在Python中,替换文件中的敏感词可以通过读取文件内容、使用字符串的替换方法、并将替换后的内容写回文件来实现。首先,可以通过读取文件内容并识别敏感词,然后使用字符串的replace方法替换敏感词,最后将修改后的内容写回文件。主要步骤包括:读取文件内容、替换敏感词、写回文件。 下面将详细介绍如何实现这一过程。
一、读取文件内容
首先需要读取文件内容,可以使用Python的内置函数open
来打开文件,并使用read
方法读取文件内容。以下是一个示例代码:
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
这个函数接收一个文件路径作为参数,打开文件并读取其内容,然后返回读取到的内容。
二、替换敏感词
接下来,我们需要定义敏感词列表,并使用字符串的replace
方法将敏感词替换为指定的替换字符(例如,*
)。以下是一个示例代码:
def replace_sensitive_words(content, sensitive_words, replacement="*"):
for word in sensitive_words:
content = content.replace(word, replacement * len(word))
return content
这个函数接收文件内容、敏感词列表和替换字符作为参数,遍历敏感词列表并将每个敏感词替换为指定的替换字符,最后返回替换后的内容。
三、写回文件
最后,我们需要将替换后的内容写回文件,可以使用open
函数的写模式来实现。以下是一个示例代码:
def write_file(file_path, content):
with open(file_path, 'w', encoding='utf-8') as file:
file.write(content)
这个函数接收文件路径和内容作为参数,打开文件并将内容写入文件。
四、完整的实现流程
将上述步骤整合在一起,形成一个完整的实现流程:
def replace_sensitive_words_in_file(file_path, sensitive_words, replacement="*"):
# 读取文件内容
content = read_file(file_path)
# 替换敏感词
content = replace_sensitive_words(content, sensitive_words, replacement)
# 写回文件
write_file(file_path, content)
五、示例代码
以下是一个完整的示例代码,演示如何替换文件中的敏感词:
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
def replace_sensitive_words(content, sensitive_words, replacement="*"):
for word in sensitive_words:
content = content.replace(word, replacement * len(word))
return content
def write_file(file_path, content):
with open(file_path, 'w', encoding='utf-8') as file:
file.write(content)
def replace_sensitive_words_in_file(file_path, sensitive_words, replacement="*"):
content = read_file(file_path)
content = replace_sensitive_words(content, sensitive_words, replacement)
write_file(file_path, content)
示例使用
file_path = 'example.txt'
sensitive_words = ['badword1', 'badword2', 'badword3']
replace_sensitive_words_in_file(file_path, sensitive_words)
这个示例代码演示了如何定义敏感词列表,并调用replace_sensitive_words_in_file
函数来替换文件中的敏感词。
六、处理更复杂的情况
上述示例适用于简单的敏感词替换,但在实际应用中可能需要处理更复杂的情况,例如:
- 区分大小写:可以使用正则表达式来实现区分大小写的替换。
- 部分匹配:可以使用正则表达式来匹配敏感词的一部分,并进行替换。
- 多文件处理:可以扩展代码以处理多个文件中的敏感词替换。
区分大小写
为了区分大小写,可以使用Python的re
模块进行正则表达式替换。以下是一个示例代码:
import re
def replace_sensitive_words_case_insensitive(content, sensitive_words, replacement="*"):
for word in sensitive_words:
pattern = re.compile(re.escape(word), re.IGNORECASE)
content = pattern.sub(replacement * len(word), content)
return content
这个函数使用正则表达式来匹配敏感词,并进行区分大小写的替换。
部分匹配
为了实现部分匹配,可以使用正则表达式来匹配敏感词的一部分。以下是一个示例代码:
def replace_partial_sensitive_words(content, sensitive_patterns, replacement="*"):
for pattern in sensitive_patterns:
content = re.sub(pattern, replacement, content)
return content
这个函数接收敏感词模式列表,并使用正则表达式替换匹配到的部分内容。
多文件处理
为了处理多个文件中的敏感词替换,可以扩展代码以遍历文件列表,并对每个文件进行替换。以下是一个示例代码:
def replace_sensitive_words_in_files(file_paths, sensitive_words, replacement="*"):
for file_path in file_paths:
replace_sensitive_words_in_file(file_path, sensitive_words, replacement)
示例使用
file_paths = ['example1.txt', 'example2.txt', 'example3.txt']
sensitive_words = ['badword1', 'badword2', 'badword3']
replace_sensitive_words_in_files(file_paths, sensitive_words)
这个示例代码演示了如何定义文件路径列表,并调用replace_sensitive_words_in_files
函数来替换多个文件中的敏感词。
七、总结
在Python中,替换文件中的敏感词可以通过读取文件内容、使用字符串的替换方法、并将替换后的内容写回文件来实现。具体步骤包括读取文件内容、替换敏感词、写回文件。可以使用正则表达式来处理更复杂的情况,例如区分大小写和部分匹配。扩展代码还可以处理多个文件中的敏感词替换。
相关问答FAQs:
如何在Python中读取文件内容并替换敏感词?
在Python中,可以使用内置的文件操作函数来读取文件内容。通过打开文件并读取其内容后,可以利用字符串的replace()
方法来替换敏感词。替换后的内容可以再写回到文件中,或者保存到新的文件中。以下是一个简单的示例代码:
with open('file.txt', 'r', encoding='utf-8') as file:
content = file.read()
sensitive_words = {'敏感词1': '替换词1', '敏感词2': '替换词2'}
for word, replacement in sensitive_words.items():
content = content.replace(word, replacement)
with open('file.txt', 'w', encoding='utf-8') as file:
file.write(content)
可以使用正则表达式来替换文件中的敏感词吗?
是的,使用re
模块可以通过正则表达式更加灵活地替换敏感词。正则表达式允许你使用模式匹配来识别并替换多个相似的敏感词,这在处理变体或拼写错误时尤其有用。以下是一个示例:
import re
with open('file.txt', 'r', encoding='utf-8') as file:
content = file.read()
sensitive_words = {'敏感词1': '替换词1', '敏感词2': '替换词2'}
pattern = re.compile('|'.join(re.escape(key) for key in sensitive_words.keys()))
def replace(match):
return sensitive_words[match.group(0)]
content = pattern.sub(replace, content)
with open('file.txt', 'w', encoding='utf-8') as file:
file.write(content)
如何确保在替换敏感词时不会破坏文件的原始格式?
为了在替换敏感词的同时保持文件的原始格式,可以在替换之前先将文件内容读取到一个变量中,进行替换后再写回文件。确保使用相同的编码方式(如UTF-8)打开文件,以避免字符编码问题。此外,使用文本编辑器查看文件前后内容,确保格式和结构未受影响。如果文件包含特定的格式(如Markdown或HTML),可以使用相应的解析库来处理,以避免意外更改格式。
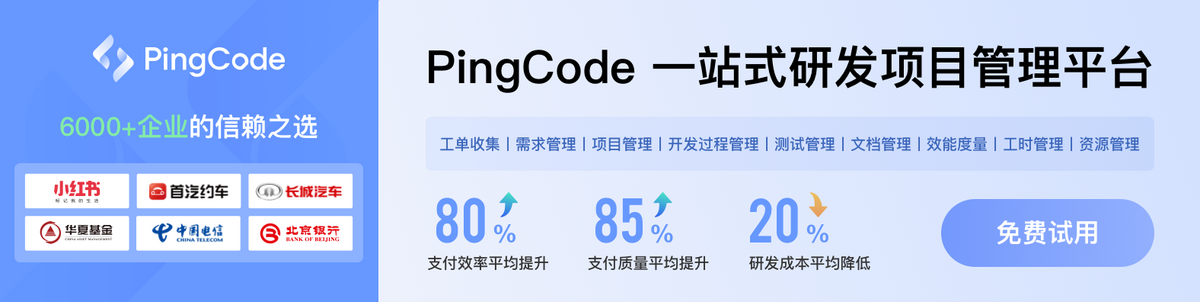