在Python中,实现运算符重载的方式是通过定义特殊方法(魔法方法),如__add__
, __sub__
, __mul__
等。运算符重载可以让自定义对象使用内置运算符操作,增强代码的可读性、简洁性、并提高操作的直观性。 例如,通过重载__add__
方法,可以让自定义类的对象直接使用+
运算符进行相加操作。
以下是详细描述如何在Python中实现运算符重载的步骤、方法和注意事项。
一、定义运算符重载方法
运算符重载通过定义特殊的方法来实现。这些方法是Python的内置方法,通常以双下划线开头和结尾。例如:
__add__(self, other)
用于重载加法运算符+
__sub__(self, other)
用于重载减法运算符-
__mul__(self, other)
用于重载乘法运算符*
__truediv__(self, other)
用于重载除法运算符/
__floordiv__(self, other)
用于重载整数除法运算符//
__mod__(self, other)
用于重载取模运算符%
__pow__(self, other)
用于重载幂运算符
二、示例:重载加法运算符
我们可以通过一个简单的示例来展示如何在Python中重载加法运算符。假设我们有一个表示二维向量的类Vector
,我们希望能够直接使用+
运算符来相加两个Vector
对象。
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
if isinstance(other, Vector):
return Vector(self.x + other.x, self.y + other.y)
return NotImplemented
def __str__(self):
return f"Vector({self.x}, {self.y})"
示例使用
v1 = Vector(2, 3)
v2 = Vector(4, 5)
v3 = v1 + v2
print(v3) # 输出: Vector(6, 8)
在上面的代码中,我们定义了一个Vector
类,并重载了__add__
方法来实现加法运算符的重载。当我们使用v1 + v2
时,实际上调用的是v1.__add__(v2)
方法,这个方法返回一个新的Vector
对象,其x
和y
属性分别是两个向量对应属性的和。
三、重载其他运算符
除了加法运算符,我们还可以重载其他运算符。例如:
1、重载减法运算符
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __sub__(self, other):
if isinstance(other, Vector):
return Vector(self.x - other.x, self.y - other.y)
return NotImplemented
def __str__(self):
return f"Vector({self.x}, {self.y})"
示例使用
v1 = Vector(5, 7)
v2 = Vector(3, 2)
v3 = v1 - v2
print(v3) # 输出: Vector(2, 5)
2、重载乘法运算符
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __mul__(self, scalar):
if isinstance(scalar, (int, float)):
return Vector(self.x * scalar, self.y * scalar)
return NotImplemented
def __str__(self):
return f"Vector({self.x}, {self.y})"
示例使用
v1 = Vector(2, 3)
v2 = v1 * 3
print(v2) # 输出: Vector(6, 9)
四、重载比较运算符
除了算术运算符,Python还允许我们重载比较运算符,例如==
, !=
, <
, >
, <=
, >=
。这些运算符对应的特殊方法分别是__eq__
, __ne__
, __lt__
, __gt__
, __le__
, __ge__
。
1、重载相等运算符
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __eq__(self, other):
if isinstance(other, Vector):
return self.x == other.x and self.y == other.y
return NotImplemented
示例使用
v1 = Vector(2, 3)
v2 = Vector(2, 3)
v3 = Vector(4, 5)
print(v1 == v2) # 输出: True
print(v1 == v3) # 输出: False
2、重载大于运算符
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __gt__(self, other):
if isinstance(other, Vector):
return (self.x <strong> 2 + self.y </strong> 2) > (other.x <strong> 2 + other.y </strong> 2)
return NotImplemented
示例使用
v1 = Vector(3, 4)
v2 = Vector(1, 1)
v3 = Vector(2, 2)
print(v1 > v2) # 输出: True
print(v1 > v3) # 输出: True
五、其他常用重载方法
除了上面提到的运算符,Python还提供了一些常用的重载方法,例如:
__str__(self)
:定义对象的字符串表示,通常用于print
函数。__repr__(self)
:定义对象的正式字符串表示,通常用于调试。__len__(self)
:定义对象的长度,通常用于内置len
函数。__getitem__(self, key)
:定义对象的索引访问,通常用于[]
运算符。__setitem__(self, key, value)
:定义对象的索引赋值,通常用于[]
运算符。
1、重载字符串表示方法
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return f"Vector({self.x}, {self.y})"
def __repr__(self):
return f"Vector(x={self.x}, y={self.y})"
示例使用
v = Vector(2, 3)
print(str(v)) # 输出: Vector(2, 3)
print(repr(v)) # 输出: Vector(x=2, y=3)
2、重载长度方法
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __len__(self):
return 2
示例使用
v = Vector(2, 3)
print(len(v)) # 输出: 2
3、重载索引访问方法
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __getitem__(self, index):
if index == 0:
return self.x
elif index == 1:
return self.y
else:
raise IndexError("Index out of range")
def __setitem__(self, index, value):
if index == 0:
self.x = value
elif index == 1:
self.y = value
else:
raise IndexError("Index out of range")
示例使用
v = Vector(2, 3)
print(v[0]) # 输出: 2
print(v[1]) # 输出: 3
v[0] = 5
print(v[0]) # 输出: 5
六、注意事项
- 返回NotImplemented:当重载方法中遇到不支持的操作时,应该返回
NotImplemented
,而不是抛出异常。这样可以让其他操作符有机会处理该操作。 - 对称性:对于某些运算符(如加法和乘法),应该确保操作的对称性,即
a + b
和b + a
具有相同的效果。这可以通过重载相关的反向方法(如__radd__
,__rmul__
)来实现。 - 保持一致性:在重载运算符时,确保新定义的行为与运算符的预期行为一致。例如,加法运算符应该用于相加,而不是进行其他非相关操作。
通过以上的步骤和示例,我们可以在Python中实现运算符重载,从而使自定义对象能够使用内置运算符进行操作,提升代码的可读性和简洁性。
相关问答FAQs:
运算符重载在Python中有什么实际应用场景?
运算符重载在Python中可以用于自定义类的行为,使得自定义对象能够像内置数据类型一样使用运算符。例如,在实现一个复数类时,可以重载加法运算符,使得可以直接对两个复数对象进行相加。此外,运算符重载还可用于实现集合类、矩阵运算等场景,这样可以提升代码的可读性和简洁性。
如何在Python中重载特定的运算符?
在Python中,可以通过定义特殊方法来重载运算符。例如,重载加法运算符可以实现__add__
方法,重载减法运算符则可以实现__sub__
方法。通过在自定义类中实现这些方法,可以控制在使用这些运算符时所执行的具体操作。每个运算符都有对应的特殊方法,可以查阅Python文档获取详细信息。
重载运算符时需要注意哪些事项?
在重载运算符时,确保重载的行为符合运算符的语义非常重要。例如,重载加法运算符时,应该确保返回的结果是两个对象的合理组合。此外,要注意类型检查和异常处理,确保在操作不适用的类型时不会导致程序崩溃。良好的文档和注释也能帮助其他开发者理解自定义的运算符行为。
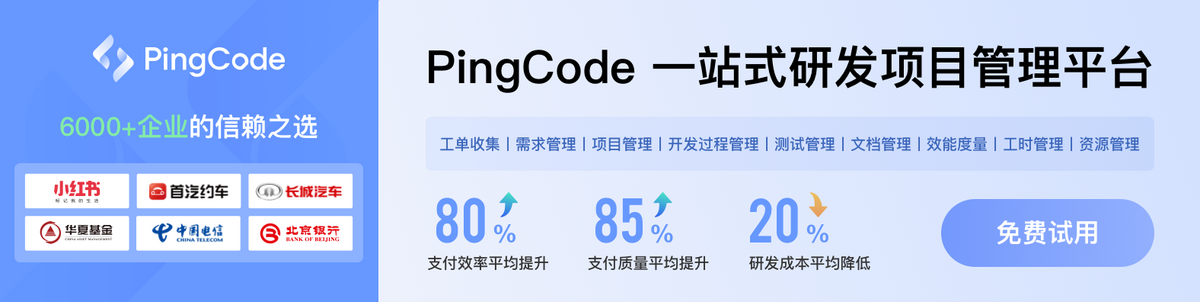