使用Python实现一个定时器,可以通过以下几种方式:使用time.sleep()、threading.Timer、sched模块。本文将详细介绍这几种方法,并重点介绍使用threading.Timer来实现定时器的方式。
一、使用time.sleep()
time.sleep()
是Python中最简单的实现定时器的方法,它可以让程序暂停指定的时间。
import time
def timer_function():
print("Timer finished!")
print("Starting timer for 5 seconds...")
time.sleep(5)
timer_function()
在上面的代码中,我们使用time.sleep(5)
让程序暂停5秒,然后调用timer_function()
函数。
二、使用threading.Timer
threading.Timer
是一个更高级的定时器实现方法,它允许我们在后台执行定时任务。
1、基本用法
import threading
def timer_function():
print("Timer finished!")
Set up a timer that will call the function after 5 seconds
timer = threading.Timer(5.0, timer_function)
print("Starting timer for 5 seconds...")
timer.start()
在上面的代码中,我们创建了一个threading.Timer
对象,该对象将在5秒后调用timer_function()
函数。
2、取消定时器
我们还可以在定时器触发之前取消它。
import threading
def timer_function():
print("Timer finished!")
Set up a timer that will call the function after 5 seconds
timer = threading.Timer(5.0, timer_function)
print("Starting timer for 5 seconds...")
timer.start()
Cancel the timer before it finishes
timer.cancel()
print("Timer cancelled!")
在上面的代码中,我们在定时器触发之前调用timer.cancel()
方法取消定时器。
三、使用sched模块
sched
模块提供了一个通用的事件调度器,可以用来实现定时器。
1、基本用法
import sched
import time
def timer_function():
print("Timer finished!")
scheduler = sched.scheduler(time.time, time.sleep)
scheduler.enter(5, 1, timer_function)
print("Starting timer for 5 seconds...")
scheduler.run()
在上面的代码中,我们使用sched.scheduler
创建一个调度器,并使用enter
方法安排一个5秒后的事件。
2、取消事件
我们还可以在事件触发之前取消它。
import sched
import time
def timer_function():
print("Timer finished!")
scheduler = sched.scheduler(time.time, time.sleep)
event = scheduler.enter(5, 1, timer_function)
print("Starting timer for 5 seconds...")
scheduler.cancel(event)
print("Timer cancelled!")
在上面的代码中,我们在事件触发之前调用scheduler.cancel(event)
方法取消事件。
四、使用 asyncio
asyncio
是Python 3.5引入的标准库,它提供了一种更加现代的异步编程方式,可以用来实现定时器。
1、基本用法
import asyncio
async def timer_function():
print("Timer finished!")
async def main():
print("Starting timer for 5 seconds...")
await asyncio.sleep(5)
await timer_function()
asyncio.run(main())
在上面的代码中,我们使用asyncio.sleep(5)
暂停5秒,然后调用timer_function()
函数。
2、取消任务
我们还可以在任务完成之前取消它。
import asyncio
async def timer_function():
print("Timer finished!")
async def main():
task = asyncio.create_task(timer_function())
print("Starting timer for 5 seconds...")
await asyncio.sleep(5)
task.cancel()
print("Timer cancelled!")
asyncio.run(main())
在上面的代码中,我们在任务完成之前调用task.cancel()
方法取消任务。
五、 使用APScheduler库
APScheduler
是一个强大的调度库,可以用来实现复杂的定时任务。它支持多种调度方式,包括固定时间间隔、指定时间点等。
1、安装APScheduler
首先,我们需要安装APScheduler
库:
pip install apscheduler
2、基本用法
from apscheduler.schedulers.background import BackgroundScheduler
import time
def timer_function():
print("Timer finished!")
scheduler = BackgroundScheduler()
scheduler.add_job(timer_function, 'interval', seconds=5)
print("Starting timer for 5 seconds...")
scheduler.start()
Keep the script running
try:
while True:
time.sleep(2)
except (KeyboardInterrupt, SystemExit):
scheduler.shutdown()
在上面的代码中,我们使用APScheduler
创建了一个后台调度器,并安排每隔5秒调用一次timer_function()
函数。
六、 使用 signal
signal
模块提供了一种基于信号的定时器实现方法,适用于Unix系统。
1、基本用法
import signal
import time
def timer_function(signum, frame):
print("Timer finished!")
signal.signal(signal.SIGALRM, timer_function)
signal.alarm(5)
print("Starting timer for 5 seconds...")
Keep the script running
time.sleep(6)
在上面的代码中,我们使用signal.alarm(5)
安排一个5秒后的信号,并在信号处理函数中调用timer_function()
函数。
七、 使用 Timer 类
我们还可以自定义一个Timer
类来实现定时器。
1、基本用法
import time
import threading
class Timer:
def __init__(self, interval, function):
self.interval = interval
self.function = function
self.thread = threading.Thread(target=self.run)
self.thread.daemon = True
def start(self):
self.thread.start()
def run(self):
time.sleep(self.interval)
self.function()
def timer_function():
print("Timer finished!")
timer = Timer(5, timer_function)
print("Starting timer for 5 seconds...")
timer.start()
Keep the script running
time.sleep(6)
在上面的代码中,我们自定义了一个Timer
类,并在run
方法中实现了定时逻辑。
总结:本文介绍了多种使用Python实现定时器的方法,包括time.sleep()
、threading.Timer
、sched
模块、asyncio
、APScheduler
库、signal
模块和自定义Timer
类。每种方法都有其适用的场景和优缺点,读者可以根据实际需求选择合适的方法来实现定时器。
相关问答FAQs:
如何在Python中创建一个简单的定时器?
要创建一个简单的定时器,可以使用threading
模块中的Timer
类。这个类允许你设置一个延迟时间,到达后执行指定的函数。示例代码如下:
import time
from threading import Timer
def hello():
print("Hello, world!")
# 创建一个定时器,延迟5秒后执行hello函数
t = Timer(5, hello)
t.start()
这个代码将在5秒后打印“Hello, world!”。
Python定时器可以用于哪些实际应用场景?
Python定时器可以广泛应用于多种场景。例如,定时任务的调度、周期性数据抓取、定时发送提醒消息等。在自动化测试中,定时器也可以用来控制测试执行的时间间隔,以确保系统在特定时间内的响应。
如何取消一个正在运行的定时器?
如果你需要在定时器执行之前取消它,可以调用定时器对象的cancel()
方法。以下是一个示例:
import time
from threading import Timer
def hello():
print("Hello, world!")
t = Timer(5, hello)
t.start()
# 在3秒后取消定时器
time.sleep(3)
t.cancel()
print("定时器已取消")
在这个例子中,定时器将在3秒后被取消,因此不会执行hello
函数。
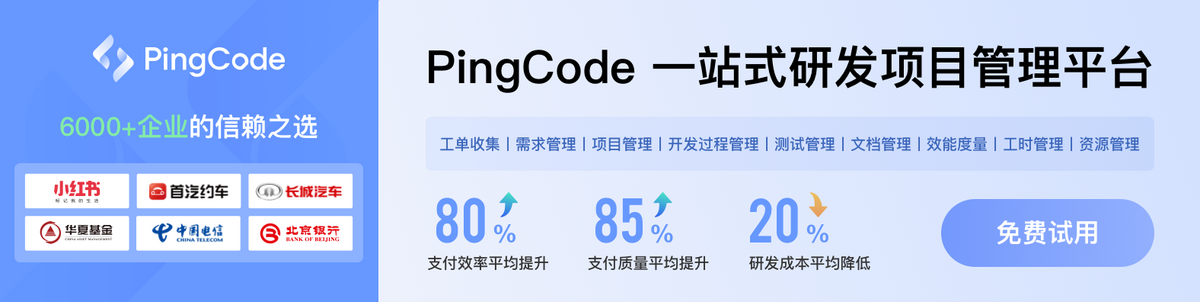