Python中给每一行加行号的方法有很多,比如使用enumerate()、循环遍历、列表推导式等。本文将详细介绍这几种方法,并通过实例演示如何在实际应用中添加行号。
一、使用 enumerate() 函数
使用 enumerate()
函数是 Python 中较为简洁的方法之一,它可以在遍历列表或任何可迭代对象时自动生成索引。
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
for index, line in enumerate(lines, start=1):
print(f"{index}: {line}")
上面的代码会输出每行的行号,结果如下:
1: This is the first line.
2: This is the second line.
3: This is the third line.
二、使用循环遍历
使用循环遍历也是一种常见的方法,适合对列表进行行号添加。
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
for i in range(len(lines)):
print(f"{i + 1}: {lines[i]}")
同样,输出结果如下:
1: This is the first line.
2: This is the second line.
3: This is the third line.
三、使用列表推导式
列表推导式不仅可以用于生成新列表,还可以用于对现有列表进行处理和修改。
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
numbered_lines = [f"{i + 1}: {line}" for i, line in enumerate(lines)]
for line in numbered_lines:
print(line)
输出结果:
1: This is the first line.
2: This is the second line.
3: This is the third line.
四、处理文件内容
在实际应用中,我们常常需要对文件的每一行添加行号。以下是一个读取文件并添加行号的实例:
with open('input.txt', 'r') as file:
lines = file.readlines()
with open('output.txt', 'w') as file:
for index, line in enumerate(lines, start=1):
file.write(f"{index}: {line}")
这个代码段会读取 input.txt
文件,给每一行添加行号,并将结果写入 output.txt
文件。
五、在 Pandas DataFrame 中添加行号
在数据处理领域,Pandas 是一个强大的工具库。我们可以轻松地在 DataFrame 中添加行号。
import pandas as pd
data = {
"text": [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
}
df = pd.DataFrame(data)
df['line_number'] = range(1, len(df) + 1)
print(df)
输出结果:
text line_number
0 This is the first line. 1
1 This is the second line. 2
2 This is the third line. 3
六、使用生成器函数
生成器函数是一种高效处理大数据的方法。我们可以使用生成器函数为每一行添加行号。
def add_line_numbers(lines):
for index, line in enumerate(lines, start=1):
yield f"{index}: {line}"
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
for line in add_line_numbers(lines):
print(line)
这个方法的优点是节省内存,适用于处理大量数据。
七、在 Jupyter Notebook 中添加行号
在 Jupyter Notebook 中,我们可以使用 IPython 的 magic 命令来添加行号。
from IPython.display import display, HTML
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
display(HTML("<br>".join([f"{i + 1}: {line}" for i, line in enumerate(lines)])))
这个方法可以让你在 Jupyter Notebook 中美观地显示带行号的文本。
八、使用正则表达式
正则表达式是一种强大的文本处理工具,可以用于复杂文本操作。
import re
text = """
This is the first line.
This is the second line.
This is the third line.
"""
lines = text.strip().split('\n')
numbered_lines = [f"{i + 1}: {line}" for i, line in enumerate(lines)]
numbered_text = "\n".join(numbered_lines)
print(numbered_text)
输出结果:
1: This is the first line.
2: This is the second line.
3: This is the third line.
九、使用 Numpy 库
Numpy 是一个高性能的科学计算库,可以用于处理数组和矩阵。
import numpy as np
lines = np.array([
"This is the first line.",
"This is the second line.",
"This is the third line."
])
for i in range(len(lines)):
print(f"{i + 1}: {lines[i]}")
输出结果:
1: This is the first line.
2: This is the second line.
3: This is the third line.
十、总结
在 Python 中给每一行加行号的方法多种多样,使用 enumerate() 函数、循环遍历、列表推导式、处理文件内容、在 Pandas DataFrame 中添加行号、使用生成器函数、在 Jupyter Notebook 中添加行号、使用正则表达式、使用 Numpy 库等方法都可以有效地实现这一需求。根据具体的应用场景和数据规模,选择合适的方法可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python中为每一行添加行号?
在Python中,可以通过读取文件内容并使用枚举函数为每一行添加行号。使用enumerate()
函数可以轻松实现这一点,它为每一行提供一个索引,从而能够在输出中添加行号。
是否可以在输出中自定义行号的起始值?
是的,enumerate()
函数允许您设置起始值。通过传递第二个参数,可以指定行号从特定的数字开始。例如,如果希望行号从1开始,可以使用enumerate(lines, start=1)
。
如何处理大文件以添加行号而不占用过多内存?
在处理大文件时,可以逐行读取文件,而不是一次性加载整个文件内容到内存中。使用with open('filename.txt') as file:
结构,结合enumerate()
函数,可以有效地为每一行添加行号,而不会消耗过多内存。这样的方法适合处理大文件,确保程序运行高效且稳定。
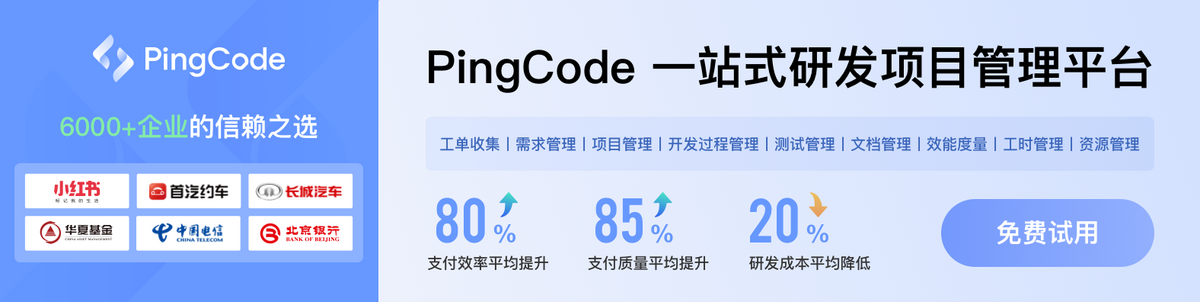