要将字节流输出到文件中,你可以使用Python的内置函数和库,例如open
函数、write
方法。首先,使用open
函数以二进制写入模式打开文件,然后使用文件对象的write
方法将字节流写入文件。确保正确关闭文件,以避免数据丢失。下面将详细描述如何实现这一过程。
一、打开文件
在Python中,你可以使用open
函数打开文件。open
函数的第一个参数是文件的路径,第二个参数是文件打开模式。要以二进制写入模式打开文件,使用'wb'
模式。wb
表示写入二进制文件。
file_path = 'example.bin'
file = open(file_path, 'wb')
二、写入字节流
接下来,使用文件对象的write
方法将字节流写入文件。字节流可以来自各种来源,例如网络数据、内存中的数据等。以下示例显示了如何将字节流写入文件:
byte_stream = b'Hello, world!' # 这是一个字节流
file.write(byte_stream)
三、关闭文件
写入完成后,关闭文件以确保数据被正确写入磁盘。你可以使用文件对象的close
方法来完成此操作:
file.close()
四、使用with
语句
在Python中,推荐使用with
语句来处理文件操作。with
语句确保文件在块结束时自动关闭,即使发生异常。以下是一个使用with
语句的示例:
file_path = 'example.bin'
byte_stream = b'Hello, world!'
with open(file_path, 'wb') as file:
file.write(byte_stream)
通过使用with
语句,你可以避免显式地调用close
方法,并确保文件在所有情况下都能正确关闭。
五、从网络获取字节流并写入文件
在实际应用中,字节流可能来自网络。例如,使用requests
库从URL下载文件,并将其写入本地文件:
import requests
url = 'https://example.com/somefile.zip'
response = requests.get(url)
file_path = 'downloaded_file.zip'
with open(file_path, 'wb') as file:
file.write(response.content)
这个示例演示了如何使用requests
库从URL获取字节流,并将其写入本地文件。
六、从内存中的数据写入文件
有时,字节流可能已经在内存中存储,例如在处理图像或音频数据时。以下示例演示了如何将内存中的字节流写入文件:
byte_stream = b'\x89PNG\r\n\x1a\n\x00\x00\x00\rIHDR\x00\x00\x00\x10\x00\x00\x00\x10\x08\x06\x00\x00\x00\x1f\xf3\xff\xa6\x00\x00\x00\x04gAMA\x00\x00\xb1\x8f\x0b\xfc\x61\x05\x00\x00\x00\x19tEXtSoftware\x00Adobe ImageReadyq\xc9e<\x00\x00\x00\x89IDATx\xdab\xfc\xff\xff?\x03\x35\x00\x00\x00\xff\xff\x03\x00\x00\x00\x00IEND\xaeB`\x82' # PNG图像字节流
file_path = 'image.png'
with open(file_path, 'wb') as file:
file.write(byte_stream)
该示例演示了如何将内存中的PNG图像字节流写入文件。
七、从标准输入读取字节流并写入文件
在某些情况下,你可能需要从标准输入读取字节流,并将其写入文件。以下示例演示了如何实现这一点:
import sys
file_path = 'output.bin'
with open(file_path, 'wb') as file:
byte_stream = sys.stdin.buffer.read()
file.write(byte_stream)
在这个示例中,字节流从标准输入读取,并写入文件output.bin
。
八、使用BytesIO对象
有时,你可能会使用io.BytesIO
对象来处理字节流。BytesIO
对象可以像文件一样操作。以下示例演示了如何将BytesIO
对象中的字节流写入文件:
import io
byte_stream = b'Hello, BytesIO!'
bytes_io = io.BytesIO(byte_stream)
file_path = 'bytes_io_output.bin'
with open(file_path, 'wb') as file:
file.write(bytes_io.getvalue())
这个示例演示了如何将BytesIO
对象中的字节流写入文件。
九、处理大文件
在处理大文件时,你可能需要逐块读取和写入数据,而不是一次性将整个字节流读取到内存中。以下示例演示了如何使用块读取和写入大文件:
chunk_size = 1024 # 每次读取和写入1KB
source_file_path = 'large_input_file.bin'
destination_file_path = 'large_output_file.bin'
with open(source_file_path, 'rb') as source_file, open(destination_file_path, 'wb') as destination_file:
while True:
chunk = source_file.read(chunk_size)
if not chunk:
break
destination_file.write(chunk)
这个示例演示了如何逐块读取和写入大文件,以节省内存。
十、使用shutil
库shutil
库提供了高级文件操作功能,包括复制文件和目录。以下示例演示了如何使用shutil.copyfileobj
函数将字节流从一个文件复制到另一个文件:
import shutil
source_file_path = 'input.bin'
destination_file_path = 'output.bin'
with open(source_file_path, 'rb') as source_file, open(destination_file_path, 'wb') as destination_file:
shutil.copyfileobj(source_file, destination_file)
这个示例演示了如何使用shutil.copyfileobj
函数将字节流从一个文件复制到另一个文件。
十一、使用numpy
库
如果你处理的是数值数据,例如图像或科学计算数据,numpy
库可以帮助你更高效地处理字节流。以下示例演示了如何使用numpy
库将数组数据写入文件:
import numpy as np
data = np.array([1, 2, 3, 4, 5], dtype=np.uint8)
file_path = 'numpy_output.bin'
with open(file_path, 'wb') as file:
file.write(data.tobytes())
这个示例演示了如何将numpy
数组数据写入文件。
十二、使用pickle
库pickle
库允许你将Python对象序列化为字节流,并将其写入文件。以下示例演示了如何使用pickle
库将字典对象写入文件:
import pickle
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
file_path = 'pickle_output.bin'
with open(file_path, 'wb') as file:
pickle.dump(data, file)
这个示例演示了如何使用pickle
库将字典对象序列化为字节流,并写入文件。
十三、使用struct
库struct
库允许你将数据打包成字节流,并将其写入文件。以下示例演示了如何使用struct
库将整数和字符串数据写入文件:
import struct
data = struct.pack('i10s', 12345, b'HelloWorld')
file_path = 'struct_output.bin'
with open(file_path, 'wb') as file:
file.write(data)
这个示例演示了如何使用struct
库将整数和字符串数据打包成字节流,并写入文件。
十四、处理多线程环境
在多线程环境中,你可能需要确保文件操作是线程安全的。以下示例演示了如何使用threading
库在多线程环境中写入文件:
import threading
file_path = 'thread_output.bin'
byte_stream = b'Hello, threading!'
def write_to_file():
with open(file_path, 'ab') as file: # 以追加模式打开文件
file.write(byte_stream)
threads = []
for _ in range(5):
thread = threading.Thread(target=write_to_file)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
这个示例演示了如何在多线程环境中写入文件,确保文件操作是线程安全的。
总结
通过这些示例,你可以看到如何在不同情况下将字节流写入文件。无论字节流来自网络、内存、标准输入,还是需要逐块处理、并发处理,Python提供了丰富的工具和库来帮助你实现这一目标。使用这些方法,你可以高效地处理和存储字节流数据。
相关问答FAQs:
如何在Python中将字节流写入文件?
在Python中,可以使用内置的open()
函数以二进制写入模式打开文件,并使用write()
方法将字节流写入文件。具体示例如下:
byte_data = b'Hello, World!' # 示例字节流
with open('output_file.bin', 'wb') as file:
file.write(byte_data)
此代码会在当前目录下创建一个名为output_file.bin
的文件,并将字节流内容写入其中。
在写入字节流时应该注意哪些事项?
在写入字节流之前,确保以二进制模式打开文件(例如,'wb'),以避免数据损坏。此外,确保字节流的内容是有效的字节数据,而不是字符串或其他类型的数据。可以使用bytes
或bytearray
类型来确保数据格式正确。
如何从文件中读取字节流?
读取字节流的过程与写入类似。使用open()
函数以二进制读取模式打开文件,并使用read()
方法读取字节流。例如:
with open('output_file.bin', 'rb') as file:
byte_data = file.read()
上述代码会从output_file.bin
文件中读取所有字节并将其存储在byte_data
变量中。
有什么方法可以检查文件的写入结果?
可以通过读取文件并打印其内容来验证写入结果。打开文件并读取字节流后,可以使用print()
函数查看内容,或者通过某种方式将其转换为可读的格式(如解码为字符串)进行检查。
with open('output_file.bin', 'rb') as file:
byte_data = file.read()
print(byte_data.decode('utf-8')) # 假设字节流是UTF-8编码
这样可以确认文件中存储的字节流是否与原始数据相匹配。
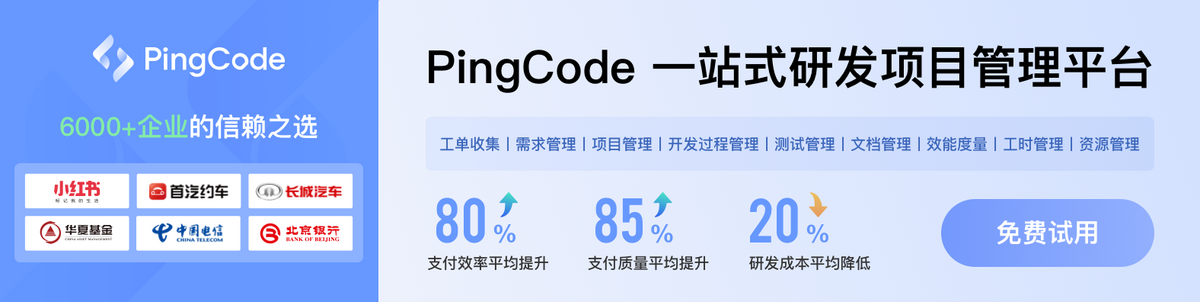