回答标题:如何用python制作出搜索引擎
用Python制作一个搜索引擎的核心步骤包括:数据收集、数据预处理、索引构建、查询处理、结果排序。首先,数据收集是搜索引擎的基础,通过网络爬虫获取网页数据。然后,进行数据预处理,包括清洗和解析。接下来,构建倒排索引,使搜索更加高效。查询处理阶段,解析用户输入的查询语句并在索引中查找相关文档。最后,通过特定的排序算法对结果进行排序展示。下面将详细介绍每个步骤。
一、数据收集
数据收集是搜索引擎的基础,通常通过网络爬虫获取网页数据。Python中常用的爬虫框架有Scrapy和Beautiful Soup。
1、使用Scrapy进行数据收集
Scrapy是一个功能强大的爬虫框架,支持多线程爬取,能够处理复杂的网页结构。使用Scrapy编写爬虫时,首先需要安装Scrapy:
pip install scrapy
然后,创建一个新的Scrapy项目:
scrapy startproject search_engine
cd search_engine
scrapy genspider example example.com
在生成的spider文件中,编写爬取网页的逻辑:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.css('title::text').getall():
yield {'title': title}
运行爬虫:
scrapy crawl example
2、使用Beautiful Soup进行数据收集
Beautiful Soup是一个用于解析HTML和XML文档的库,适合处理简单的网页结构。首先,安装Beautiful Soup和requests库:
pip install beautifulsoup4 requests
编写爬虫逻辑:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for title in soup.find_all('title'):
print(title.get_text())
二、数据预处理
数据预处理是指对收集到的数据进行清洗和解析,以便后续的索引构建和查询处理。常见的预处理步骤包括去除HTML标签、去除停用词、词干提取等。
1、去除HTML标签
可以使用Beautiful Soup或者正则表达式去除HTML标签:
from bs4 import BeautifulSoup
def remove_html_tags(text):
soup = BeautifulSoup(text, 'html.parser')
return soup.get_text()
text = '<html><body><p>Example text.</p></body></html>'
clean_text = remove_html_tags(text)
print(clean_text)
或者使用正则表达式:
import re
def remove_html_tags(text):
clean = re.compile('<.*?>')
return re.sub(clean, '', text)
text = '<html><body><p>Example text.</p></body></html>'
clean_text = remove_html_tags(text)
print(clean_text)
2、去除停用词
停用词是指在文本处理中常见但对实际意义贡献较小的词汇,如“的”、“是”、“在”等。可以使用NLTK库提供的停用词列表:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
def remove_stop_words(text):
words = text.split()
filtered_words = [word for word in words if word.lower() not in stop_words]
return ' '.join(filtered_words)
text = 'This is an example of text with stopwords.'
clean_text = remove_stop_words(text)
print(clean_text)
3、词干提取
词干提取是指将单词还原为其词根形式,以便统一处理不同形式的单词。可以使用NLTK库提供的词干提取算法:
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
def stem_words(text):
words = text.split()
stemmed_words = [stemmer.stem(word) for word in words]
return ' '.join(stemmed_words)
text = 'running runs ran'
stemmed_text = stem_words(text)
print(stemmed_text)
三、索引构建
索引构建是搜索引擎的核心,通过构建倒排索引,可以快速定位包含查询词的文档。倒排索引是指记录每个词出现在哪些文档中的数据结构。
1、构建倒排索引
可以使用Python的字典数据结构来存储倒排索引。首先,定义一个函数来构建索引:
def build_index(docs):
index = {}
for doc_id, text in enumerate(docs):
words = text.split()
for word in words:
if word not in index:
index[word] = []
index[word].append(doc_id)
return index
docs = ['this is a test', 'this is another test', 'test this']
index = build_index(docs)
print(index)
2、存储和加载索引
为了提高查询效率,可以将构建的索引存储到文件中,并在查询时加载。可以使用pickle库进行序列化和反序列化:
import pickle
def save_index(index, filename):
with open(filename, 'wb') as f:
pickle.dump(index, f)
def load_index(filename):
with open(filename, 'rb') as f:
return pickle.load(f)
save_index(index, 'index.pkl')
loaded_index = load_index('index.pkl')
print(loaded_index)
四、查询处理
查询处理是指解析用户输入的查询语句,并在索引中查找相关文档。查询处理的关键在于解析查询语句,并在索引中查找包含查询词的文档。
1、解析查询语句
可以使用NLTK库对查询语句进行预处理,如去除停用词和词干提取:
def preprocess_query(query):
query = remove_html_tags(query)
query = remove_stop_words(query)
query = stem_words(query)
return query
query = 'running is fun'
preprocessed_query = preprocess_query(query)
print(preprocessed_query)
2、查找相关文档
在索引中查找包含查询词的文档:
def search(query, index):
query_words = query.split()
result = []
for word in query_words:
if word in index:
result.extend(index[word])
return set(result)
query = 'test this'
results = search(preprocessed_query, index)
print(results)
五、结果排序
结果排序是指根据特定的排序算法,对查找到的文档进行排序展示。常见的排序算法包括TF-IDF和PageRank。
1、TF-IDF排序
TF-IDF(Term Frequency-Inverse Document Frequency)是一种常用的文本检索算法,用于衡量词语在文档中的重要性。首先,计算词频(TF)和逆文档频率(IDF):
import math
def compute_tf(doc):
tf = {}
words = doc.split()
for word in words:
if word not in tf:
tf[word] = 0
tf[word] += 1
for word in tf:
tf[word] /= len(words)
return tf
def compute_idf(docs):
idf = {}
total_docs = len(docs)
for doc in docs:
words = set(doc.split())
for word in words:
if word not in idf:
idf[word] = 0
idf[word] += 1
for word in idf:
idf[word] = math.log(total_docs / idf[word])
return idf
docs = ['this is a test', 'this is another test', 'test this']
tf = [compute_tf(doc) for doc in docs]
idf = compute_idf(docs)
print(tf)
print(idf)
然后,计算TF-IDF值,并根据TF-IDF值排序文档:
def compute_tfidf(tf, idf):
tfidf = {}
for word in tf:
tfidf[word] = tf[word] * idf.get(word, 0)
return tfidf
def rank_documents(query, docs, index):
tf = [compute_tf(doc) for doc in docs]
idf = compute_idf(docs)
tfidf_docs = [compute_tfidf(doc_tf, idf) for doc_tf in tf]
query_words = query.split()
scores = []
for doc_id, tfidf in enumerate(tfidf_docs):
score = sum(tfidf.get(word, 0) for word in query_words)
scores.append((score, doc_id))
scores.sort(reverse=True, key=lambda x: x[0])
return [doc_id for score, doc_id in scores]
query = 'test this'
ranked_results = rank_documents(preprocessed_query, docs, index)
print(ranked_results)
通过上述步骤,我们可以使用Python制作一个基本的搜索引擎。实际应用中,还需要考虑更多的细节和优化,如处理大规模数据、提升查询效率、改进排序算法等。希望这篇文章对你理解和实现搜索引擎有所帮助。
相关问答FAQs:
如何开始使用Python制作一个简单的搜索引擎?
制作搜索引擎的第一步是理解其基本组件。你需要一个爬虫来抓取网页,一个索引器来整理和存储数据,以及一个查询处理器来处理用户的搜索请求。在Python中,可以使用库如Beautiful Soup或Scrapy来实现网页抓取,使用SQLite或Elasticsearch来存储索引数据。
在制作搜索引擎时,如何处理和存储大量数据?
处理和存储大量数据时,选择合适的数据库非常重要。SQLite适合小型项目,而Elasticsearch则可以处理大规模数据并提供快速的搜索能力。此外,可以考虑使用Redis作为缓存层,以加速查询响应时间,提升用户体验。
如何提高搜索引擎的搜索结果质量?
提高搜索结果质量可以通过多种方式实现。首先,优化索引算法,确保相关性高的内容优先显示。其次,可以实现自然语言处理(NLP)技术,以更好地理解用户查询的意图。此外,用户反馈机制也非常重要,收集用户的点击数据和反馈信息,实时调整算法,以提升搜索结果的精准度。
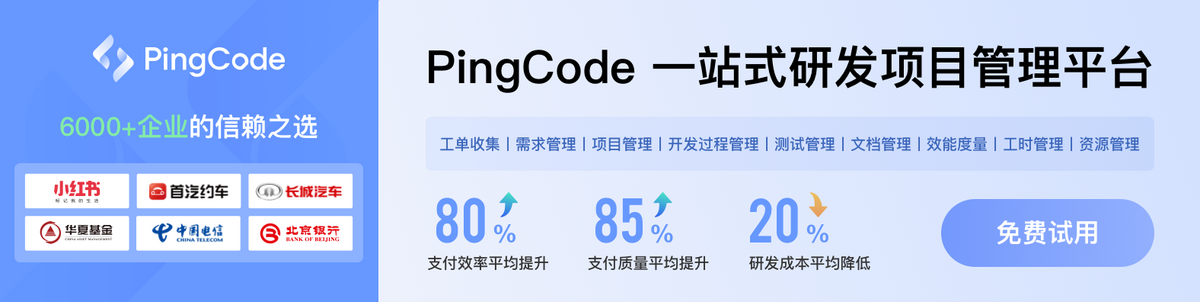