Python在一个平面显示多图的方法主要有:使用Matplotlib库的subplot功能、使用Gridspec模块、使用seaborn库等。 以下将详细展开Matplotlib库的subplot功能。
Matplotlib是Python中最常用的绘图库之一,它提供了丰富的功能来创建各种类型的图表。subplot功能使我们可以在一个平面上展示多个图表。通过调整subplot的参数,我们可以灵活地控制图表的布局和显示。
一、使用Matplotlib库的subplot功能
Matplotlib的subplot功能允许我们在一个平面上排列多个图表。通过指定行数和列数,我们可以创建一个网格,然后在每个子图中绘制不同的图表。
1、基本用法
以下是一个简单的例子,展示了如何使用subplot函数在一个平面上显示多个图表:
import matplotlib.pyplot as plt
创建一个包含2行2列的子图网格
fig, axs = plt.subplots(2, 2)
在第一个子图中绘制折线图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 0].set_title('Line Plot')
在第二个子图中绘制散点图
axs[0, 1].scatter([1, 2, 3], [1, 4, 9])
axs[0, 1].set_title('Scatter Plot')
在第三个子图中绘制条形图
axs[1, 0].bar([1, 2, 3], [1, 4, 9])
axs[1, 0].set_title('Bar Plot')
在第四个子图中绘制饼图
axs[1, 1].pie([10, 20, 30])
axs[1, 1].set_title('Pie Chart')
调整子图之间的间距
plt.tight_layout()
显示图表
plt.show()
在这个例子中,我们创建了一个2行2列的子图网格,并在每个子图中绘制了不同类型的图表。使用plt.tight_layout()
来自动调整子图之间的间距,确保图表不会重叠。
2、调整子图的大小和比例
有时候我们需要调整子图的大小和比例,以便更好地展示数据。可以使用figsize
参数来控制整个图表的大小,并使用gridspec_kw
参数来调整子图的比例。
import matplotlib.pyplot as plt
创建一个包含2行2列的子图网格,并设置图表大小
fig, axs = plt.subplots(2, 2, figsize=(10, 10), gridspec_kw={'width_ratios': [2, 1], 'height_ratios': [1, 2]})
在子图中绘制图表
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 0].set_title('Line Plot')
axs[0, 1].scatter([1, 2, 3], [1, 4, 9])
axs[0, 1].set_title('Scatter Plot')
axs[1, 0].bar([1, 2, 3], [1, 4, 9])
axs[1, 0].set_title('Bar Plot')
axs[1, 1].pie([10, 20, 30])
axs[1, 1].set_title('Pie Chart')
调整子图之间的间距
plt.tight_layout()
显示图表
plt.show()
在这个例子中,我们使用figsize
参数将整个图表的大小设置为10×10英寸,并使用gridspec_kw
参数调整子图的宽度和高度比例。
二、使用Gridspec模块
Gridspec模块提供了更多的灵活性来控制子图的布局。通过指定子图的行列跨度,我们可以创建更加复杂的布局。
1、基本用法
以下是一个示例,展示了如何使用Gridspec模块创建不规则的子图布局:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建一个包含3行3列的子图网格
fig = plt.figure(figsize=(10, 10))
gs = gridspec.GridSpec(3, 3)
在指定位置和跨度创建子图
ax1 = fig.add_subplot(gs[0, 0:2]) # 占据第1行的前两列
ax2 = fig.add_subplot(gs[0, 2]) # 占据第1行的第3列
ax3 = fig.add_subplot(gs[1:, 0]) # 占据第2行和第3行的第1列
ax4 = fig.add_subplot(gs[1, 1:]) # 占据第2行的第2列和第3列
ax5 = fig.add_subplot(gs[2, 1:]) # 占据第3行的第2列和第3列
在子图中绘制图表
ax1.plot([1, 2, 3], [1, 4, 9])
ax1.set_title('Line Plot')
ax2.scatter([1, 2, 3], [1, 4, 9])
ax2.set_title('Scatter Plot')
ax3.bar([1, 2, 3], [1, 4, 9])
ax3.set_title('Bar Plot')
ax4.pie([10, 20, 30])
ax4.set_title('Pie Chart')
ax5.hist([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
ax5.set_title('Histogram')
调整子图之间的间距
plt.tight_layout()
显示图表
plt.show()
在这个例子中,我们使用Gridspec模块创建了一个3行3列的子图网格,并通过指定子图的行列跨度来创建不规则的布局。
2、更多的布局控制
Gridspec模块还允许我们精确控制子图之间的间距和边距,可以使用GridSpec
类的update
方法来设置这些参数:
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建一个包含3行3列的子图网格
fig = plt.figure(figsize=(10, 10))
gs = gridspec.GridSpec(3, 3)
更新子图的间距和边距
gs.update(wspace=0.5, hspace=0.5, left=0.1, right=0.9, top=0.9, bottom=0.1)
在指定位置和跨度创建子图
ax1 = fig.add_subplot(gs[0, 0:2])
ax2 = fig.add_subplot(gs[0, 2])
ax3 = fig.add_subplot(gs[1:, 0])
ax4 = fig.add_subplot(gs[1, 1:])
ax5 = fig.add_subplot(gs[2, 1:])
在子图中绘制图表
ax1.plot([1, 2, 3], [1, 4, 9])
ax1.set_title('Line Plot')
ax2.scatter([1, 2, 3], [1, 4, 9])
ax2.set_title('Scatter Plot')
ax3.bar([1, 2, 3], [1, 4, 9])
ax3.set_title('Bar Plot')
ax4.pie([10, 20, 30])
ax4.set_title('Pie Chart')
ax5.hist([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
ax5.set_title('Histogram')
显示图表
plt.show()
在这个例子中,我们通过gs.update
方法设置了子图之间的水平间距(wspace)和垂直间距(hspace),以及图表的左右(left、right)和上下(top、bottom)边距。
三、使用seaborn库
Seaborn是基于Matplotlib的高级绘图库,它提供了更加简洁的API和美观的默认样式。虽然Seaborn没有直接的subplot功能,但我们可以结合使用Matplotlib的subplot功能来在一个平面上显示多个图表。
1、基本用法
以下是一个示例,展示了如何使用Seaborn库和Matplotlib的subplot功能在一个平面上显示多个图表:
import seaborn as sns
import matplotlib.pyplot as plt
加载示例数据集
tips = sns.load_dataset('tips')
创建一个包含2行2列的子图网格
fig, axs = plt.subplots(2, 2, figsize=(10, 10))
在子图中绘制图表
sns.lineplot(x='total_bill', y='tip', data=tips, ax=ax1)
axs[0, 0].set_title('Line Plot')
sns.scatterplot(x='total_bill', y='tip', data=tips, ax=ax2)
axs[0, 1].set_title('Scatter Plot')
sns.barplot(x='day', y='total_bill', data=tips, ax=ax3)
axs[1, 0].set_title('Bar Plot')
sns.histplot(tips['total_bill'], kde=True, ax=ax4)
axs[1, 1].set_title('Histogram')
调整子图之间的间距
plt.tight_layout()
显示图表
plt.show()
在这个例子中,我们使用Seaborn库加载了示例数据集,并在Matplotlib的子图中绘制了不同类型的图表。
2、组合使用Seaborn和Gridspec
我们还可以结合使用Seaborn库和Gridspec模块来创建更加复杂的子图布局:
import seaborn as sns
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
加载示例数据集
tips = sns.load_dataset('tips')
创建一个包含3行3列的子图网格
fig = plt.figure(figsize=(10, 10))
gs = gridspec.GridSpec(3, 3)
在指定位置和跨度创建子图
ax1 = fig.add_subplot(gs[0, 0:2])
ax2 = fig.add_subplot(gs[0, 2])
ax3 = fig.add_subplot(gs[1:, 0])
ax4 = fig.add_subplot(gs[1, 1:])
ax5 = fig.add_subplot(gs[2, 1:])
在子图中绘制图表
sns.lineplot(x='total_bill', y='tip', data=tips, ax=ax1)
ax1.set_title('Line Plot')
sns.scatterplot(x='total_bill', y='tip', data=tips, ax=ax2)
ax2.set_title('Scatter Plot')
sns.barplot(x='day', y='total_bill', data=tips, ax=ax3)
ax3.set_title('Bar Plot')
sns.histplot(tips['total_bill'], kde=True, ax=ax4)
ax4.set_title('Histogram')
sns.boxplot(x='day', y='total_bill', data=tips, ax=ax5)
ax5.set_title('Box Plot')
调整子图之间的间距
plt.tight_layout()
显示图表
plt.show()
在这个例子中,我们结合使用了Seaborn库和Gridspec模块,创建了一个3行3列的不规则子图布局,并在每个子图中绘制了不同类型的图表。
四、总结
通过使用Matplotlib库的subplot功能、Gridspec模块以及Seaborn库,我们可以在一个平面上显示多个图表,并灵活地控制子图的布局和显示。Matplotlib的subplot功能适用于简单的子图布局,而Gridspec模块提供了更多的灵活性来创建复杂的布局。Seaborn库虽然没有直接的subplot功能,但我们可以结合使用Matplotlib的subplot功能来在一个平面上显示多个美观的图表。
相关问答FAQs:
如何在Python中使用Matplotlib显示多个图像?
可以使用Matplotlib库中的subplot
或subplots
函数来在一个平面上显示多个图像。subplot
允许您在一个图形窗口中创建多个子图,而subplots
可以一次性创建多个子图的网格。您可以通过调整行和列的参数来改变图像的布局,并使用imshow
函数来显示图像。
在显示多个图像时,如何控制每个图像的大小和间距?
在使用subplots
时,可以通过设置figsize
参数来调整整个图形的大小。同时,可以使用plt.subplots_adjust()
函数来控制子图之间的间距,例如通过设置hspace
和wspace
参数来调整高度和宽度的间距,从而使显示效果更佳。
如何在多个图像上添加标题和标签?
可以使用set_title()
方法为每个子图添加标题,同时使用set_xlabel()
和set_ylabel()
方法为每个图像添加X轴和Y轴标签。这些方法可以帮助您更好地解释每个图像的内容和意义。
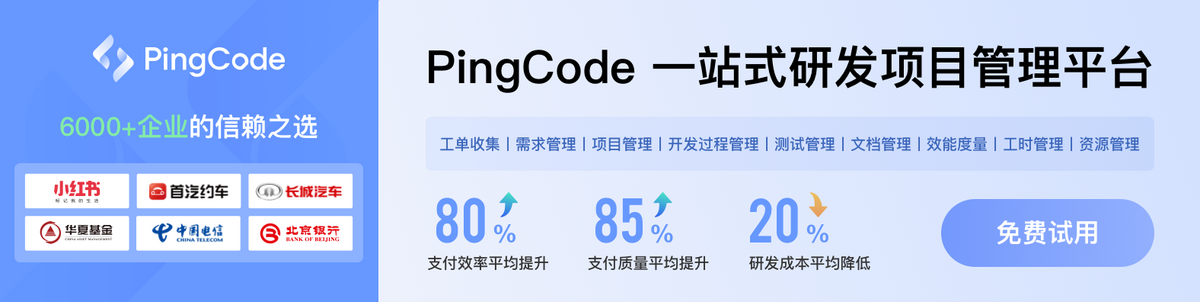