在Python中,四元数(Quaternion)是一种用来表示三维空间旋转的数学工具。、四元数转换可以通过几个步骤实现:、使用scipy库和numpy库能够简化这个过程。、以下是详细的解释和示例代码。 我们将通过定义四元数类、实现基本的四元数操作以及展示如何将四元数转换成旋转矩阵、欧拉角和轴角表示。
四元数转换的基本操作
四元数是由一个实部和三个虚部组成的复数,通常表示为 q = w + xi + yj + zk,其中w, x, y, z是实数。四元数可以用于表示和计算三维旋转。接下来,我们将探讨四元数的转换方法。
一、定义四元数类
为了更方便地操作四元数,我们可以定义一个四元数类。这个类将包含表示四元数的基本属性和方法。
import numpy as np
class Quaternion:
def __init__(self, w, x, y, z):
self.w = w
self.x = x
self.y = y
self.z = z
def __repr__(self):
return f"Quaternion({self.w}, {self.x}, {self.y}, {self.z})"
def to_array(self):
return np.array([self.w, self.x, self.y, self.z])
def norm(self):
return np.sqrt(self.w<strong>2 + self.x</strong>2 + self.y<strong>2 + self.z</strong>2)
def normalize(self):
n = self.norm()
self.w /= n
self.x /= n
self.y /= n
self.z /= n
return self
def conjugate(self):
return Quaternion(self.w, -self.x, -self.y, -self.z)
二、四元数乘法
四元数的乘法是一个重要的操作,用于组合旋转。四元数的乘法不是交换的,即 q1 * q2 ≠ q2 * q1。
def quaternion_multiply(q1, q2):
w1, x1, y1, z1 = q1.to_array()
w2, x2, y2, z2 = q2.to_array()
w = w1 * w2 - x1 * x2 - y1 * y2 - z1 * z2
x = w1 * x2 + x1 * w2 + y1 * z2 - z1 * y2
y = w1 * y2 - x1 * z2 + y1 * w2 + z1 * x2
z = w1 * z2 + x1 * y2 - y1 * x2 + z1 * w2
return Quaternion(w, x, y, z)
三、将四元数转换为旋转矩阵
旋转矩阵是另一种表示三维旋转的方式,可以从四元数中推导出来。
def quaternion_to_rotation_matrix(q):
q = q.normalize()
w, x, y, z = q.to_array()
R = np.array([
[1 - 2*y<strong>2 - 2*z</strong>2, 2*x*y - 2*z*w, 2*x*z + 2*y*w],
[2*x*y + 2*z*w, 1 - 2*x<strong>2 - 2*z</strong>2, 2*y*z - 2*x*w],
[2*x*z - 2*y*w, 2*y*z + 2*x*w, 1 - 2*x<strong>2 - 2*y</strong>2]
])
return R
四、将四元数转换为欧拉角
欧拉角是另一种表示旋转的方法,通常以三个角度表示绕不同轴的旋转。
def quaternion_to_euler(q):
q = q.normalize()
w, x, y, z = q.to_array()
t0 = +2.0 * (w * x + y * z)
t1 = +1.0 - 2.0 * (x * x + y * y)
roll_x = np.arctan2(t0, t1)
t2 = +2.0 * (w * y - z * x)
t2 = np.clip(t2, -1.0, +1.0)
pitch_y = np.arcsin(t2)
t3 = +2.0 * (w * z + x * y)
t4 = +1.0 - 2.0 * (y * y + z * z)
yaw_z = np.arctan2(t3, t4)
return roll_x, pitch_y, yaw_z
五、将四元数转换为轴角表示
轴角表示也是一种常见的旋转表示方法,包含一个旋转轴和一个旋转角度。
def quaternion_to_axis_angle(q):
q = q.normalize()
w, x, y, z = q.to_array()
angle = 2 * np.arccos(w)
s = np.sqrt(1 - w2)
if s < 1e-8:
x = 1
y = 0
z = 0
else:
x /= s
y /= s
z /= s
return (x, y, z), angle
六、从旋转矩阵、欧拉角和轴角生成四元数
除了将四元数转换为其他表示形式,我们还可以从其他表示形式生成四元数。
def rotation_matrix_to_quaternion(R):
trace = np.trace(R)
if trace > 0:
s = 0.5 / np.sqrt(trace + 1.0)
w = 0.25 / s
x = (R[2, 1] - R[1, 2]) * s
y = (R[0, 2] - R[2, 0]) * s
z = (R[1, 0] - R[0, 1]) * s
else:
if R[0, 0] > R[1, 1] and R[0, 0] > R[2, 2]:
s = 2.0 * np.sqrt(1.0 + R[0, 0] - R[1, 1] - R[2, 2])
w = (R[2, 1] - R[1, 2]) / s
x = 0.25 * s
y = (R[0, 1] + R[1, 0]) / s
z = (R[0, 2] + R[2, 0]) / s
elif R[1, 1] > R[2, 2]:
s = 2.0 * np.sqrt(1.0 + R[1, 1] - R[0, 0] - R[2, 2])
w = (R[0, 2] - R[2, 0]) / s
x = (R[0, 1] + R[1, 0]) / s
y = 0.25 * s
z = (R[1, 2] + R[2, 1]) / s
else:
s = 2.0 * np.sqrt(1.0 + R[2, 2] - R[0, 0] - R[1, 1])
w = (R[1, 0] - R[0, 1]) / s
x = (R[0, 2] + R[2, 0]) / s
y = (R[1, 2] + R[2, 1]) / s
z = 0.25 * s
return Quaternion(w, x, y, z)
def euler_to_quaternion(roll, pitch, yaw):
cy = np.cos(yaw * 0.5)
sy = np.sin(yaw * 0.5)
cp = np.cos(pitch * 0.5)
sp = np.sin(pitch * 0.5)
cr = np.cos(roll * 0.5)
sr = np.sin(roll * 0.5)
w = cr * cp * cy + sr * sp * sy
x = sr * cp * cy - cr * sp * sy
y = cr * sp * cy + sr * cp * sy
z = cr * cp * sy - sr * sp * cy
return Quaternion(w, x, y, z)
def axis_angle_to_quaternion(axis, angle):
x, y, z = axis
s = np.sin(angle / 2)
w = np.cos(angle / 2)
return Quaternion(w, x * s, y * s, z * s)
七、示例代码
为了更好地理解上面的内容,以下是一个完整的示例代码,展示了如何使用上述方法进行四元数转换。
if __name__ == "__main__":
q = Quaternion(1, 0, 1, 0)
q = q.normalize()
print("Normalized Quaternion:", q)
R = quaternion_to_rotation_matrix(q)
print("Rotation Matrix:\n", R)
roll, pitch, yaw = quaternion_to_euler(q)
print("Euler Angles:", roll, pitch, yaw)
axis, angle = quaternion_to_axis_angle(q)
print("Axis-Angle:", axis, angle)
q_from_R = rotation_matrix_to_quaternion(R)
print("Quaternion from Rotation Matrix:", q_from_R)
q_from_euler = euler_to_quaternion(roll, pitch, yaw)
print("Quaternion from Euler Angles:", q_from_euler)
q_from_axis_angle = axis_angle_to_quaternion(axis, angle)
print("Quaternion from Axis-Angle:", q_from_axis_angle)
通过这些步骤,我们可以在Python中方便地进行四元数转换,并将其应用于三维空间旋转的各种表示形式。四元数的灵活性和高效性使其成为计算机图形学、机器人学和航空航天等领域中的重要工具。
相关问答FAQs:
如何在Python中实现四元数到欧拉角的转换?
在Python中,您可以使用numpy
库和scipy
库中的Rotation
类来将四元数转换为欧拉角。首先,确保您已经安装了这两个库。然后,您可以按以下步骤进行转换:
import numpy as np
from scipy.spatial.transform import Rotation as R
# 定义四元数
quaternion = [w, x, y, z] # 替换为您的四元数值
r = R.from_quat(quaternion)
# 转换为欧拉角(以弧度为单位)
euler_angles = r.as_euler('xyz')
print(euler_angles)
这里的'xyz'
表示您希望以XYZ顺序获取欧拉角,您可以根据需要更改顺序。
在Python中如何将四元数转换为旋转矩阵?
要将四元数转换为旋转矩阵,可以使用scipy
库中的Rotation
类。在定义四元数后,您可以调用as_matrix()
方法获取旋转矩阵。以下是示例代码:
import numpy as np
from scipy.spatial.transform import Rotation as R
# 定义四元数
quaternion = [w, x, y, z] # 替换为您的四元数值
r = R.from_quat(quaternion)
# 转换为旋转矩阵
rotation_matrix = r.as_matrix()
print(rotation_matrix)
旋转矩阵可以用于各种计算和变换。
四元数转换时,有哪些常见的错误需要避免?
在进行四元数转换时,有几种常见的错误需要注意。首先,确保四元数是单位四元数,即其模长为1。如果四元数不是单位四元数,转换结果可能不准确。其次,注意四元数的顺序,通常使用的是[w, x, y, z]格式,但在某些库中可能会使用不同的顺序。确保您使用的库与您提供的四元数格式一致,以避免误解。
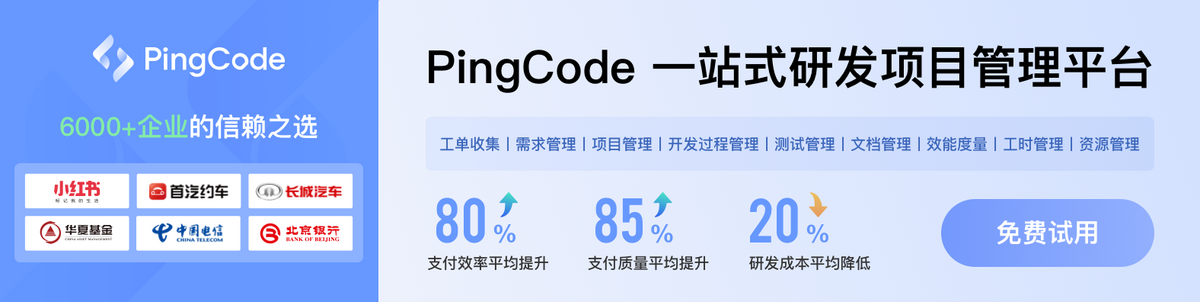