在Python中计算球的表面积的过程可以通过使用数学公式和Python编程语言来实现。本文将详细介绍如何使用Python编写代码来计算球的表面积,并通过多个例子和不同的方法来帮助你更好地理解这一过程。使用数学公式、使用函数、使用面向对象编程是几种常见的方法。下面将对使用数学公式的方法进行详细描述。
一、使用数学公式计算球的表面积
球的表面积计算公式为:[ A = 4 \pi r^2 ]
其中,A表示球的表面积,r表示球的半径,π(pi)是一个常数,约为3.14159。我们可以利用Python的math模块中的pi常量来进行计算。
1. 导入必要的模块
首先,我们需要导入Python的math模块,该模块包含了许多数学函数和常量,包括pi。
import math
2. 编写计算球表面积的函数
接下来,我们可以编写一个函数来计算球的表面积。函数将接受一个参数——球的半径,并返回计算出的表面积。
def sphere_surface_area(radius):
return 4 * math.pi * radius 2
3. 使用函数计算表面积
最后,我们可以调用这个函数,并传入球的半径来计算表面积。例如:
radius = 5
surface_area = sphere_surface_area(radius)
print(f"The surface area of the sphere with radius {radius} is {surface_area:.2f}")
这个示例代码将计算半径为5的球的表面积,并输出结果。
二、使用函数封装计算逻辑
为了提高代码的可读性和可维护性,我们可以将计算球表面积的逻辑封装到一个函数中。这样可以方便地在不同的地方调用这个函数,而不需要重复编写相同的计算逻辑。
1. 定义函数
我们可以定义一个函数calculate_sphere_surface_area
,它接受一个参数radius
,并返回计算出的表面积。
def calculate_sphere_surface_area(radius):
return 4 * math.pi * radius 2
2. 调用函数
在需要计算球表面积的地方,我们可以直接调用这个函数。例如:
radius = 7
surface_area = calculate_sphere_surface_area(radius)
print(f"The surface area of the sphere with radius {radius} is {surface_area:.2f}")
通过这种方式,我们可以方便地计算不同半径的球的表面积,而不需要重复编写计算逻辑。
三、使用面向对象编程计算球的表面积
除了使用函数计算球的表面积之外,我们还可以使用面向对象编程(OOP)的方式来实现这一功能。这种方法可以更好地组织代码,并使其更具扩展性和可维护性。
1. 定义类
我们可以定义一个Sphere
类,包含一个计算表面积的方法。
class Sphere:
def __init__(self, radius):
self.radius = radius
def surface_area(self):
return 4 * math.pi * self.radius 2
2. 创建对象并计算表面积
接下来,我们可以创建一个Sphere
对象,并调用其surface_area
方法来计算表面积。
sphere = Sphere(10)
surface_area = sphere.surface_area()
print(f"The surface area of the sphere with radius {sphere.radius} is {surface_area:.2f}")
通过这种方式,我们可以将球的属性和方法封装到一个类中,从而使代码更加模块化和易于维护。
四、扩展:计算球的体积
除了计算球的表面积之外,我们还可以使用类似的方法来计算球的体积。球的体积计算公式为:[ V = \frac{4}{3} \pi r^3 ]
1. 在函数中计算体积
我们可以在前面定义的函数中添加计算体积的功能。
def calculate_sphere_properties(radius):
surface_area = 4 * math.pi * radius 2
volume = (4/3) * math.pi * radius 3
return surface_area, volume
2. 调用函数
我们可以调用这个函数,并同时获取球的表面积和体积。
radius = 5
surface_area, volume = calculate_sphere_properties(radius)
print(f"The surface area of the sphere with radius {radius} is {surface_area:.2f}")
print(f"The volume of the sphere with radius {radius} is {volume:.2f}")
3. 在类中计算体积
同样地,我们可以在Sphere
类中添加计算体积的方法。
class Sphere:
def __init__(self, radius):
self.radius = radius
def surface_area(self):
return 4 * math.pi * self.radius 2
def volume(self):
return (4/3) * math.pi * self.radius 3
我们可以创建一个Sphere
对象,并分别调用其surface_area
和volume
方法。
sphere = Sphere(10)
surface_area = sphere.surface_area()
volume = sphere.volume()
print(f"The surface area of the sphere with radius {sphere.radius} is {surface_area:.2f}")
print(f"The volume of the sphere with radius {sphere.radius} is {volume:.2f}")
五、结合用户输入
为了使程序更加实用,我们可以结合用户输入来计算球的表面积和体积。这样,用户可以输入不同的半径值,并获取相应的计算结果。
1. 获取用户输入
我们可以使用input
函数来获取用户输入的半径值。
radius = float(input("Enter the radius of the sphere: "))
2. 调用函数计算表面积和体积
我们可以使用前面定义的函数来计算表面积和体积,并输出结果。
surface_area, volume = calculate_sphere_properties(radius)
print(f"The surface area of the sphere with radius {radius} is {surface_area:.2f}")
print(f"The volume of the sphere with radius {radius} is {volume:.2f}")
3. 在类中使用用户输入
同样地,我们可以在创建Sphere
对象时使用用户输入的半径值。
radius = float(input("Enter the radius of the sphere: "))
sphere = Sphere(radius)
surface_area = sphere.surface_area()
volume = sphere.volume()
print(f"The surface area of the sphere with radius {sphere.radius} is {surface_area:.2f}")
print(f"The volume of the sphere with radius {sphere.radius} is {volume:.2f}")
六、处理异常情况
在实际应用中,用户输入的半径值可能会包含无效数据(如负数或非数字字符)。我们需要处理这些异常情况,以确保程序的健壮性和稳定性。
1. 使用try-except块处理异常
我们可以使用try-except
块来捕获和处理异常情况。例如,当用户输入非数字字符时,程序将捕获ValueError
异常,并提示用户重新输入。
while True:
try:
radius = float(input("Enter the radius of the sphere: "))
if radius < 0:
raise ValueError("The radius must be a positive number.")
break
except ValueError as e:
print(f"Invalid input: {e}. Please try again.")
2. 结合异常处理进行计算
在捕获到有效的半径值后,我们可以继续进行表面积和体积的计算。
surface_area, volume = calculate_sphere_properties(radius)
print(f"The surface area of the sphere with radius {radius} is {surface_area:.2f}")
print(f"The volume of the sphere with radius {radius} is {volume:.2f}")
通过这种方式,我们可以确保程序在处理用户输入时更加健壮,并能够应对各种异常情况。
七、总结
本文详细介绍了如何使用Python计算球的表面积,并通过多个示例展示了不同的方法,包括使用数学公式、函数封装、面向对象编程以及结合用户输入和异常处理。通过这些方法,我们可以编写出更加模块化、可维护和健壮的代码,从而在实际应用中更加高效地解决问题。希望通过本文的学习,你能够掌握使用Python计算球的表面积的技巧,并将其应用到实际项目中。
相关问答FAQs:
1. 用Python计算球的表面积需要哪些数学公式?
计算球的表面积需要使用球的表面积公式,即 ( S = 4 \pi r^2 ),其中 ( S ) 表示表面积,( r ) 是球的半径,( \pi ) 是圆周率。可以使用Python中的math库来获取π的值,便于计算。
2. 如何在Python中实现计算球的表面积的代码?
可以通过定义一个函数来实现球的表面积计算。以下是一个示例代码:
import math
def calculate_sphere_surface_area(radius):
return 4 * math.pi * radius**2
# 示例:计算半径为5的球的表面积
radius = 5
surface_area = calculate_sphere_surface_area(radius)
print(f"半径为{radius}的球的表面积是:{surface_area}")
这个代码片段将根据输入的半径计算并输出球的表面积。
3. 如果我只知道球的体积,如何通过体积计算表面积?
如果已知球的体积 ( V ) 可以使用公式 ( V = \frac{4}{3} \pi r^3 ) 来计算半径 ( r )。一旦获得半径,可以使用表面积公式 ( S = 4 \pi r^2 ) 进行计算。以下是实现这个过程的Python示例代码:
def calculate_radius_from_volume(volume):
return ((3 * volume) / (4 * math.pi))**(1/3)
def calculate_surface_area_from_volume(volume):
radius = calculate_radius_from_volume(volume)
return 4 * math.pi * radius**2
# 示例:已知体积为100的球
volume = 100
surface_area = calculate_surface_area_from_volume(volume)
print(f"体积为{volume}的球的表面积是:{surface_area}")
这个代码段将帮助用户根据已知体积计算球的表面积。
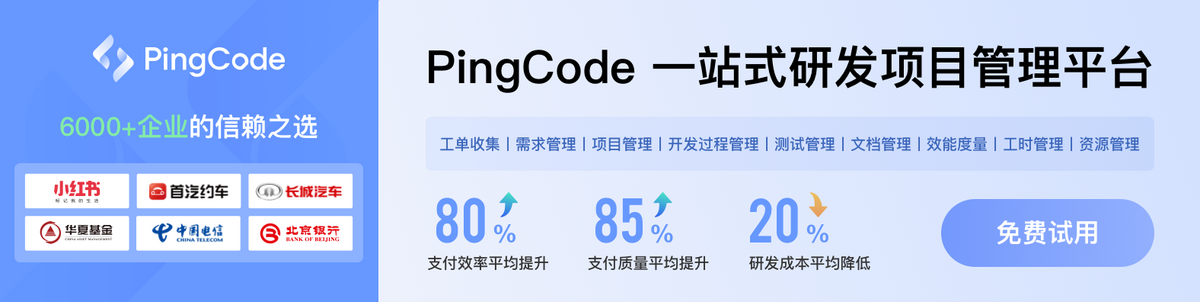