Python可以与PHP服务器端进行交互的主要方法包括:使用HTTP请求、通过命令行执行PHP脚本、以及通过Web服务进行API调用。 其中,最常用且高效的方法是通过HTTP请求,这种方式允许Python应用程序与PHP服务器端通信,发送和接收数据。下面将详细介绍如何实现这些交互方法。
一、HTTP请求
通过HTTP请求,Python可以向PHP服务器发送请求,并接收服务器的响应数据。这种方法通常用于Web应用程序和API集成。
1、使用requests库
requests
库是Python中最常用的HTTP库,可以轻松发送HTTP请求和处理响应。
import requests
url = 'http://your-php-server.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=data)
if response.status_code == 200:
print('Success:', response.json())
else:
print('Failed:', response.status_code)
在PHP服务器端,您可以使用以下代码处理POST请求:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$key1 = $_POST['key1'];
$key2 = $_POST['key2'];
// 处理数据并返回响应
$response = array('status' => 'success', 'data' => array($key1, $key2));
echo json_encode($response);
}
?>
2、GET请求
requests
库也可以轻松发送GET请求,适用于需要从PHP服务器获取数据的场景。
import requests
url = 'http://your-php-server.com/api?param1=value1¶m2=value2'
response = requests.get(url)
if response.status_code == 200:
print('Success:', response.json())
else:
print('Failed:', response.status_code)
在PHP服务器端,您可以使用以下代码处理GET请求:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'GET') {
$param1 = $_GET['param1'];
$param2 = $_GET['param2'];
// 处理数据并返回响应
$response = array('status' => 'success', 'data' => array($param1, $param2));
echo json_encode($response);
}
?>
二、命令行执行PHP脚本
Python还可以通过命令行执行PHP脚本,并获取脚本的输出结果。这种方法适用于需要在本地或服务器上运行PHP脚本的场景。
1、使用subprocess模块
subprocess
模块可以在Python中创建新的进程,执行命令,并获取命令的输出。
import subprocess
php_script = 'path/to/your_script.php'
result = subprocess.run(['php', php_script], capture_output=True, text=True)
if result.returncode == 0:
print('Success:', result.stdout)
else:
print('Failed:', result.stderr)
2、传递参数
如果需要向PHP脚本传递参数,可以在命令行中添加参数。
import subprocess
php_script = 'path/to/your_script.php'
params = ['param1', 'param2']
result = subprocess.run(['php', php_script] + params, capture_output=True, text=True)
if result.returncode == 0:
print('Success:', result.stdout)
else:
print('Failed:', result.stderr)
在PHP脚本中,您可以使用$argv
数组获取命令行参数:
<?php
if (isset($argv)) {
$param1 = $argv[1];
$param2 = $argv[2];
// 处理参数并输出结果
echo "Parameter 1: $param1\n";
echo "Parameter 2: $param2\n";
}
?>
三、Web服务API调用
通过创建Web服务,您可以让PHP服务器提供API接口,Python通过HTTP请求调用这些接口进行交互。
1、创建PHP API接口
首先,在PHP服务器端创建一个简单的API接口。例如,使用Slim框架创建一个GET接口:
<?php
require 'vendor/autoload.php';
$app = new \Slim\App;
$app->get('/api/data', function ($request, $response, $args) {
$data = array('key1' => 'value1', 'key2' => 'value2');
return $response->withJson($data);
});
$app->run();
?>
2、调用API接口
在Python中,使用requests
库调用PHP API接口,并处理响应数据:
import requests
url = 'http://your-php-server.com/api/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print('Success:', data)
else:
print('Failed:', response.status_code)
四、数据格式转换
为了确保Python和PHP之间的数据传输顺利进行,通常需要对数据进行格式转换。例如,使用JSON格式进行数据传输。
1、Python发送JSON数据
在Python中,可以使用json
模块将数据转换为JSON格式,并通过requests
库发送:
import json
import requests
url = 'http://your-php-server.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
print('Success:', response.json())
else:
print('Failed:', response.status_code)
2、PHP接收JSON数据
在PHP服务器端,可以使用file_get_contents
和json_decode
函数接收和解析JSON数据:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$json_data = file_get_contents('php://input');
$data = json_decode($json_data, true);
$key1 = $data['key1'];
$key2 = $data['key2'];
// 处理数据并返回响应
$response = array('status' => 'success', 'data' => array($key1, $key2));
echo json_encode($response);
}
?>
五、错误处理和调试
在实际开发中,错误处理和调试是确保Python与PHP服务器端交互成功的重要步骤。
1、Python错误处理
在Python中,可以使用try-except
语句捕获异常,并进行适当的处理:
import requests
url = 'http://your-php-server.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
try:
response = requests.post(url, data=data)
response.raise_for_status()
print('Success:', response.json())
except requests.exceptions.RequestException as e:
print('Failed:', e)
2、PHP错误处理
在PHP服务器端,可以使用try-catch
语句捕获异常,并返回适当的错误响应:
<?php
try {
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$json_data = file_get_contents('php://input');
$data = json_decode($json_data, true);
$key1 = $data['key1'];
$key2 = $data['key2'];
// 处理数据并返回响应
$response = array('status' => 'success', 'data' => array($key1, $key2));
echo json_encode($response);
}
} catch (Exception $e) {
$response = array('status' => 'error', 'message' => $e->getMessage());
echo json_encode($response);
}
?>
通过以上详细介绍,您可以掌握Python与PHP服务器端交互的多种方法,并根据实际需求选择合适的实现方式。无论是通过HTTP请求、命令行执行PHP脚本,还是通过Web服务API调用,这些方法都可以帮助您实现Python与PHP服务器端的高效通信。
相关问答FAQs:
如何在Python中与PHP服务器进行交互?
可以通过多种方式实现Python与PHP服务器的交互。例如,您可以使用HTTP请求库(如requests
)在Python中发送GET或POST请求到PHP脚本,获取其返回的数据。确保PHP脚本能够处理请求并返回JSON或其他格式的数据,以便Python可以轻松解析。
在Python中处理PHP返回的数据时需要注意什么?
处理PHP返回的数据时,确保数据格式正确是至关重要的。如果PHP返回JSON数据,可以使用Python的json
模块来解析。务必检查HTTP响应状态码,以确保请求成功,并妥善处理可能的错误。
有哪些常见的安全性考虑在Python与PHP服务器交互时需要关注?
安全性非常重要。在进行请求时,确保使用HTTPS协议以加密数据传输,避免中间人攻击。此外,验证服务器的SSL证书,以确保连接的安全性。同时,避免在请求中直接暴露敏感信息,并对用户输入进行适当的验证和清理,以防止SQL注入或其他攻击。
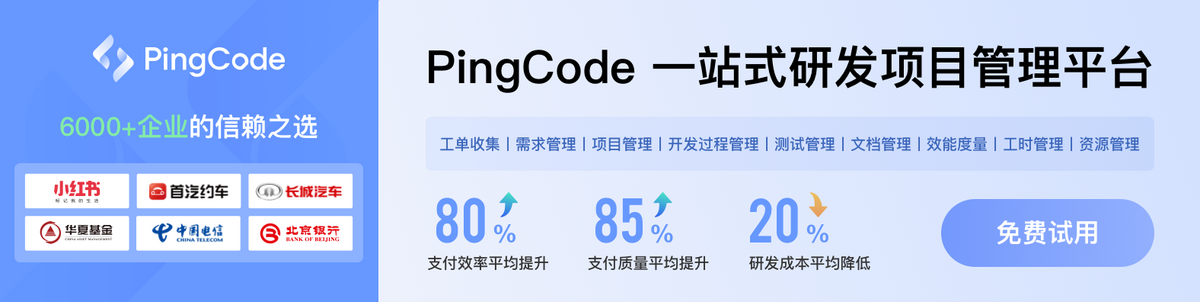