用Python修改指定行的方法有很多,例如:读取文件内容到列表,修改列表中指定行的内容,再将列表写回文件。 下面将详细介绍一种常见的方法,即使用文件读写操作来修改指定行的内容。
一、读取文件内容到列表
首先,我们需要将文件的内容读取到一个列表中,以便后续的修改操作。Python 提供了多种读取文件的方法,最常用的是使用 open()
函数配合 readlines()
方法。
def read_file_to_list(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
二、修改列表中指定行的内容
读取文件内容到列表之后,我们可以通过列表的索引来修改指定行的内容。下面的代码展示了如何修改列表中的某一行:
def modify_line_in_list(lines, line_number, new_content):
if 0 <= line_number < len(lines):
lines[line_number] = new_content + '\n'
else:
raise IndexError("Line number out of range")
三、将修改后的列表写回文件
修改完列表中的内容后,我们需要将列表重新写回文件。可以使用 writelines()
方法将列表中的所有行写入文件。
def write_list_to_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
四、完整代码示例
下面是将上述三个步骤整合到一起的完整代码示例:
def read_file_to_list(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
def modify_line_in_list(lines, line_number, new_content):
if 0 <= line_number < len(lines):
lines[line_number] = new_content + '\n'
else:
raise IndexError("Line number out of range")
def write_list_to_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
def modify_file_line(file_path, line_number, new_content):
lines = read_file_to_list(file_path)
modify_line_in_list(lines, line_number, new_content)
write_list_to_file(file_path, lines)
示例用法
file_path = 'example.txt'
line_number = 2
new_content = 'This is the new content for line 3'
modify_file_line(file_path, line_number, new_content)
五、处理异常情况
在实际应用中,我们还需要考虑一些异常情况,例如文件不存在、文件权限不足等。这些情况可以通过捕获异常来处理。
def modify_file_line(file_path, line_number, new_content):
try:
lines = read_file_to_list(file_path)
modify_line_in_list(lines, line_number, new_content)
write_list_to_file(file_path, lines)
except FileNotFoundError:
print(f"Error: The file {file_path} does not exist.")
except PermissionError:
print(f"Error: Permission denied when accessing the file {file_path}.")
except IndexError as e:
print(f"Error: {e}")
示例用法
file_path = 'example.txt'
line_number = 2
new_content = 'This is the new content for line 3'
modify_file_line(file_path, line_number, new_content)
六、更多高级操作
除了基本的文件读写操作外,Python 还提供了一些高级文件处理方法,例如使用 with open()
语句实现文件的上下文管理、使用 shutil
模块进行文件的复制和移动等。
1、使用 with open()
上下文管理
使用 with open()
语句可以自动管理文件的打开和关闭,避免忘记关闭文件导致资源泄漏的问题。
def read_file_to_list(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
def write_list_to_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
2、使用 shutil
模块进行文件操作
shutil
模块提供了高级的文件操作方法,例如复制文件、移动文件等。
import shutil
复制文件
shutil.copy('source.txt', 'destination.txt')
移动文件
shutil.move('source.txt', 'destination.txt')
七、结论
通过本文的介绍,我们学习了如何使用 Python 修改文件中指定行的内容。主要步骤包括读取文件内容到列表、修改列表中指定行的内容、将列表重新写回文件。并且,我们还介绍了处理异常情况和一些高级的文件操作方法。希望这些内容能够帮助你在实际项目中更好地处理文件操作问题。
继续学习和实践,你将会发现 Python 在文件处理方面的强大和灵活。
相关问答FAQs:
如何在Python中读取特定行的数据?
在Python中,可以使用文件的readlines()
方法读取所有行,然后根据索引访问特定行。例如,使用with open('文件名.txt', 'r') as file:
打开文件后,可以通过lines = file.readlines()
读取所有行,接着使用lines[行号]
访问特定行。
如何在Python中修改文件的特定行?
要修改文件中的特定行,首先读取所有行并存储在一个列表中,接着对该列表进行修改,最后将修改后的内容写回文件。例如,可以使用with open('文件名.txt', 'w') as file:
打开文件并写入更新后的行,确保文件的内容被正确替换。
有没有简单的方式在Python中使用pandas修改特定行?
使用pandas库可以更方便地处理数据。首先,通过pd.read_csv('文件名.csv')
读取数据,然后使用dataframe.loc[行索引]
来修改特定行的值。修改完成后,可以使用dataframe.to_csv('文件名.csv', index=False)
将更改写回CSV文件。
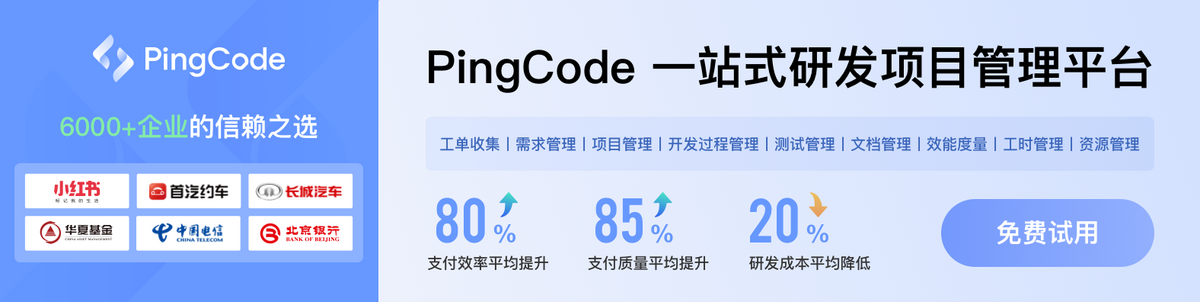