如何自定义路径文件名字python
要自定义路径文件名字python,你可以使用os模块、pathlib模块、字符串操作等方法,以下将详细介绍os模块的使用。
os模块是Python的标准库模块之一,它提供了非常丰富的方法来处理文件和目录。通过os模块,你可以方便地创建、删除、移动和命名文件和目录。以下是详细的介绍:
一、OS模块的使用
1. 导入os模块
要使用os模块,首先需要导入它:
import os
2. 获取当前工作目录
通过os.getcwd()函数可以获取当前的工作目录:
current_directory = os.getcwd()
print("Current Directory:", current_directory)
3. 创建新的目录
通过os.mkdir()函数可以创建新的目录:
new_directory = "new_folder"
os.mkdir(new_directory)
print("Directory created:", new_directory)
4. 检查目录是否存在
通过os.path.exists()函数可以检查目录是否存在:
directory = "new_folder"
if os.path.exists(directory):
print("Directory exists:", directory)
else:
print("Directory does not exist:", directory)
5. 更改文件名
通过os.rename()函数可以更改文件名:
old_name = "old_file.txt"
new_name = "new_file.txt"
os.rename(old_name, new_name)
print("File renamed from", old_name, "to", new_name)
二、PATHLIB模块的使用
1. 导入pathlib模块
要使用pathlib模块,首先需要导入它:
from pathlib import Path
2. 创建路径对象
通过Path类可以创建路径对象:
path = Path("new_folder")
print("Path:", path)
3. 创建新的目录
通过Path.mkdir()方法可以创建新的目录:
path.mkdir()
print("Directory created:", path)
4. 检查目录是否存在
通过Path.exists()方法可以检查目录是否存在:
if path.exists():
print("Directory exists:", path)
else:
print("Directory does not exist:", path)
5. 更改文件名
通过Path.rename()方法可以更改文件名:
old_path = Path("old_file.txt")
new_path = Path("new_file.txt")
old_path.rename(new_path)
print("File renamed from", old_path, "to", new_path)
三、字符串操作
1. 拼接路径
通过字符串操作可以拼接路径:
directory = "new_folder"
file_name = "new_file.txt"
path = directory + "/" + file_name
print("Path:", path)
2. 替换路径中的部分
通过字符串操作可以替换路径中的部分:
path = "old_folder/old_file.txt"
new_path = path.replace("old_folder", "new_folder")
print("New Path:", new_path)
3. 拆分路径
通过字符串操作可以拆分路径:
path = "new_folder/new_file.txt"
directory, file_name = path.rsplit("/", 1)
print("Directory:", directory)
print("File Name:", file_name)
四、实例演示
以下是一个完整的实例演示,包含创建目录、检查目录、创建文件、重命名文件等操作:
import os
from pathlib import Path
创建新的目录
directory = "example_folder"
if not os.path.exists(directory):
os.mkdir(directory)
print("Directory created:", directory)
else:
print("Directory already exists:", directory)
创建新的文件
file_path = os.path.join(directory, "example_file.txt")
with open(file_path, "w") as file:
file.write("Hello, World!")
print("File created:", file_path)
更改文件名
new_file_path = os.path.join(directory, "new_example_file.txt")
os.rename(file_path, new_file_path)
print("File renamed from", file_path, "to", new_file_path)
使用pathlib模块创建新的目录
path = Path("example_folder_2")
if not path.exists():
path.mkdir()
print("Directory created:", path)
else:
print("Directory already exists:", path)
使用pathlib模块创建新的文件
file_path = path / "example_file_2.txt"
with file_path.open("w") as file:
file.write("Hello, Python!")
print("File created:", file_path)
使用pathlib模块更改文件名
new_file_path = path / "new_example_file_2.txt"
file_path.rename(new_file_path)
print("File renamed from", file_path, "to", new_file_path)
五、总结
通过os模块、pathlib模块和字符串操作,你可以方便地自定义路径和文件名字。os模块提供了非常丰富的方法来处理文件和目录,pathlib模块提供了面向对象的路径处理方法,字符串操作则提供了灵活的路径操作能力。掌握这些方法可以帮助你在Python编程中更方便地处理文件和目录。
相关问答FAQs:
如何在Python中设置自定义文件路径和文件名?
在Python中,可以使用os
模块和pathlib
库来设置自定义的文件路径和文件名。使用os.path.join()
可以方便地组合路径,而pathlib.Path
则提供了面向对象的方法来处理文件路径。例如,你可以这样创建一个自定义路径和文件名:
import os
directory = 'my_directory'
filename = 'my_file.txt'
path = os.path.join(directory, filename)
# 或者使用pathlib
from pathlib import Path
path = Path('my_directory') / 'my_file.txt'
在Python中如何确保文件路径的有效性?
确保文件路径有效性可以通过os.path.exists()
和os.makedirs()
函数来实现。os.path.exists()
可以检查路径是否存在,而os.makedirs()
则可以在路径不存在时创建所需的目录。例如:
if not os.path.exists(directory):
os.makedirs(directory)
这样可以避免在写入文件时遇到路径不存在的错误。
如何在Python中处理文件名中的特殊字符?
在Python中处理文件名中的特殊字符时,最好避免使用这些字符,因为它们可能会导致文件系统错误。可使用字符串的replace()
方法来替换或去除特殊字符。例如:
safe_filename = filename.replace(' ', '_').replace(':', '-')
这样可以确保生成的文件名在大多数操作系统中都是有效的。
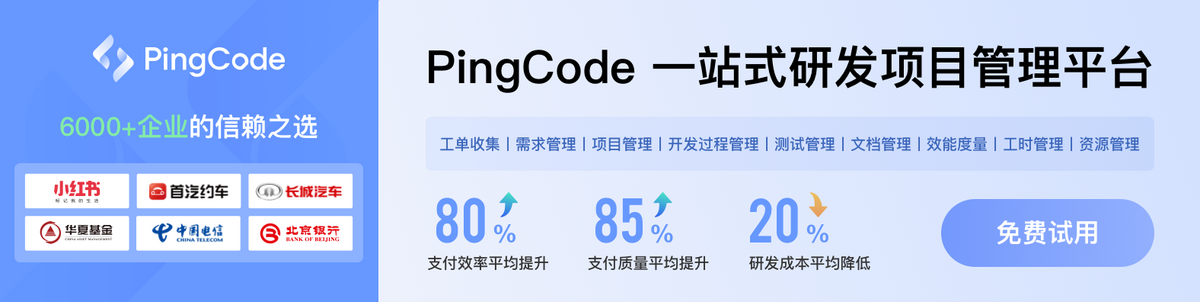