Python 获取进程中间运行的结果有几种不同的方式,常用的方法包括使用多线程、多进程以及异步I/O,其中一种方式是使用 multiprocessing
模块和 Queue
来获取进程中间的运行结果。通过在子进程中定期将结果发送到队列中,主进程可以从队列中读取这些中间结果。以下是一些方法和详细描述:
一、使用多进程模块 multiprocessing
Python 的 multiprocessing
模块允许你创建和管理多个进程。可以使用 Queue
来在主进程和子进程之间传递数据。
1. 创建和管理进程
multiprocessing
模块提供了一个简单的接口来创建和管理进程。通过使用 Process
对象,可以轻松启动和停止进程。
import multiprocessing
def worker(queue):
for i in range(5):
queue.put(f"Result {i}")
time.sleep(1)
if __name__ == "__main__":
queue = multiprocessing.Queue()
process = multiprocessing.Process(target=worker, args=(queue,))
process.start()
while process.is_alive() or not queue.empty():
while not queue.empty():
result = queue.get()
print(f"Received: {result}")
time.sleep(0.5)
process.join()
在上面的示例中,主进程启动一个子进程,并使用 Queue
来传递中间结果。主进程从队列中读取并打印这些结果。
2. 使用 Pipe
进行进程间通信
multiprocessing
模块还提供了 Pipe
对象,用于在两个进程之间进行通信。
import multiprocessing
def worker(conn):
for i in range(5):
conn.send(f"Result {i}")
time.sleep(1)
conn.close()
if __name__ == "__main__":
parent_conn, child_conn = multiprocessing.Pipe()
process = multiprocessing.Process(target=worker, args=(child_conn,))
process.start()
while process.is_alive() or parent_conn.poll():
if parent_conn.poll():
result = parent_conn.recv()
print(f"Received: {result}")
time.sleep(0.5)
process.join()
在这个示例中,使用 Pipe
进行进程间通信。Pipe
提供了 send
和 recv
方法,用于在两个进程之间发送和接收数据。
二、使用线程和队列
Python 的 threading
模块允许你创建和管理多个线程。可以使用 queue
模块来在主线程和工作线程之间传递数据。
1. 使用 queue.Queue
queue.Queue
是一个线程安全的队列,可以在多个线程之间传递数据。
import threading
import queue
import time
def worker(q):
for i in range(5):
q.put(f"Result {i}")
time.sleep(1)
if __name__ == "__main__":
q = queue.Queue()
thread = threading.Thread(target=worker, args=(q,))
thread.start()
while thread.is_alive() or not q.empty():
while not q.empty():
result = q.get()
print(f"Received: {result}")
time.sleep(0.5)
thread.join()
在这个示例中,主线程启动一个工作线程,并使用 queue.Queue
来传递中间结果。主线程从队列中读取并打印这些结果。
三、使用异步 I/O
Python 的 asyncio
模块允许你编写异步代码,以便在等待 I/O 操作时不阻塞其他操作。可以使用 asyncio.Queue
来在协程之间传递数据。
1. 使用 asyncio.Queue
asyncio.Queue
是一个异步队列,可以在多个协程之间传递数据。
import asyncio
async def worker(queue):
for i in range(5):
await queue.put(f"Result {i}")
await asyncio.sleep(1)
async def main():
queue = asyncio.Queue()
task = asyncio.create_task(worker(queue))
while not task.done() or not queue.empty():
while not queue.empty():
result = await queue.get()
print(f"Received: {result}")
await asyncio.sleep(0.5)
await task
asyncio.run(main())
在这个示例中,使用 asyncio
编写异步代码。asyncio.Queue
用于在协程之间传递数据,主协程从队列中读取并打印这些结果。
四、总结
通过以上几种方法,您可以在 Python 中获取进程中间运行的结果:
- 使用
multiprocessing
模块和Queue
对象进行进程间通信。 - 使用
multiprocessing
模块和Pipe
对象进行进程间通信。 - 使用
threading
模块和queue.Queue
对象进行线程间通信。 - 使用
asyncio
模块和asyncio.Queue
对象进行异步协程间通信。
选择哪种方法取决于具体的应用场景和需求。对于 CPU 密集型任务,建议使用多进程;对于 I/O 密集型任务,建议使用多线程或异步 I/O。通过合理使用这些工具,可以实现高效的并发编程。
相关问答FAQs:
如何在Python中捕获进程的实时输出?
在Python中,可以使用subprocess
模块来启动子进程并实时捕获其输出。通过设置stdout
参数为subprocess.PIPE
,可以将子进程的标准输出连接到主进程,从而读取其运行结果。例如,使用for line in process.stdout
可以逐行读取输出,实现实时监控。
是否可以在Python中将进程的输出保存到文件中?
是的,可以将子进程的输出重定向到文件。在使用subprocess.Popen
时,可以指定stdout
参数为一个打开的文件对象,这样进程的输出将自动写入该文件。例如,使用with open('output.txt', 'w') as f:
可以方便地保存进程输出。
如何处理Python进程中的错误信息?
在Python中,可以通过subprocess
模块捕获错误信息。可以将stderr
参数设置为subprocess.PIPE
,这样可以获取到子进程的错误输出。通过读取process.stderr
,可以实时监控和处理进程中的错误情况,确保程序的健壮性。
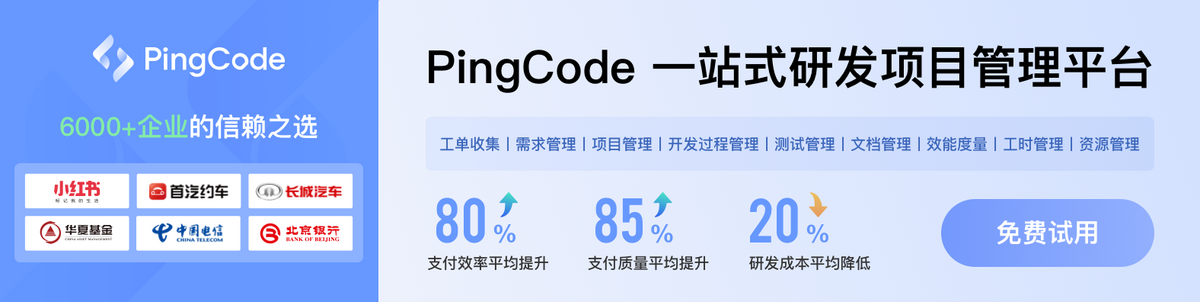