在Python中,删除最后的换行符可以使用字符串的rstrip()方法、切片操作或正则表达式等方式。 在此,我们将详细介绍其中的一种方法,即使用rstrip()方法。
使用rstrip()方法可以轻松删除字符串末尾的换行符。该方法会删除字符串末尾的所有空白字符,包括空格、制表符和换行符。我们可以通过将参数传递给rstrip()方法来专门删除换行符。
def remove_last_newline(string):
return string.rstrip('\n')
示例
example_string = "Hello, World!\n"
cleaned_string = remove_last_newline(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
一、使用rstrip()方法
字符串的rstrip()方法是最常用的删除末尾换行符的方法之一。该方法不仅能够删除换行符,还可以删除其他空白字符。
def remove_last_newline(string):
return string.rstrip('\n')
example_string = "Hello, World!\n"
cleaned_string = remove_last_newline(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
在上面的示例中,rstrip('\n')
会删除字符串末尾的换行符。如果字符串末尾没有换行符,该方法不会对字符串做任何修改。
二、使用切片操作
切片操作也是删除字符串末尾字符的一种方法。切片操作可以用于删除字符串的最后一个字符,如果该字符是换行符。
def remove_last_newline(string):
if string.endswith('\n'):
return string[:-1]
return string
example_string = "Hello, World!\n"
cleaned_string = remove_last_newline(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
在上面的示例中,string[:-1]
会返回一个新的字符串,去掉了最后一个字符。如果最后一个字符是换行符,该方法将成功删除该换行符。
三、使用正则表达式
正则表达式提供了更强大的字符串操作功能,可以用于删除字符串末尾的换行符。我们可以使用re模块来执行这个操作。
import re
def remove_last_newline(string):
return re.sub(r'\n$', '', string)
example_string = "Hello, World!\n"
cleaned_string = remove_last_newline(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
在上面的示例中,re.sub(r'\n$', '', string)
会将字符串末尾的换行符替换为空字符串。正则表达式r'\n$'
匹配字符串末尾的换行符。
四、使用自定义函数
有时,为了处理一些特定的需求,我们可能需要编写自己的函数来删除字符串末尾的换行符。以下是一个示例,该函数可以删除字符串末尾的所有换行符:
def remove_all_trailing_newlines(string):
while string.endswith('\n'):
string = string[:-1]
return string
example_string = "Hello, World!\n\n\n"
cleaned_string = remove_all_trailing_newlines(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
在上面的示例中,while string.endswith('\n')
会不断删除字符串末尾的换行符,直到字符串末尾不再有换行符为止。
五、处理文件中的换行符
在实际应用中,我们经常需要处理文件中的换行符。以下是一个示例,展示如何删除文件每行末尾的换行符并将结果写入另一个文件:
def remove_newlines_from_file(input_file, output_file):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
cleaned_line = line.rstrip('\n')
outfile.write(cleaned_line + '\n')
input_file = 'input.txt'
output_file = 'output.txt'
remove_newlines_from_file(input_file, output_file)
在上面的示例中,line.rstrip('\n')
会删除每行末尾的换行符,然后将处理后的行写入输出文件。
六、使用字符串库的strip()方法
Python的内置字符串库提供了一个strip()方法,可以删除字符串两端的空白字符,包括换行符。虽然不是专门用于删除末尾换行符,但它在某些情况下也非常有用:
def remove_trailing_whitespace(string):
return string.strip()
example_string = " Hello, World! \n"
cleaned_string = remove_trailing_whitespace(example_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!'
在上面的示例中,string.strip()
会删除字符串两端的空白字符,包括换行符。
七、处理多行字符串
在某些情况下,我们需要处理多行字符串,删除每行末尾的换行符。以下是一个示例,展示如何删除多行字符串中每行末尾的换行符:
def remove_newlines_from_multiline_string(multiline_string):
lines = multiline_string.splitlines()
cleaned_lines = [line.rstrip('\n') for line in lines]
return '\n'.join(cleaned_lines)
multiline_string = """Hello, World!
This is a test.
Goodbye, World!
"""
cleaned_string = remove_newlines_from_multiline_string(multiline_string)
print(repr(cleaned_string)) # 输出: 'Hello, World!\nThis is a test.\nGoodbye, World!'
在上面的示例中,splitlines()
方法会将多行字符串拆分成一个行列表,然后使用列表推导式[line.rstrip('\n') for line in lines]
删除每行末尾的换行符,最后将处理后的行重新组合成一个字符串。
八、总结
在本文中,我们介绍了多种删除Python字符串末尾换行符的方法,包括使用rstrip()
方法、切片操作、正则表达式、自定义函数、处理文件中的换行符、使用字符串库的strip()
方法和处理多行字符串等。每种方法都有其适用的场景和优缺点,开发者可以根据具体需求选择合适的方法。
删除字符串末尾的换行符是Python编程中常见的任务,掌握这些方法将帮助你更高效地处理文本数据。
相关问答FAQs:
如何在Python中删除字符串末尾的换行符?
在Python中,可以使用rstrip()
方法来删除字符串末尾的换行符。这个方法会移除字符串右侧的空白字符,包括换行符。例如:
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.rstrip()
print(cleaned_string) # 输出: Hello, World!
这样就可以有效去除字符串末尾的换行符。
是否可以使用正则表达式删除换行符?
确实可以。使用re
模块中的sub()
函数,您可以通过正则表达式删除字符串末尾的换行符。示例如下:
import re
string_with_newline = "Hello, World!\n"
cleaned_string = re.sub(r'\n$', '', string_with_newline)
print(cleaned_string) # 输出: Hello, World!
这种方法在处理复杂字符串时特别有用。
在处理文件时,如何删除每行末尾的换行符?
读取文件时,可以通过遍历每一行并使用rstrip()
方法来删除换行符。示例代码如下:
with open('example.txt', 'r') as file:
lines = [line.rstrip() for line in file]
这样,您可以在保持文件内容的同时,去除每行末尾的换行符。
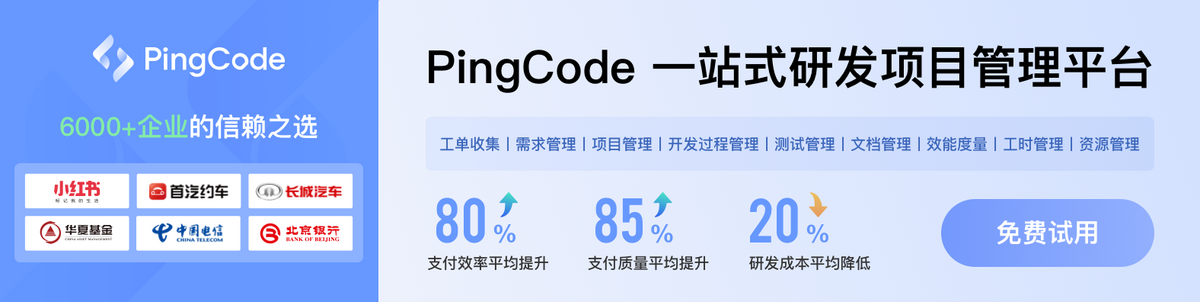