要用Python来统计分数段人数,可以使用以下几个核心步骤:收集数据、定义分数段、遍历数据并统计、输出结果。下面将详细描述如何完成这些步骤。
一、收集数据
首先,需要收集要统计的分数数据。这些数据可以来源于不同的地方,比如从文件中读取、用户输入或者是直接在程序中定义一个分数列表。为了简化示例,我们可以先在程序中定义一个分数列表。
scores = [67, 89, 45, 90, 72, 88, 94, 56, 73, 81, 65, 77, 82, 92, 61, 70, 55, 40, 85, 95]
二、定义分数段
定义分数段是统计的关键部分,这里我们可以将分数段定义为:0-59, 60-69, 70-79, 80-89, 90-100。为了方便统计,我们可以使用一个字典来表示每个分数段。
score_ranges = {
"0-59": 0,
"60-69": 0,
"70-79": 0,
"80-89": 0,
"90-100": 0
}
三、遍历数据并统计
接下来,需要遍历分数列表,并根据每个分数所在的分数段,对应增加字典中的计数值。
for score in scores:
if score < 60:
score_ranges["0-59"] += 1
elif score < 70:
score_ranges["60-69"] += 1
elif score < 80:
score_ranges["70-79"] += 1
elif score < 90:
score_ranges["80-89"] += 1
else:
score_ranges["90-100"] += 1
四、输出结果
最后,将统计结果输出,展示每个分数段的人数。
for range_key, count in score_ranges.items():
print(f"Scores in range {range_key}: {count} students")
完整示例代码
# Sample scores list
scores = [67, 89, 45, 90, 72, 88, 94, 56, 73, 81, 65, 77, 82, 92, 61, 70, 55, 40, 85, 95]
Define score ranges
score_ranges = {
"0-59": 0,
"60-69": 0,
"70-79": 0,
"80-89": 0,
"90-100": 0
}
Count the number of scores in each range
for score in scores:
if score < 60:
score_ranges["0-59"] += 1
elif score < 70:
score_ranges["60-69"] += 1
elif score < 80:
score_ranges["70-79"] += 1
elif score < 90:
score_ranges["80-89"] += 1
else:
score_ranges["90-100"] += 1
Print the result
for range_key, count in score_ranges.items():
print(f"Scores in range {range_key}: {count} students")
进一步优化
上述代码已经能够实现基本的分数段统计功能,但在实际应用中,我们可能需要更多的功能和优化。例如:
- 处理动态输入:从文件或用户输入读取分数列表。
- 自定义分数段:允许用户自定义分数段。
- 可视化结果:使用图表或其他方式展示统计结果。
下面我们将进一步探讨这些优化。
一、处理动态输入
要从文件中读取分数列表,可以使用Python的文件操作功能。假设我们有一个文本文件scores.txt
,其中每行包含一个分数。
# Read scores from a file
def read_scores_from_file(filename):
scores = []
with open(filename, 'r') as file:
for line in file:
scores.append(int(line.strip()))
return scores
scores = read_scores_from_file('scores.txt')
或者,从用户输入读取分数列表。
# Read scores from user input
def read_scores_from_input():
scores = []
print("Enter scores (type 'done' to finish):")
while True:
score = input()
if score.lower() == 'done':
break
try:
scores.append(int(score))
except ValueError:
print("Invalid input. Please enter a valid score.")
return scores
scores = read_scores_from_input()
二、自定义分数段
可以允许用户自定义分数段,通过输入起始和结束分数来定义分数段。
# Define score ranges based on user input
def define_score_ranges():
ranges = {}
print("Enter score ranges (type 'done' to finish):")
while True:
range_input = input()
if range_input.lower() == 'done':
break
try:
range_start, range_end = map(int, range_input.split('-'))
ranges[f"{range_start}-{range_end}"] = 0
except ValueError:
print("Invalid input. Please enter a valid range in the format 'start-end'.")
return ranges
score_ranges = define_score_ranges()
三、可视化结果
使用Python的matplotlib
库,可以将统计结果以图表形式展示。
import matplotlib.pyplot as plt
Data for plotting
labels = list(score_ranges.keys())
counts = list(score_ranges.values())
Plotting the bar chart
plt.bar(labels, counts)
plt.xlabel('Score Ranges')
plt.ylabel('Number of Students')
plt.title('Score Distribution')
plt.show()
完整优化示例代码
import matplotlib.pyplot as plt
Function to read scores from a file
def read_scores_from_file(filename):
scores = []
with open(filename, 'r') as file:
for line in file:
scores.append(int(line.strip()))
return scores
Function to read scores from user input
def read_scores_from_input():
scores = []
print("Enter scores (type 'done' to finish):")
while True:
score = input()
if score.lower() == 'done':
break
try:
scores.append(int(score))
except ValueError:
print("Invalid input. Please enter a valid score.")
return scores
Function to define score ranges based on user input
def define_score_ranges():
ranges = {}
print("Enter score ranges (type 'done' to finish):")
while True:
range_input = input()
if range_input.lower() == 'done':
break
try:
range_start, range_end = map(int, range_input.split('-'))
ranges[f"{range_start}-{range_end}"] = 0
except ValueError:
print("Invalid input. Please enter a valid range in the format 'start-end'.")
return ranges
Function to count the number of scores in each range
def count_scores_in_ranges(scores, score_ranges):
for score in scores:
for range_key in score_ranges:
range_start, range_end = map(int, range_key.split('-'))
if range_start <= score <= range_end:
score_ranges[range_key] += 1
break
return score_ranges
Function to plot the results
def plot_results(score_ranges):
labels = list(score_ranges.keys())
counts = list(score_ranges.values())
plt.bar(labels, counts)
plt.xlabel('Score Ranges')
plt.ylabel('Number of Students')
plt.title('Score Distribution')
plt.show()
Main function
def main():
scores = read_scores_from_input() # or read_scores_from_file('scores.txt')
score_ranges = define_score_ranges()
score_ranges = count_scores_in_ranges(scores, score_ranges)
for range_key, count in score_ranges.items():
print(f"Scores in range {range_key}: {count} students")
plot_results(score_ranges)
Run the main function
if __name__ == "__main__":
main()
通过上述代码,你可以灵活地读取分数数据、自定义分数段,并使用图表展示统计结果。这样不仅提高了程序的实用性,还增强了用户体验。
相关问答FAQs:
如何使用Python读取成绩数据并进行统计?
使用Python读取成绩数据通常可以借助Pandas库。首先,确保你的数据以CSV或Excel文件格式存在。你可以使用pandas.read_csv()
或pandas.read_excel()
函数来导入数据。数据导入后,可以通过DataFrame
中的条件过滤来统计不同分数段的人数。例如,使用df[(df['score'] >= 60) & (df['score'] < 70)].count()
可以统计60到69分之间的人数。
如何定义分数段以便进行统计?
在统计分数段人数时,定义合理的分数段是非常重要的。你可以根据需求将分数划分为多个区间,例如:0-59、60-69、70-79、80-89、90-100等。使用Python中的numpy
库可以方便地创建这些区间,例如使用numpy.histogram()
函数来获取各分数段的频数分布。
如何可视化分数段统计结果?
为了更直观地展示分数段统计结果,可以使用Python的Matplotlib或Seaborn库进行可视化。你可以绘制柱状图或饼图,帮助更清晰地展示各分数段的人数分布。举个例子,使用plt.bar()
函数可以快速生成柱状图,展示各分数段的人数,让数据更加易于理解。
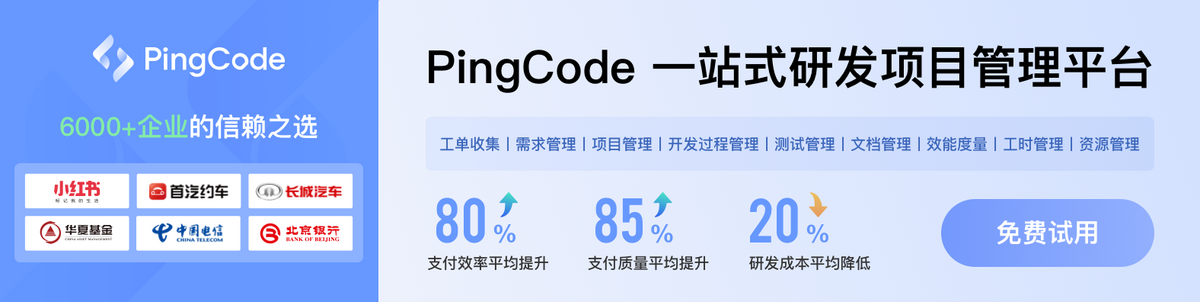