使用__dict__
属性、使用dir()
函数、使用vars()
函数是Python中查看一个类的属性的三种常见方法。__dict__
属性包含类的属性和方法的字典、dir()
函数列出类的所有属性和方法、vars()
函数以字典形式返回类的属性。下面我们将详细描述其中的一种方法。
__dict__
属性是一个类的所有属性和方法的字典。通过访问一个类的__dict__
属性,我们可以查看该类的所有属性和方法,包括私有属性。使用__dict__
属性的方法如下:
class MyClass:
class_attribute = "I am a class attribute"
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def my_method(self):
pass
Create an instance of MyClass
my_instance = MyClass("I am an instance attribute")
Access the __dict__ attribute of the class
print(MyClass.__dict__)
Output: {'__module__': '__main__', 'class_attribute': 'I am a class attribute', '__init__': <function MyClass.__init__ at 0x7f8e0c0b1e50>, 'my_method': <function MyClass.my_method at 0x7f8e0c0b1ee0>, '__dict__': <attribute '__dict__' of 'MyClass' objects>, '__weakref__': <attribute '__weakref__' of 'MyClass' objects>, '__doc__': None}
Access the __dict__ attribute of the instance
print(my_instance.__dict__)
Output: {'instance_attribute': 'I am an instance attribute'}
在以上代码中,我们定义了一个类MyClass
,它包含一个类属性class_attribute
、一个实例属性instance_attribute
和一个方法my_method
。我们通过访问类的__dict__
属性,查看了类的所有属性和方法。我们还通过访问实例的__dict__
属性,查看了实例的所有属性。
一、dir()
函数
dir()
函数返回类的所有属性和方法,包括继承的属性和方法。使用dir()
函数的方法如下:
class MyClass:
class_attribute = "I am a class attribute"
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def my_method(self):
pass
Create an instance of MyClass
my_instance = MyClass("I am an instance attribute")
Use dir() to get the list of attributes and methods of the class
print(dir(MyClass))
Output: ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'class_attribute', 'my_method']
Use dir() to get the list of attributes and methods of the instance
print(dir(my_instance))
Output: ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'class_attribute', 'instance_attribute', 'my_method']
在以上代码中,我们定义了一个类MyClass
,它包含一个类属性class_attribute
、一个实例属性instance_attribute
和一个方法my_method
。我们通过dir()
函数,查看了类和实例的所有属性和方法。
二、vars()
函数
vars()
函数返回类或实例的所有属性和方法的字典。使用vars()
函数的方法如下:
class MyClass:
class_attribute = "I am a class attribute"
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def my_method(self):
pass
Create an instance of MyClass
my_instance = MyClass("I am an instance attribute")
Use vars() to get the dictionary of attributes and methods of the class
print(vars(MyClass))
Output: {'__module__': '__main__', 'class_attribute': 'I am a class attribute', '__init__': <function MyClass.__init__ at 0x7f8e0c0b1e50>, 'my_method': <function MyClass.my_method at 0x7f8e0c0b1ee0>, '__dict__': <attribute '__dict__' of 'MyClass' objects>, '__weakref__': <attribute '__weakref__' of 'MyClass' objects>, '__doc__': None}
Use vars() to get the dictionary of attributes and methods of the instance
print(vars(my_instance))
Output: {'instance_attribute': 'I am an instance attribute'}
在以上代码中,我们定义了一个类MyClass
,它包含一个类属性class_attribute
、一个实例属性instance_attribute
和一个方法my_method
。我们通过vars()
函数,查看了类和实例的所有属性和方法。
三、实例属性和类属性的区别
在Python中,类属性是类级别的属性,所有实例共享同一个类属性。实例属性是实例级别的属性,每个实例都有自己独立的实例属性。我们可以通过__dict__
、dir()
和vars()
函数分别查看类属性和实例属性。
class MyClass:
class_attribute = "I am a class attribute"
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def my_method(self):
pass
Create two instances of MyClass
instance1 = MyClass("I am instance 1")
instance2 = MyClass("I am instance 2")
Modify the class attribute
MyClass.class_attribute = "Modified class attribute"
Modify the instance attribute
instance1.instance_attribute = "Modified instance 1"
Access the __dict__ attribute of the class
print(MyClass.__dict__)
Output: {'__module__': '__main__', 'class_attribute': 'Modified class attribute', '__init__': <function MyClass.__init__ at 0x7f8e0c0b1e50>, 'my_method': <function MyClass.my_method at 0x7f8e0c0b1ee0>, '__dict__': <attribute '__dict__' of 'MyClass' objects>, '__weakref__': <attribute '__weakref__' of 'MyClass' objects>, '__doc__': None}
Access the __dict__ attribute of the instances
print(instance1.__dict__)
Output: {'instance_attribute': 'Modified instance 1'}
print(instance2.__dict__)
Output: {'instance_attribute': 'I am instance 2'}
在以上代码中,我们修改了类属性class_attribute
和实例属性instance_attribute
。我们通过__dict__
属性分别查看了类和实例的属性,发现类属性是共享的,而实例属性是独立的。
四、使用自定义方法查看类属性
我们还可以通过自定义方法来查看类的属性。以下是一个示例:
class MyClass:
class_attribute = "I am a class attribute"
def __init__(self, instance_attribute):
self.instance_attribute = instance_attribute
def my_method(self):
pass
@classmethod
def get_class_attributes(cls):
return {key: value for key, value in cls.__dict__.items() if not key.startswith('__')}
def get_instance_attributes(self):
return self.__dict__
Create an instance of MyClass
my_instance = MyClass("I am an instance attribute")
Use custom methods to get class and instance attributes
print(MyClass.get_class_attributes())
Output: {'class_attribute': 'I am a class attribute', 'my_method': <function MyClass.my_method at 0x7f8e0c0b1e50>, 'get_class_attributes': <bound method MyClass.get_class_attributes of <class '__main__.MyClass'>>}
print(my_instance.get_instance_attributes())
Output: {'instance_attribute': 'I am an instance attribute'}
在以上代码中,我们定义了两个自定义方法get_class_attributes
和get_instance_attributes
,分别用于查看类属性和实例属性。通过这些方法,我们可以更方便地查看类的属性。
五、总结
在本文中,我们介绍了Python中查看一个类的属性的三种常见方法:使用__dict__
属性、使用dir()
函数、使用vars()
函数。我们详细描述了每种方法的使用方法,并介绍了实例属性和类属性的区别。最后,我们展示了如何使用自定义方法来查看类的属性。这些方法和技巧可以帮助我们更好地理解和操作Python中的类和对象。
相关问答FAQs:
如何在Python中查看一个类的所有属性和方法?
要查看一个类的所有属性和方法,可以使用内置的dir()
函数。这个函数会列出类的所有成员,包括属性和方法。此外,使用vars()
函数可以获取类的属性字典,提供更详细的信息。
是否可以查看类的私有属性?
在Python中,私有属性以双下划线开头。虽然这些属性并不是真正私有的,但访问它们需要使用特定的语法。例如,可以通过类名._类名__私有属性名
来访问私有属性。不过,不建议直接访问私有属性,因为这样可能会破坏类的封装性。
如何通过反射机制查看类的属性?
反射机制可以通过getattr()
函数来获取类的属性。通过传入类实例和属性名,能够动态地访问其属性值。这种方法在处理动态属性时非常有用,可以在不知道属性名的情况下获取相应的值。
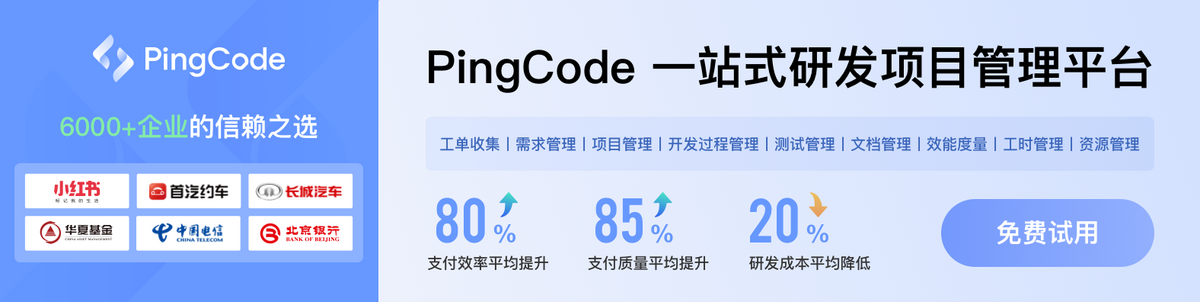