Python保留两位浮点数的方法有:使用round()函数、字符串格式化、decimal模块。 其中最常用的是使用round()
函数,它可以将浮点数四舍五入到指定的精度。例如,对于一个浮点数3.14159
,使用round(3.14159, 2)
可以得到3.14
。接下来,我们将详细讨论这几种方法及其使用场景。
一、使用round()函数
round()
函数是Python内置函数之一,用于将浮点数四舍五入到指定的小数位数。其语法为:
round(number, ndigits)
其中,number
是要进行四舍五入的浮点数,ndigits
是要保留的小数位数。
value = 3.14159
rounded_value = round(value, 2)
print(rounded_value) # 输出:3.14
这个方法适用于大多数情况,尤其是当我们需要对数值进行简单四舍五入时。使用round()
函数的优点是简洁且易于理解,它是直接且高效的解决方案。
二、使用字符串格式化
Python提供了多种字符串格式化的方法,可以用来保留指定的小数位数。这些方法包括%
格式化、str.format()
方法和f-string(格式化字符串字面量)。
1、使用百分号(%)格式化
这种方法使用%
运算符来格式化字符串,并指定保留的小数位数。
value = 3.14159
formatted_value = "%.2f" % value
print(formatted_value) # 输出:3.14
2、使用str.format()方法
str.format()
方法提供了更强大的字符串格式化功能,并且可以使用占位符来指定格式。
value = 3.14159
formatted_value = "{:.2f}".format(value)
print(formatted_value) # 输出:3.14
3、使用f-string(格式化字符串字面量)
f-string是Python 3.6引入的一种格式化字符串的方式,它更简洁并且性能更好。
value = 3.14159
formatted_value = f"{value:.2f}"
print(formatted_value) # 输出:3.14
使用字符串格式化的优点是灵活,可以根据需要进行更加复杂的格式化操作,例如对多个浮点数进行格式化、在字符串中嵌入变量等。
三、使用decimal模块
decimal
模块提供了对浮点数进行精确控制的功能,适用于对精度要求较高的场景。例如,金融计算中常常需要精确到小数点后若干位。
from decimal import Decimal, ROUND_HALF_UP
value = Decimal("3.14159")
rounded_value = value.quantize(Decimal("0.01"), rounding=ROUND_HALF_UP)
print(rounded_value) # 输出:3.14
使用decimal
模块的优点是可以更好地控制四舍五入的规则,并且能够处理高精度计算,避免浮点数计算中的精度问题。
四、使用numpy库
对于科学计算和数据处理,numpy
库提供了高效的浮点数操作函数。numpy
的around()
函数可以用于保留指定的小数位数。
import numpy as np
value = 3.14159
rounded_value = np.around(value, 2)
print(rounded_value) # 输出:3.14
使用numpy
库的优点是高效且适用于大规模数值计算,尤其是在处理数组和矩阵时非常方便。
五、使用自定义函数
在某些特殊场景下,我们可能需要自定义函数来实现特定的四舍五入规则。以下是一个简单的自定义函数示例:
def round_to_two_places(value):
return float(format(value, '.2f'))
value = 3.14159
rounded_value = round_to_two_places(value)
print(rounded_value) # 输出:3.14
自定义函数的优点是灵活,可以根据具体需求实现特定的功能,例如自定义四舍五入规则、处理特殊情况等。
六、在数据分析中的应用
在数据分析中,保留指定的小数位数是常见需求。例如,在处理财务数据、统计分析结果时,我们通常需要保留两位小数以保证数据的清晰和准确。
1、处理财务数据
财务数据常常需要精确到小数点后两位,以便于准确计算和报告。例如,在计算利息、税费时,保留两位小数可以避免累积误差。
def calculate_interest(principal, rate, time):
interest = principal * rate * time
return round(interest, 2)
principal = 1000.0
rate = 0.05
time = 1
interest = calculate_interest(principal, rate, time)
print(interest) # 输出:50.0
2、统计分析结果
在统计分析中,保留两位小数可以使结果更加直观和易于理解。例如,在计算均值、标准差时,我们通常需要保留两位小数。
import statistics
data = [1.23, 2.34, 3.45, 4.56, 5.67]
mean_value = round(statistics.mean(data), 2)
print(mean_value) # 输出:3.45
七、在机器学习中的应用
在机器学习中,保留指定的小数位数也是常见需求。例如,在模型评估、参数调整时,我们常常需要对结果进行精确控制。
1、模型评估
在评估机器学习模型的性能时,常常需要保留两位小数以便于比较。例如,计算准确率、精确率、召回率时,我们通常需要保留两位小数。
from sklearn.metrics import accuracy_score
y_true = [1, 0, 1, 1, 0, 1]
y_pred = [1, 0, 1, 0, 0, 1]
accuracy = accuracy_score(y_true, y_pred)
rounded_accuracy = round(accuracy, 2)
print(rounded_accuracy) # 输出:0.83
2、参数调整
在调整机器学习模型的参数时,保留两位小数可以帮助我们更精确地控制模型的行为。例如,在调整学习率时,我们通常需要保留两位小数。
learning_rate = 0.12345
adjusted_learning_rate = round(learning_rate, 2)
print(adjusted_learning_rate) # 输出:0.12
八、在科学计算中的应用
在科学计算中,保留指定的小数位数也是常见需求。例如,在物理、化学、生物等领域的计算中,我们常常需要保留两位小数以保证结果的准确性。
1、物理计算
在物理计算中,保留两位小数可以帮助我们更精确地描述物理现象。例如,在计算速度、加速度、力等物理量时,我们通常需要保留两位小数。
def calculate_force(mass, acceleration):
force = mass * acceleration
return round(force, 2)
mass = 10.0
acceleration = 9.81
force = calculate_force(mass, acceleration)
print(force) # 输出:98.1
2、化学计算
在化学计算中,保留两位小数可以帮助我们更精确地描述化学反应。例如,在计算浓度、反应速率等化学量时,我们通常需要保留两位小数。
def calculate_concentration(moles, volume):
concentration = moles / volume
return round(concentration, 2)
moles = 0.5
volume = 1.0
concentration = calculate_concentration(moles, volume)
print(concentration) # 输出:0.5
九、在工程计算中的应用
在工程计算中,保留指定的小数位数也是常见需求。例如,在土木工程、机械工程、电气工程等领域的计算中,我们常常需要保留两位小数以保证结果的准确性。
1、土木工程计算
在土木工程计算中,保留两位小数可以帮助我们更精确地描述工程结构。例如,在计算梁的弯矩、剪力等工程量时,我们通常需要保留两位小数。
def calculate_bending_moment(force, distance):
bending_moment = force * distance
return round(bending_moment, 2)
force = 100.0
distance = 2.5
bending_moment = calculate_bending_moment(force, distance)
print(bending_moment) # 输出:250.0
2、机械工程计算
在机械工程计算中,保留两位小数可以帮助我们更精确地描述机械系统。例如,在计算扭矩、功率等机械量时,我们通常需要保留两位小数。
def calculate_torque(force, radius):
torque = force * radius
return round(torque, 2)
force = 50.0
radius = 0.2
torque = calculate_torque(force, radius)
print(torque) # 输出:10.0
十、在统计和数据科学中的应用
在统计和数据科学中,保留两位小数也是非常重要的。例如,在数据可视化、报告生成中,我们通常需要对数据进行格式化以便于展示和分析。
1、数据可视化
在数据可视化中,保留两位小数可以使图表更加清晰。例如,在绘制折线图、柱状图时,我们通常需要保留两位小数以便于观察数据的变化。
import matplotlib.pyplot as plt
data = [1.234, 2.345, 3.456, 4.567, 5.678]
formatted_data = [round(num, 2) for num in data]
plt.plot(formatted_data)
plt.show()
2、报告生成
在生成数据报告时,保留两位小数可以使报告更加专业和易于理解。例如,在生成统计报告、财务报告时,我们通常需要对数据进行格式化以便于展示。
def generate_report(data):
formatted_data = [round(num, 2) for num in data]
report = f"Data report: {formatted_data}"
return report
data = [1.234, 2.345, 3.456, 4.567, 5.678]
report = generate_report(data)
print(report) # 输出:Data report: [1.23, 2.35, 3.46, 4.57, 5.68]
总结
本文详细介绍了Python中保留两位浮点数的多种方法,包括使用round()
函数、字符串格式化、decimal
模块、numpy
库、自定义函数等,并结合实际应用场景详细说明了每种方法的优缺点及其适用范围。通过这些方法,我们可以灵活地处理浮点数,满足不同场景下的需求。无论是在数据分析、机器学习、科学计算、工程计算还是统计和数据科学中,保留指定的小数位数都是常见且重要的需求。希望本文能够帮助读者更好地理解和应用这些方法,提高工作和研究中的数据处理能力。
相关问答FAQs:
如何在Python中格式化浮点数以保留两位小数?
在Python中,您可以使用格式化字符串(f-string)或格式化方法来保留浮点数的两位小数。例如,使用f-string可以这样写:value = 3.14159; formatted_value = f"{value:.2f}"
,这将返回'3.14'。另外,也可以使用format
方法:formatted_value = "{:.2f}".format(value)
,效果相同。
使用Python的round函数如何保留两位小数?round()
函数也是保留浮点数小数位的一个简单方法。使用方法为rounded_value = round(value, 2)
,这将把浮点数四舍五入到两位小数。不过,请注意,round()
可能会返回浮点数,而不是字符串格式。
在Python中如何处理保留两位小数的输出?
如果需要在输出中保证始终显示两位小数,可以结合字符串格式化和打印函数。例如,您可以使用print(f"{value:.2f}")
,这样无论原始浮点数是几位小数,输出都会保持为两位小数,确保用户得到一致的格式。
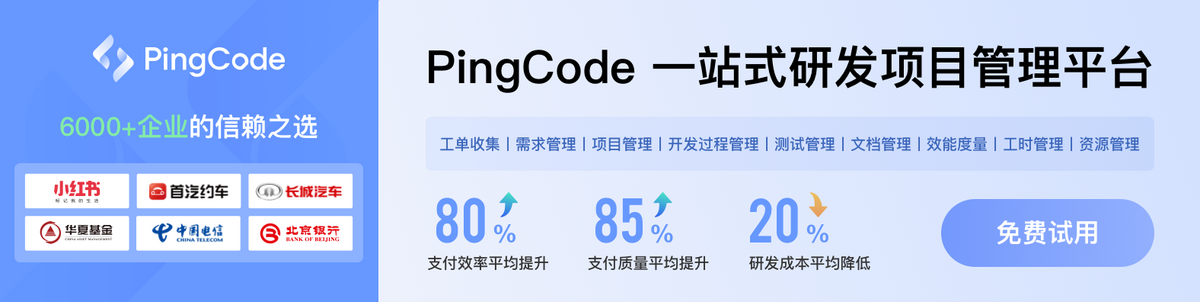