Python在列表中取出最大的数字:使用内置的max()函数、使用循环遍历、使用sorted()函数。其中使用内置的max()函数是最常用且最简便的方法。内置函数max()用于返回列表中的最大值。接下来,我们将详细描述如何使用这些方法。
一、使用内置的max()函数
Python提供了一个内置函数max(),可以直接用于找到列表中的最大值。这是最简便和直接的方法。
numbers = [3, 5, 7, 2, 8, 9]
max_number = max(numbers)
print("The maximum number in the list is:", max_number)
在这个例子中,max()
函数直接返回列表numbers
中的最大值9
。
二、使用循环遍历
除了使用内置函数,还可以通过循环遍历的方式找到列表中的最大值。这种方法适用于更复杂的情况,例如需要在遍历时执行额外的操作。
- 定义一个变量来存储最大值,初始值设置为列表中的第一个元素。
- 使用for循环遍历列表中的每一个元素,比较当前元素和当前最大值,如果当前元素大于当前最大值,则更新最大值。
- 遍历结束后,当前最大值即为列表中的最大值。
numbers = [3, 5, 7, 2, 8, 9]
max_number = numbers[0]
for number in numbers:
if number > max_number:
max_number = number
print("The maximum number in the list is:", max_number)
这个方法虽然多了几行代码,但能够让你更好地理解最大值的查找过程。
三、使用sorted()函数
sorted()函数用于对列表进行排序,默认情况下是升序排序。我们可以利用这一点来获取列表中的最大值,即排序后的最后一个元素。
numbers = [3, 5, 7, 2, 8, 9]
sorted_numbers = sorted(numbers)
max_number = sorted_numbers[-1]
print("The maximum number in the list is:", max_number)
在这个例子中,sorted()
函数将列表numbers
按升序排序,sorted_numbers[-1]
即为排序后的最后一个元素,亦即列表中的最大值。
四、使用numpy库中的amax()函数
如果你在使用NumPy库,NumPy提供了更高效的数组操作方法。可以使用numpy.amax()
函数来找到数组中的最大值。
import numpy as np
numbers = np.array([3, 5, 7, 2, 8, 9])
max_number = np.amax(numbers)
print("The maximum number in the list is:", max_number)
在这个例子中,numpy.amax()
函数返回NumPy数组numbers
中的最大值。
五、使用reduce()函数
Python的functools模块提供了reduce()函数,它可以将一个二元操作函数作用在序列的每一个元素上,从而得到一个累计结果。
from functools import reduce
numbers = [3, 5, 7, 2, 8, 9]
max_number = reduce(lambda x, y: x if x > y else y, numbers)
print("The maximum number in the list is:", max_number)
在这个例子中,reduce()
函数使用了一个lambda函数来比较两个元素的大小,从而最终返回列表中的最大值。
六、使用pandas库
如果你正在处理的数据是用pandas库的DataFrame表示的,那么可以使用pandas中的max()函数。
import pandas as pd
df = pd.DataFrame({'numbers': [3, 5, 7, 2, 8, 9]})
max_number = df['numbers'].max()
print("The maximum number in the list is:", max_number)
在这个例子中,df['numbers'].max()
返回DataFrame中numbers
列的最大值。
七、结合多个方法
有时,你可能需要结合多种方法来找到最大值。例如,你可能需要在查找最大值的过程中同时记录出现的次数,或者在查找最大值后进行一些其他操作。
numbers = [3, 5, 7, 2, 8, 9]
max_number = numbers[0]
count = 1
for number in numbers[1:]:
if number > max_number:
max_number = number
count = 1
elif number == max_number:
count += 1
print("The maximum number in the list is:", max_number)
print("The maximum number appears", count, "times")
在这个例子中,我们不仅找到了列表中的最大值,还记录了最大值出现的次数。
总结
通过以上几种方法,我们可以看到在Python中找到列表中的最大值有多种实现方式。使用内置的max()函数是最简便的方法,但在特定的情况下,其他方法如循环遍历、sorted()函数、NumPy库、reduce()函数和pandas库也有其独特的优势。根据具体需求,选择最合适的方法来找到列表中的最大值。
相关问答FAQs:
如何在Python列表中找到最大值?
在Python中,可以使用内置的max()
函数轻松找到列表中的最大值。例如,给定一个列表numbers = [3, 5, 1, 8, 2]
,你可以通过max_value = max(numbers)
来获取最大的数字,这会返回8。
如果列表中包含负数,如何找到最大的数字?
即使列表中包含负数,max()
函数依然能够准确地返回最大值。比如,如果有一个列表numbers = [-10, -5, -3, -1]
,调用max(numbers)
将返回-1,这是这个列表中的最大值。
在列表中查找最大值时,如何处理重复的最大值?
当列表中存在多个相同的最大值时,max()
函数仍然会返回这个最大值。例如,在列表numbers = [4, 4, 2, 4]
中,调用max(numbers)
会返回4。若需要找出最大值的所有索引,可以使用列表推导式结合max()
函数来实现。
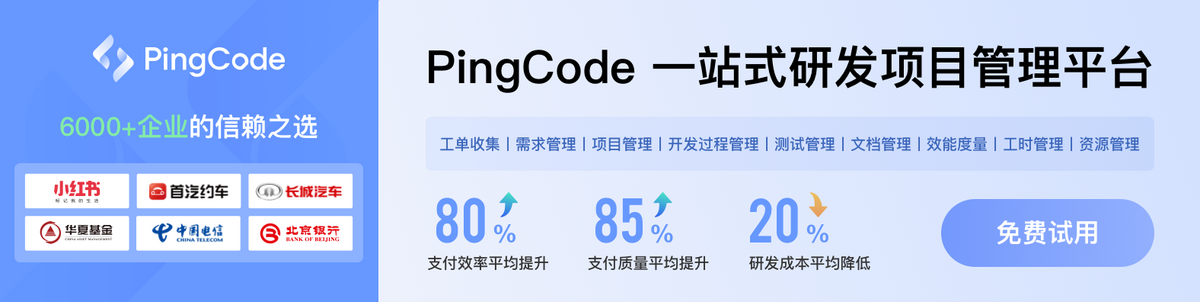