实现全网爬取美女图,可以通过以下几个步骤:选择合适的爬虫框架、解析网页内容、处理图片存储、多线程或分布式爬取、遵守法律法规和网站爬取协议。 在这几个核心步骤中,选择合适的爬虫框架是最重要的一步。选择合适的爬虫框架可以大大提高爬虫的效率和稳定性,下面将详细介绍如何选择和使用爬虫框架。
一、选择合适的爬虫框架
Python中有很多优秀的爬虫框架,如Scrapy、BeautifulSoup、Requests、Selenium等。每个框架都有其独特的优点和适用场景。
1、Scrapy
Scrapy是一个非常强大的爬虫框架,适用于复杂的爬取任务。它提供了丰富的功能,支持异步处理,能够高效地爬取大量数据。
import scrapy
class BeautySpider(scrapy.Spider):
name = "beauty"
start_urls = ['http://example.com']
def parse(self, response):
for image_url in response.css('img::attr(src)').getall():
yield {'image_url': image_url}
2、BeautifulSoup和Requests
BeautifulSoup和Requests是两个常用的库,适用于简单的爬取任务。BeautifulSoup用于解析HTML文档,Requests用于发送HTTP请求。
import requests
from bs4 import BeautifulSoup
response = requests.get('http://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
for img in soup.find_all('img'):
print(img['src'])
二、解析网页内容
解析网页内容是爬虫的核心任务之一。通过分析网页的HTML结构,提取出我们需要的数据。常用的解析方法包括CSS选择器、XPath等。
1、使用CSS选择器
CSS选择器是一种简单而强大的解析方法,适用于大多数网页。
for image_url in response.css('img::attr(src)').getall():
yield {'image_url': image_url}
2、使用XPath
XPath是一种强大的解析方法,适用于复杂的网页结构。
for image_url in response.xpath('//img/@src').getall():
yield {'image_url': image_url}
三、处理图片存储
爬取到图片的URL之后,需要将图片下载并存储到本地。可以使用Requests库来下载图片,并使用Python的文件操作来保存图片。
import requests
def download_image(image_url, file_path):
response = requests.get(image_url)
with open(file_path, 'wb') as file:
file.write(response.content)
四、多线程或分布式爬取
为了提高爬取效率,可以使用多线程或分布式爬取。Python的threading
库可以实现多线程爬取,而Scrapy-Redis可以实现分布式爬取。
1、多线程爬取
多线程爬取可以显著提高爬取速度,适用于中小规模的爬取任务。
import threading
def crawl(image_url):
download_image(image_url, 'images/' + image_url.split('/')[-1])
threads = []
for image_url in image_urls:
thread = threading.Thread(target=crawl, args=(image_url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、分布式爬取
分布式爬取适用于大规模的爬取任务,能够充分利用多台机器的计算资源。
# 使用Scrapy-Redis实现分布式爬取
from scrapy_redis.spiders import RedisSpider
class DistributedBeautySpider(RedisSpider):
name = 'distributed_beauty'
redis_key = 'beauty:start_urls'
def parse(self, response):
for image_url in response.css('img::attr(src)').getall():
yield {'image_url': image_url}
五、遵守法律法规和网站爬取协议
在进行爬取操作时,必须遵守相关法律法规和网站的爬取协议(如robots.txt文件)。这不仅是为了保护网站的合法权益,也是为了防止自己的爬虫被封禁。
1、查看robots.txt文件
通过查看网站的robots.txt文件,可以了解哪些页面允许爬取,哪些页面禁止爬取。
import requests
response = requests.get('http://example.com/robots.txt')
print(response.text)
2、设置爬虫的请求头和延时
为了避免对网站造成过大压力,可以设置爬虫的请求头和延时。
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get('http://example.com', headers=headers)
time.sleep(1) # 延时1秒
六、示例代码
综合以上步骤,我们可以编写一个完整的爬虫示例代码,实现全网爬取美女图。
import requests
from bs4 import BeautifulSoup
import threading
import os
import time
创建存储图片的文件夹
if not os.path.exists('images'):
os.makedirs('images')
def download_image(image_url, file_path):
try:
response = requests.get(image_url, timeout=10)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
except Exception as e:
print(f"Failed to download {image_url}: {e}")
def crawl(url):
try:
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers, timeout=10)
response.raise_for_status()
soup = BeautifulSoup(response.text, 'html.parser')
for img in soup.find_all('img'):
image_url = img['src']
if not image_url.startswith('http'):
continue
file_path = os.path.join('images', image_url.split('/')[-1])
download_image(image_url, file_path)
time.sleep(1) # 延时1秒
except Exception as e:
print(f"Failed to crawl {url}: {e}")
需要爬取的网页列表
urls = ['http://example.com/page1', 'http://example.com/page2']
threads = []
for url in urls:
thread = threading.Thread(target=crawl, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("Crawling completed.")
总结
通过选择合适的爬虫框架、解析网页内容、处理图片存储、多线程或分布式爬取以及遵守法律法规和网站爬取协议,可以实现全网爬取美女图的任务。以上介绍的步骤和示例代码可以帮助你快速入门并实现自己的爬虫项目。在实际应用中,可能还需要根据具体情况进行优化和调整。
相关问答FAQs:
如何选择合适的库进行图片爬取?
在进行全网爬取美女图时,选择合适的库至关重要。Python中有多个库可以帮助你完成这个任务,比如Requests
用于发送HTTP请求,BeautifulSoup
用于解析HTML文档,Scrapy
是一个功能强大的爬虫框架,适合大规模数据抓取。根据项目的复杂度和需求选择合适的工具,可以提高开发效率和爬取成功率。
在爬取美女图时,如何处理反爬虫机制?
许多网站会设置反爬虫机制来防止数据被大量抓取。为了有效对抗这些机制,可以使用随机用户代理(User-Agent)来伪装成浏览器访问,同时可以设置请求间隔,避免频繁访问同一页面。此外,使用代理IP也是一种有效的方法,可以降低被封禁的风险。
如何确保爬取的图片遵循版权法规?
在爬取美女图时,遵循版权法规非常重要。建议在爬取之前,查看目标网站的使用条款,确保图片的使用是合法的。如果打算将爬取的图片用于商业用途,最好获取图片的授权,或者选择使用那些标明为可自由使用的图片资源,如Creative Commons授权的图片。这样不仅能保护自己的合法权益,也能尊重原作者的创作劳动。
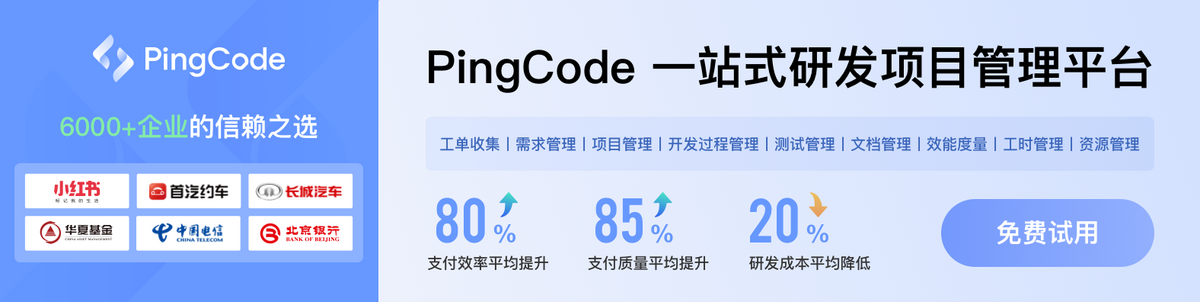