开头段落:
Python查找文本中的高频词汇可以通过以下方法实现:使用collections.Counter模块、使用NLTK库、使用re正则表达式模块。其中,使用collections.Counter模块是一种高效且简洁的方法。Counter模块是Python标准库中的一部分,它可以帮助我们轻松地统计文本中每个单词的出现次数,并找出高频词汇。下面我们将详细介绍这些方法的实现步骤及相关代码示例,以便更好地理解和使用这些工具来分析文本中的高频词汇。
一、使用collections.Counter模块
使用collections.Counter模块是查找文本中高频词汇的一种简单且高效的方法。Counter是一个专门用于计数的类,它的主要功能是帮助我们统计元素的出现次数。我们可以使用Counter来统计文本中每个单词的出现次数,然后根据次数进行排序,找到高频词汇。
from collections import Counter
def find_high_freq_words(text, n=10):
# 分割文本为单词列表
words = text.split()
# 使用Counter统计每个单词的出现次数
word_counts = Counter(words)
# 找出出现次数最多的n个单词
high_freq_words = word_counts.most_common(n)
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words = find_high_freq_words(text)
print(high_freq_words)
以上代码示例展示了如何使用Counter模块来统计文本中的高频词汇。首先,我们将文本分割为单词列表,然后使用Counter统计每个单词的出现次数,最后找出出现次数最多的若干个单词。
二、使用NLTK库
NLTK(Natural Language Toolkit)是一个用于处理自然语言文本的库,包含了丰富的文本处理工具和资源。我们可以使用NLTK库来查找文本中的高频词汇。NLTK库提供了分词、词性标注、去除停用词等多种功能,使得文本处理更加方便和精准。
import nltk
from nltk.corpus import stopwords
from collections import Counter
下载NLTK的停用词列表
nltk.download('stopwords')
def find_high_freq_words_nltk(text, n=10):
# 分割文本为单词列表
words = nltk.word_tokenize(text)
# 去除停用词
filtered_words = [word for word in words if word.lower() not in stopwords.words('english')]
# 使用Counter统计每个单词的出现次数
word_counts = Counter(filtered_words)
# 找出出现次数最多的n个单词
high_freq_words = word_counts.most_common(n)
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words_nltk = find_high_freq_words_nltk(text)
print(high_freq_words_nltk)
在以上代码示例中,我们使用NLTK库中的word_tokenize函数将文本分割为单词列表,并使用stopwords模块去除常见的停用词。然后,使用Counter统计每个单词的出现次数,并找出出现次数最多的若干个单词。
三、使用re正则表达式模块
正则表达式(Regular Expressions)是用于匹配字符串的一种工具。Python中的re模块提供了对正则表达式的支持,使得我们可以使用正则表达式对文本进行复杂的模式匹配和处理。我们可以使用re模块来提取文本中的单词,并统计每个单词的出现次数,以找出高频词汇。
import re
from collections import Counter
def find_high_freq_words_re(text, n=10):
# 使用正则表达式提取文本中的单词
words = re.findall(r'\b\w+\b', text)
# 使用Counter统计每个单词的出现次数
word_counts = Counter(words)
# 找出出现次数最多的n个单词
high_freq_words = word_counts.most_common(n)
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words_re = find_high_freq_words_re(text)
print(high_freq_words_re)
在以上代码示例中,我们使用re模块的findall函数提取文本中的单词,并使用Counter统计每个单词的出现次数。然后,根据次数找出出现次数最多的若干个单词。
四、使用Pandas库
Pandas是一个强大的数据处理和分析库,主要用于结构化数据的处理。我们可以使用Pandas库来处理文本数据,并统计文本中的高频词汇。Pandas库提供了丰富的数据操作方法,使得数据处理更加灵活和高效。
import pandas as pd
from collections import Counter
def find_high_freq_words_pandas(text, n=10):
# 分割文本为单词列表
words = text.split()
# 创建一个DataFrame
df = pd.DataFrame(words, columns=['word'])
# 统计每个单词的出现次数
word_counts = df['word'].value_counts()
# 找出出现次数最多的n个单词
high_freq_words = word_counts.head(n)
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words_pandas = find_high_freq_words_pandas(text)
print(high_freq_words_pandas)
在以上代码示例中,我们使用Pandas库创建一个DataFrame,并使用value_counts方法统计每个单词的出现次数。然后,找出出现次数最多的若干个单词。
五、使用Scikit-learn库
Scikit-learn是一个用于机器学习的Python库,包含了丰富的机器学习算法和工具。我们可以使用Scikit-learn库中的CountVectorizer来统计文本中的词频,并找出高频词汇。CountVectorizer是一个用于将文本转换为词频矩阵的工具,非常适合用于文本分析和自然语言处理。
from sklearn.feature_extraction.text import CountVectorizer
def find_high_freq_words_sklearn(text, n=10):
# 创建CountVectorizer对象
vectorizer = CountVectorizer()
# 将文本转换为词频矩阵
X = vectorizer.fit_transform([text])
# 获取词汇表
vocab = vectorizer.get_feature_names_out()
# 获取词频
word_counts = X.toarray().flatten()
# 创建词汇和词频的字典
word_freq_dict = dict(zip(vocab, word_counts))
# 按词频排序并找出高频词汇
high_freq_words = sorted(word_freq_dict.items(), key=lambda x: x[1], reverse=True)[:n]
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words_sklearn = find_high_freq_words_sklearn(text)
print(high_freq_words_sklearn)
在以上代码示例中,我们使用Scikit-learn库中的CountVectorizer将文本转换为词频矩阵,并获取词汇表和词频。然后,我们创建一个词汇和词频的字典,并按词频排序,找出高频词汇。
六、使用Gensim库
Gensim是一个用于主题建模和文档相似度计算的Python库,适用于大规模文本处理。我们可以使用Gensim库来统计文本中的词频,并找出高频词汇。Gensim库提供了丰富的文本处理工具,使得文本分析更加高效和准确。
from gensim import corpora
def find_high_freq_words_gensim(text, n=10):
# 分割文本为单词列表
words = text.split()
# 创建词汇表
dictionary = corpora.Dictionary([words])
# 统计每个单词的出现次数
word_counts = dictionary.dfs
# 按词频排序并找出高频词汇
high_freq_words = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)[:n]
# 获取词汇和词频
high_freq_words = [(dictionary[word_id], freq) for word_id, freq in high_freq_words]
return high_freq_words
示例文本
text = "Python is a powerful programming language. Python is used for web development, data analysis, artificial intelligence, and scientific computing. Python is popular among developers."
找出高频词汇
high_freq_words_gensim = find_high_freq_words_gensim(text)
print(high_freq_words_gensim)
在以上代码示例中,我们使用Gensim库创建词汇表,并统计每个单词的出现次数。然后,按词频排序,找出高频词汇,并获取对应的词汇和词频。
总结:
本文介绍了Python查找文本中高频词汇的多种方法,包括使用collections.Counter模块、使用NLTK库、使用re正则表达式模块、使用Pandas库、使用Scikit-learn库、使用Gensim库等。这些方法各有优劣,用户可以根据具体需求选择合适的方法来统计文本中的高频词汇。希望本文对大家在文本分析和自然语言处理方面有所帮助。
相关问答FAQs:
在Python中,有哪些库可以帮助我找到文本中的高频词汇?
Python提供了多个强大的库来处理文本和找到高频词汇。最常用的包括NLTK(自然语言工具包)、spaCy和collections模块。NLTK可以进行文本预处理和词频分析,spaCy则适合处理更复杂的自然语言处理任务,而collections模块中的Counter类非常适合快速统计词频。
如何处理文本以确保更准确的高频词汇分析?
为了确保高频词汇分析的准确性,文本预处理非常重要。建议对文本进行以下步骤:去除标点符号、转换为小写字母、去除停用词(如“的”、“是”、“在”等常见但信息量少的词),以及进行词干提取或词形还原。经过这些处理后,分析结果将更加可靠。
我可以使用哪些方法来可视化高频词汇的结果?
可视化高频词汇的结果有助于更直观地理解数据。常用的可视化方法包括词云图、条形图和饼图。可以使用WordCloud库生成词云,利用Matplotlib或Seaborn库创建条形图或饼图。这些可视化工具能够帮助用户更轻松地识别文本中的主要主题和关键字。
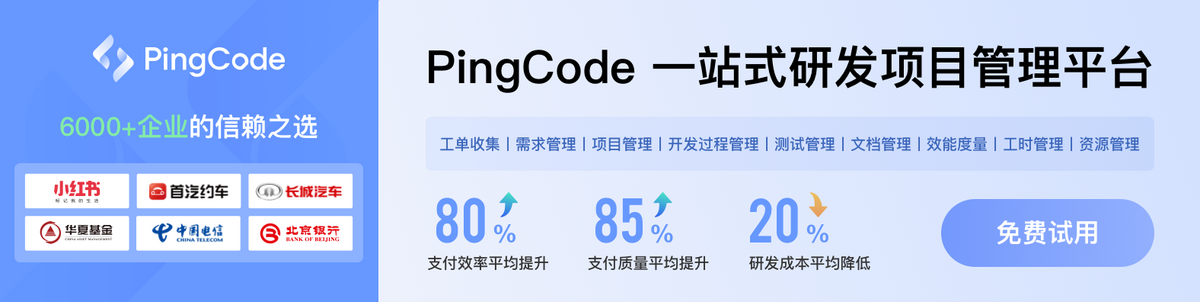