要将视频嵌入二维码中,可以通过将视频的URL或视频文件的路径编码到二维码中。
在本文中,我们将详细介绍如何使用Python实现这一目标,具体操作步骤如下:生成二维码、嵌入视频URL、解码二维码并播放视频。
一、二维码生成
首先,我们需要生成一个二维码。二维码可以通过各种库生成,其中 qrcode
库是一个常用的选择。我们将使用这个库来生成二维码。
安装必要的库
pip install qrcode[pil] opencv-python
生成二维码
我们将使用URL作为输入,这样二维码可以在扫描时打开链接到视频。
import qrcode
def generate_qr_code(data, file_path):
# Create instance of QRCode
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
# Add data to the instance
qr.add_data(data)
qr.make(fit=True)
# Create an image from the QR Code instance
img = qr.make_image(fill_color="black", back_color="white")
# Save the image to a file
img.save(file_path)
Example URL for a video
video_url = "https://www.example.com/video.mp4"
generate_qr_code(video_url, "video_qr.png")
在上述代码中,我们创建了一个二维码生成器,然后将视频的URL添加到二维码中,并将其保存为图像文件。
二、解码二维码
我们使用 OpenCV 库来解码二维码并获取其中的视频URL。
解码二维码
import cv2
def decode_qr_code(file_path):
# Read the image file
img = cv2.imread(file_path)
# Initialize the QRCode detector
detector = cv2.QRCodeDetector()
# Detect and decode the QR code
data, vertices_array, binary_qrcode = detector.detectAndDecode(img)
if vertices_array is not None:
print(f"QRCode data: {data}")
return data
else:
print("QRCode not detected")
return None
Decode the QR code
decoded_data = decode_qr_code("video_qr.png")
在上述代码中,我们使用 OpenCV 的 QRCodeDetector 类来解码二维码,并提取其中包含的视频URL。
三、播放视频
一旦我们解码了二维码并提取了视频URL,可以通过打开该URL来播放视频。这里我们将使用 webbrowser 模块来打开视频URL。
播放视频
import webbrowser
def play_video(video_url):
webbrowser.open(video_url)
Play the video from the decoded URL
if decoded_data:
play_video(decoded_data)
这段代码将打开默认的网络浏览器,并导航到视频的URL,从而播放视频。
四、完整代码示例
将上述所有步骤整合成一个完整的代码示例:
import qrcode
import cv2
import webbrowser
def generate_qr_code(data, file_path):
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="white")
img.save(file_path)
def decode_qr_code(file_path):
img = cv2.imread(file_path)
detector = cv2.QRCodeDetector()
data, vertices_array, binary_qrcode = detector.detectAndDecode(img)
if vertices_array is not None:
print(f"QRCode data: {data}")
return data
else:
print("QRCode not detected")
return None
def play_video(video_url):
webbrowser.open(video_url)
Generate the QR code
video_url = "https://www.example.com/video.mp4"
generate_qr_code(video_url, "video_qr.png")
Decode the QR code and play the video
decoded_data = decode_qr_code("video_qr.png")
if decoded_data:
play_video(decoded_data)
通过以上步骤,我们详细介绍了如何使用 Python 将视频嵌入二维码中,并通过扫描二维码来播放视频。希望这篇文章对你有所帮助!
相关问答FAQs:
如何在Python中生成包含视频的二维码?
要在Python中创建一个二维码,您可以使用qrcode
库。首先,您需要将视频上传到一个可以公开访问的网络位置,比如YouTube或其他视频托管平台。接着,获取视频的链接并使用qrcode
库生成二维码。例如:
import qrcode
# 视频链接
video_url = 'https://www.example.com/your_video_link'
qr = qrcode.QRCode(version=1, error_correction=qrcode.constants.ERROR_CORRECT_L, box_size=10, border=4)
qr.add_data(video_url)
qr.make(fit=True)
img = qr.make_image(fill='black', back_color='white')
img.save('video_qr.png')
这样,您就可以生成一个二维码,扫描后会直接链接到您的视频。
如何确保生成的二维码能被手机扫描?
在生成二维码时,确保选择合适的版本和纠错级别。版本越高,二维码能承载的信息量越大;而纠错级别越高,即使二维码部分损坏也能被识别。一般情况下,选择ERROR_CORRECT_L
就足够满足大多数用户的需求。
二维码的尺寸和清晰度对扫描有影响吗?
是的,二维码的尺寸和打印或显示的清晰度都会影响扫描效果。确保二维码在适当的尺寸下生成,避免过小,通常建议至少保持在2×2厘米以上。如果是用于打印,确保打印的清晰度高,避免模糊,这样用户在扫描时能更容易识别。
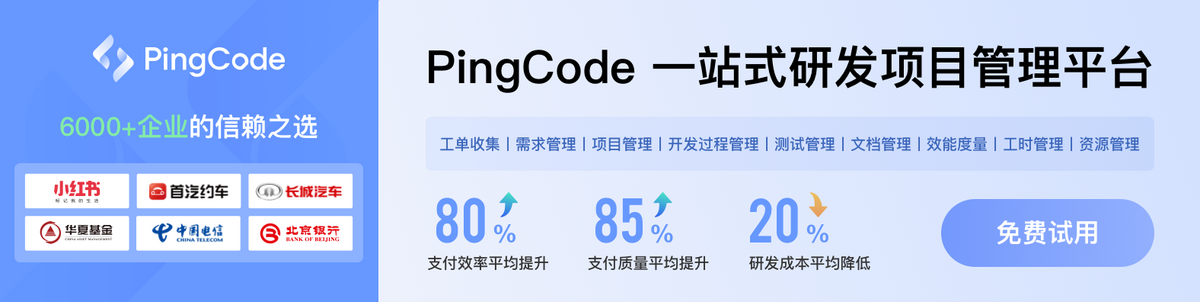