. Tkinter中的事件处理
在Tkinter中,可以使用command
参数绑定事件处理函数。例如:
import tkinter as tk
def on_button_click():
print("Button clicked!")
root = tk.Tk()
root.title("My Tkinter Application")
root.geometry("400x300")
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack(padx=10, pady=10)
root.mainloop()
2. PyQt中的事件处理
在PyQt中,可以使用connect
方法绑定事件处理函数。例如:
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QVBoxLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My PyQt Application")
self.setGeometry(100, 100, 400, 300)
layout = QVBoxLayout()
button = QPushButton("Click Me")
button.clicked.connect(self.on_button_click)
layout.addWidget(button)
container = QWidget()
container.setLayout(layout)
self.setCentralWidget(container)
def on_button_click(self):
print("Button clicked!")
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
3. Kivy中的事件处理
在Kivy中,可以使用bind
方法绑定事件处理函数。例如:
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
class MyApp(App):
def build(self):
layout = BoxLayout(orientation='vertical')
label = Label(text="Hello, Kivy!")
layout.add_widget(label)
button = Button(text="Click Me")
button.bind(on_press=self.on_button_click)
layout.add_widget(button)
return layout
def on_button_click(self, instance):
print("Button clicked!")
if __name__ == "__main__":
MyApp().run()
六、综合示例
下面是一个综合示例,将前面介绍的所有步骤整合在一起,展示如何使用Tkinter将Python代码转换成界面。
import tkinter as tk
from tkinter import ttk
class MyApp:
def __init__(self, root):
self.root = root
self.root.title("My Tkinter Application")
self.root.geometry("400x300")
self.create_widgets()
def create_widgets(self):
frame = tk.Frame(self.root)
frame.pack(fill=tk.BOTH, expand=True)
self.label = tk.Label(frame, text="Hello, Tkinter!")
self.label.pack(side=tk.TOP, padx=10, pady=10)
self.entry = tk.Entry(frame)
self.entry.pack(side=tk.TOP, padx=10, pady=10)
button = tk.Button(frame, text="Click Me", command=self.on_button_click)
button.pack(side=tk.BOTTOM, padx=10, pady=10)
def on_button_click(self):
text = self.entry.get()
self.label.config(text=f"Hello, {text}!")
if __name__ == "__main__":
root = tk.Tk()
app = MyApp(root)
root.mainloop()
以上代码展示了一个完整的Tkinter应用程序,包括主窗口、布局管理、控件添加和事件处理。用户可以在文本框中输入文本,并点击按钮将输入的文本显示在标签上。
通过以上步骤,我们可以将Python代码转换成界面,创建具有良好用户体验的GUI应用程序。无论选择Tkinter、PyQt还是Kivy,每种库都有其独特的特点和适用场景,开发者可以根据具体需求选择合适的库。
相关问答FAQs:
如何使用Python创建图形用户界面(GUI)?
Python提供了多个库来创建图形用户界面,其中最常用的是Tkinter、PyQt和Kivy。Tkinter是Python的标准GUI库,易于使用,适合初学者。你可以通过导入Tkinter模块,创建窗口、按钮和其他界面元素。PyQt则功能更强大,适合开发复杂的应用程序,而Kivy则适用于多平台开发,特别是移动设备。
有哪些工具可以将Python代码转化为独立的桌面应用程序?
要将Python代码打包为独立的桌面应用程序,可以使用工具如PyInstaller、cx_Freeze和py2exe。这些工具能够将Python脚本转换为可执行文件,用户无需安装Python环境即可运行。使用这些工具时,你需要指定要打包的脚本及其依赖项。
在转换Python代码为GUI时,有哪些最佳实践?
在转换Python代码为GUI时,遵循一些最佳实践可以提高应用的可用性和用户体验。保持界面简洁直观,确保所有功能易于访问。使用一致的布局和风格,方便用户理解。考虑使用事件驱动编程,确保界面响应用户操作,同时进行充分的错误处理,以提升应用的稳定性。
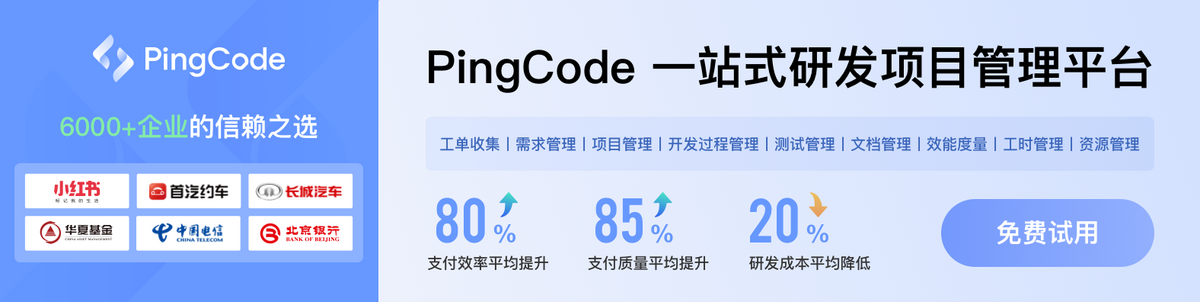