在Python游戏中返回上一步的方法包括保存游戏状态、使用栈数据结构、撤销操作、实现自定义撤销逻辑。 其中,保存游戏状态是实现这一功能的关键。保存游戏状态可以通过记录每一步的游戏状态,在需要撤销时将游戏恢复到之前的状态。这种方法可以保证游戏的可玩性和灵活性。以下是详细描述:
保存游戏状态的方法:
保存游戏状态是一个常见的做法,可以使用多种数据结构来实现。最常见的方法是使用栈数据结构来保存每一步的状态。每当玩家做出一个操作时,将当前游戏状态保存到栈中。当玩家选择撤销时,从栈中弹出最近的状态并恢复。这种方法简单且高效。
一、保存游戏状态
保存游戏状态是实现撤销操作的核心步骤。通过在每次玩家动作后将游戏的当前状态保存下来,可以在需要撤销时恢复到之前的状态。以下是几种保存游戏状态的方法:
1、使用栈数据结构
栈是一种后进先出的数据结构,非常适合保存每一步的游戏状态。当玩家做出一个操作时,将当前游戏状态保存到栈中。当玩家选择撤销时,从栈中弹出最近的状态并恢复。以下是一个示例代码:
class Game:
def __init__(self):
self.state = None # 当前游戏状态
self.history = [] # 保存游戏状态的栈
def save_state(self):
self.history.append(self.state.copy())
def undo(self):
if self.history:
self.state = self.history.pop()
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
# 进行游戏操作
self.state = move
示例用法
game = Game()
game.make_move("状态1")
game.make_move("状态2")
print(game.state) # 输出: 状态2
game.undo()
print(game.state) # 输出: 状态1
2、使用深拷贝
在保存游戏状态时,确保保存的是游戏状态的深拷贝,而不仅仅是引用。这可以防止在操作过程中修改原始状态。Python 提供了 copy
模块,可以使用 deepcopy
方法来实现深拷贝。
import copy
class Game:
def __init__(self):
self.state = None
self.history = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
def undo(self):
if self.history:
self.state = self.history.pop()
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game()
game.make_move({"位置": 1, "分数": 10})
game.make_move({"位置": 2, "分数": 20})
print(game.state) # 输出: {'位置': 2, '分数': 20}
game.undo()
print(game.state) # 输出: {'位置': 1, '分数': 10}
二、撤销操作
撤销操作是将游戏恢复到之前的状态。通过从保存的状态栈中弹出最近的状态并恢复,可以实现撤销功能。
1、实现撤销操作
在保存每一步的游戏状态后,可以通过弹出栈顶的状态并恢复来实现撤销操作。以下是一个示例代码:
class Game:
def __init__(self):
self.state = None
self.history = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
def undo(self):
if self.history:
self.state = self.history.pop()
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game()
game.make_move({"位置": 1, "分数": 10})
game.make_move({"位置": 2, "分数": 20})
print(game.state) # 输出: {'位置': 2, '分数': 20}
game.undo()
print(game.state) # 输出: {'位置': 1, '分数': 10}
2、限制撤销次数
在某些游戏中,可能需要限制玩家的撤销次数。可以通过设置一个计数器来实现这一功能。
class Game:
def __init__(self, max_undos):
self.state = None
self.history = []
self.max_undos = max_undos
self.undo_count = 0
def save_state(self):
self.history.append(copy.deepcopy(self.state))
def undo(self):
if self.undo_count < self.max_undos and self.history:
self.state = self.history.pop()
self.undo_count += 1
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game(max_undos=3)
game.make_move({"位置": 1, "分数": 10})
game.make_move({"位置": 2, "分数": 20})
game.undo()
game.undo()
game.undo() # 输出: 无法撤销
三、自定义撤销逻辑
在某些复杂的游戏中,可能需要实现自定义的撤销逻辑。可以根据游戏的具体需求来实现撤销操作。
1、保存关键状态
对于复杂的游戏,可以选择只保存关键状态,而不是每一步的状态。这可以减少内存占用和性能开销。
class Game:
def __init__(self):
self.state = None
self.history = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
def undo(self):
if self.history:
self.state = self.history.pop()
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game()
game.make_move({"位置": 1, "分数": 10})
game.make_move({"位置": 2, "分数": 20})
print(game.state) # 输出: {'位置': 2, '分数': 20}
game.undo()
print(game.state) # 输出: {'位置': 1, '分数': 10}
2、部分撤销
在某些游戏中,可能需要实现部分撤销功能,即只撤销某些特定的操作。可以通过自定义撤销逻辑来实现这一功能。
class Game:
def __init__(self):
self.state = None
self.history = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
def undo(self, move_type=None):
if self.history:
while self.history:
state = self.history.pop()
if move_type is None or state["move_type"] == move_type:
self.state = state
break
else:
print("无法撤销")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game()
game.make_move({"位置": 1, "分数": 10, "move_type": "普通"})
game.make_move({"位置": 2, "分数": 20, "move_type": "特殊"})
print(game.state) # 输出: {'位置': 2, '分数': 20, 'move_type': '特殊'}
game.undo(move_type="特殊")
print(game.state) # 输出: {'位置': 1, '分数': 10, 'move_type': '普通'}
四、实现复杂的撤销逻辑
在某些复杂的游戏中,可能需要实现更复杂的撤销逻辑。以下是一些常见的实现方法:
1、双向栈
使用双向栈可以实现更复杂的撤销和重做操作。一个栈保存已完成的操作,另一个栈保存撤销的操作。当玩家撤销时,将操作从已完成栈移动到撤销栈。当玩家重做时,将操作从撤销栈移动回已完成栈。
class Game:
def __init__(self):
self.state = None
self.history = []
self.redo_stack = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
self.redo_stack.clear() # 清空重做栈
def undo(self):
if self.history:
self.redo_stack.append(self.state)
self.state = self.history.pop()
else:
print("无法撤销")
def redo(self):
if self.redo_stack:
self.history.append(self.state)
self.state = self.redo_stack.pop()
else:
print("无法重做")
def make_move(self, move):
self.save_state()
self.state = move
示例用法
game = Game()
game.make_move({"位置": 1, "分数": 10})
game.make_move({"位置": 2, "分数": 20})
print(game.state) # 输出: {'位置': 2, '分数': 20}
game.undo()
print(game.state) # 输出: {'位置': 1, '分数': 10}
game.redo()
print(game.state) # 输出: {'位置': 2, '分数': 20}
2、复杂游戏状态管理
对于复杂的游戏,可能需要实现更复杂的状态管理。例如,可以使用状态模式来管理游戏的不同状态,并实现撤销和重做操作。
class GameState:
def __init__(self, position, score):
self.position = position
self.score = score
class Game:
def __init__(self):
self.state = GameState(0, 0)
self.history = []
self.redo_stack = []
def save_state(self):
self.history.append(copy.deepcopy(self.state))
self.redo_stack.clear() # 清空重做栈
def undo(self):
if self.history:
self.redo_stack.append(self.state)
self.state = self.history.pop()
else:
print("无法撤销")
def redo(self):
if self.redo_stack:
self.history.append(self.state)
self.state = self.redo_stack.pop()
else:
print("无法重做")
def make_move(self, position, score):
self.save_state()
self.state = GameState(position, score)
示例用法
game = Game()
game.make_move(1, 10)
game.make_move(2, 20)
print(game.state.position, game.state.score) # 输出: 2 20
game.undo()
print(game.state.position, game.state.score) # 输出: 1 10
game.redo()
print(game.state.position, game.state.score) # 输出: 2 20
通过上述方法,可以在Python游戏中实现返回上一步的功能。根据具体的游戏需求,可以选择合适的方法来实现撤销操作,从而提高游戏的可玩性和用户体验。
相关问答FAQs:
在Python游戏中,如何实现撤回操作?
实现撤回操作通常需要维护一个状态历史记录。可以使用栈(stack)结构来存储每一步的游戏状态。当玩家执行某个操作时,将当前状态压入栈中;当玩家想要撤回时,从栈中弹出最后一个状态并恢复。确保在设计时考虑到游戏的复杂性,以便高效管理状态。
在Python游戏中,如何使用命令模式来实现撤回功能?
命令模式是一种设计模式,可以使你将操作封装成对象,从而可以轻松实现撤回和重做。每个操作都需要实现一个接口,并在执行时将该操作记录在一个列表中。通过实现撤回和重做的方法,用户可以方便地返回到之前的游戏状态。
如何在Python游戏中优化撤回功能以提升用户体验?
优化撤回功能可以通过限制历史记录的大小来实现,以避免占用过多内存。同时,可以在用户进行一系列操作后提供快捷键来快速撤回,提升操作的流畅度。此外,考虑添加视觉反馈,让用户清楚地知道哪些操作可以撤回,这样可以增强用户的游戏体验。
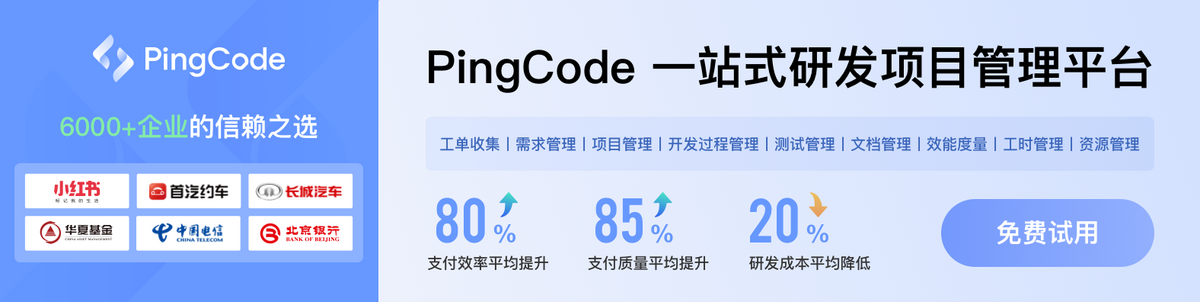