Python中如何输入三角函数
在Python中输入三角函数可以通过使用Python的数学库——math
模块来实现。首先导入math模块、使用函数如sin()
、cos()
、tan()
,并且要注意角度与弧度的转换。 例如,我们可以使用math.radians()
函数来将角度转换为弧度,然后再计算三角函数的值。这里我们详细展开使用 math
模块。
一、导入math模块
在Python中,math
模块提供了许多数学函数,其中包括各种三角函数。在开始使用这些函数之前,你需要先导入math
模块。你可以通过以下简单的代码行来导入math
模块:
import math
导入math
模块后,你就可以使用其中的三角函数了。
二、使用基本三角函数
- 正弦函数(sin)
math.sin()
函数用于计算一个角度的正弦值。需要注意的是,math.sin()
函数接受的参数是弧度而不是角度。如果你有一个角度值,可以使用math.radians()
函数将其转换为弧度。以下是一个示例:
import math
angle_degrees = 30 # 角度
angle_radians = math.radians(angle_degrees) # 将角度转换为弧度
sin_value = math.sin(angle_radians) # 计算正弦值
print(f"Sin({angle_degrees} degrees) = {sin_value}")
- 余弦函数(cos)
math.cos()
函数用于计算一个角度的余弦值。与math.sin()
类似,它也接受弧度作为参数。以下是一个示例:
import math
angle_degrees = 60 # 角度
angle_radians = math.radians(angle_degrees) # 将角度转换为弧度
cos_value = math.cos(angle_radians) # 计算余弦值
print(f"Cos({angle_degrees} degrees) = {cos_value}")
- 正切函数(tan)
math.tan()
函数用于计算一个角度的正切值。以下是一个示例:
import math
angle_degrees = 45 # 角度
angle_radians = math.radians(angle_degrees) # 将角度转换为弧度
tan_value = math.tan(angle_radians) # 计算正切值
print(f"Tan({angle_degrees} degrees) = {tan_value}")
三、使用反三角函数
math
模块还提供了反三角函数,用于从三角函数的值反推出角度。这些函数包括math.asin()
、math.acos()
和math.atan()
。
- 反正弦函数(asin)
math.asin()
函数用于计算给定正弦值的反正弦值,并返回结果的弧度值。以下是一个示例:
import math
sin_value = 0.5
angle_radians = math.asin(sin_value) # 计算反正弦值
angle_degrees = math.degrees(angle_radians) # 将弧度转换为角度
print(f"Asin({sin_value}) = {angle_degrees} degrees")
- 反余弦函数(acos)
math.acos()
函数用于计算给定余弦值的反余弦值。以下是一个示例:
import math
cos_value = 0.5
angle_radians = math.acos(cos_value) # 计算反余弦值
angle_degrees = math.degrees(angle_radians) # 将弧度转换为角度
print(f"Acos({cos_value}) = {angle_degrees} degrees")
- 反正切函数(atan)
math.atan()
函数用于计算给定正切值的反正切值。以下是一个示例:
import math
tan_value = 1
angle_radians = math.atan(tan_value) # 计算反正切值
angle_degrees = math.degrees(angle_radians) # 将弧度转换为角度
print(f"Atan({tan_value}) = {angle_degrees} degrees")
四、处理角度和弧度的转换
在使用三角函数时,通常需要在角度和弧度之间进行转换。math
模块提供了两个非常有用的函数:math.radians()
和math.degrees()
,用于角度和弧度之间的转换。
- 将角度转换为弧度
使用math.radians()
函数可以将角度转换为弧度。以下是一个示例:
import math
angle_degrees = 90
angle_radians = math.radians(angle_degrees) # 将角度转换为弧度
print(f"{angle_degrees} degrees = {angle_radians} radians")
- 将弧度转换为角度
使用math.degrees()
函数可以将弧度转换为角度。以下是一个示例:
import math
angle_radians = math.pi / 2
angle_degrees = math.degrees(angle_radians) # 将弧度转换为角度
print(f"{angle_radians} radians = {angle_degrees} degrees")
五、使用hypot函数计算斜边
在一些几何计算中,你可能需要计算一个直角三角形的斜边长度。math
模块提供了math.hypot()
函数来简化这一计算。math.hypot(x, y)
函数接受两个参数,分别表示直角三角形的两个直角边的长度,并返回斜边的长度。以下是一个示例:
import math
x = 3
y = 4
hypotenuse = math.hypot(x, y) # 计算斜边长度
print(f"The hypotenuse of a right triangle with sides {x} and {y} is {hypotenuse}")
六、使用其他三角函数
除了基本的三角函数外,math
模块还提供了其他一些有用的三角函数:
- math.sinh()、math.cosh()、math.tanh()
这些函数分别用于计算双曲正弦、双曲余弦和双曲正切。以下是一个示例:
import math
x = 1
sinh_value = math.sinh(x) # 计算双曲正弦
cosh_value = math.cosh(x) # 计算双曲余弦
tanh_value = math.tanh(x) # 计算双曲正切
print(f"Sinh({x}) = {sinh_value}")
print(f"Cosh({x}) = {cosh_value}")
print(f"Tanh({x}) = {tanh_value}")
- math.asinh()、math.acosh()、math.atanh()
这些函数分别用于计算给定值的反双曲正弦、反双曲余弦和反双曲正切。以下是一个示例:
import math
x = 1
asinh_value = math.asinh(x) # 计算反双曲正弦
acosh_value = math.acosh(x + 1) # 计算反双曲余弦(注意输入值需要大于等于1)
atanh_value = math.atanh(0.5) # 计算反双曲正切
print(f"Asinh({x}) = {asinh_value}")
print(f"Acosh({x + 1}) = {acosh_value}")
print(f"Atanh(0.5) = {atanh_value}")
七、使用numpy库进行三角函数计算
除了math
模块外,numpy
库也是进行数学计算的强大工具。numpy
库提供了更丰富的函数和更高效的数组操作,非常适合处理大规模的科学计算。以下是一些示例:
- 使用numpy计算正弦函数
import numpy as np
angles_degrees = np.array([0, 30, 45, 60, 90]) # 角度数组
angles_radians = np.radians(angles_degrees) # 将角度数组转换为弧度数组
sin_values = np.sin(angles_radians) # 计算正弦值
print(f"Angles (degrees): {angles_degrees}")
print(f"Sin values: {sin_values}")
- 使用numpy计算余弦函数
import numpy as np
angles_degrees = np.array([0, 30, 45, 60, 90]) # 角度数组
angles_radians = np.radians(angles_degrees) # 将角度数组转换为弧度数组
cos_values = np.cos(angles_radians) # 计算余弦值
print(f"Angles (degrees): {angles_degrees}")
print(f"Cos values: {cos_values}")
- 使用numpy计算正切函数
import numpy as np
angles_degrees = np.array([0, 30, 45, 60, 90]) # 角度数组
angles_radians = np.radians(angles_degrees) # 将角度数组转换为弧度数组
tan_values = np.tan(angles_radians) # 计算正切值
print(f"Angles (degrees): {angles_degrees}")
print(f"Tan values: {tan_values}")
- 使用numpy计算反三角函数
import numpy as np
sin_values = np.array([0, 0.5, 0.707, 0.866, 1]) # 正弦值数组
angles_radians = np.arcsin(sin_values) # 计算反正弦值(弧度)
angles_degrees = np.degrees(angles_radians) # 将弧度转换为角度
print(f"Sin values: {sin_values}")
print(f"Angles (degrees): {angles_degrees}")
八、总结
在Python中输入和使用三角函数是非常简单和直观的。通过导入math
模块,你可以轻松地计算正弦、余弦、正切及其反函数值。此外,了解如何在角度和弧度之间进行转换是非常重要的,因为大多数三角函数都接受弧度作为参数。
如果你需要处理大规模的科学计算,可以考虑使用numpy
库,它提供了更高效的数组操作和更丰富的函数。
总之,掌握这些基本的三角函数和转换方法将使你能够轻松应对各种数学和几何计算任务。无论是简单的角度计算还是复杂的科学计算,Python都能为你提供强大的工具和支持。
相关问答FAQs:
在Python中如何使用三角函数库?
Python提供了一个强大的数学库,名为math
,其中包含了常用的三角函数。要使用这些函数,首先需要导入math
模块。使用math.sin()
、math.cos()
和math.tan()
等函数可以计算角度的正弦、余弦和正切值。请注意,这些函数接受弧度作为输入,因此在计算前可能需要将角度转换为弧度,方法是使用math.radians()
函数。
如何在Python中处理角度与弧度之间的转换?
在进行三角函数计算时,常常需要在角度与弧度之间进行转换。在Python中,可以使用math.radians()
函数将角度转换为弧度,使用math.degrees()
函数将弧度转换为角度。例如,math.sin(math.radians(30))
可以计算30度的正弦值,结果为0.5。
Python中是否有其他库可以用于三角函数计算?
除了内置的math
库,Python还提供了其他一些库,如numpy
,专门用于科学计算和数组操作。numpy
库也包含了三角函数,使用方法类似。例如,使用numpy.sin()
和numpy.cos()
可以对数组中的每个元素同时进行三角函数计算,这在处理大量数据时非常高效。使用numpy
时,需要注意导入该库:import numpy as np
。
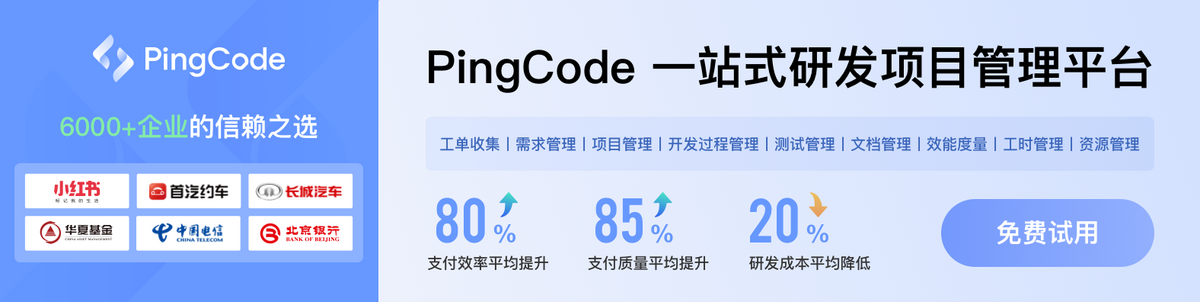