一、文本相似度分析是自然语言处理中的一种重要技术,在Python中可以使用多种方法进行文本相似度分析,如余弦相似度、Jaccard相似度和TF-IDF等。通过这些方法,能够量化两段文本之间的相似程度,从而实现文本分类、聚类等功能。余弦相似度是其中一种常用的方法,它通过计算两个向量之间的夹角余弦值来衡量文本的相似度。
余弦相似度的计算步骤如下:
- 对文本进行预处理(如去停用词、词干提取等)。
- 将文本向量化(如使用词频-逆文档频率(TF-IDF)方法)。
- 计算两个向量的余弦相似度。
通过这种方法,可以量化两段文本之间的相似程度,从而实现文本分类、聚类等功能。
二、文本预处理
在进行文本相似度分析之前,首先需要对文本进行预处理。文本预处理的步骤包括去停用词、词干提取、标点符号处理等。这些步骤能够帮助我们得到更干净、更规范的文本,从而提高文本相似度分析的准确性。
去停用词
停用词是指在文本中出现频率很高,但对文本主题贡献较小的词语,如“的”、“是”、“在”等。去除这些停用词可以减少噪音,提高文本相似度分析的准确性。在Python中,可以使用NLTK库中的停用词表来去除停用词。
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
words = text.split()
filtered_text = [word for word in words if word.lower() not in stop_words]
return ' '.join(filtered_text)
词干提取
词干提取是将单词还原为其基本形式的过程。例如,将“running”还原为“run”。词干提取可以减少词汇量,提高文本相似度分析的效果。在Python中,可以使用NLTK库中的PorterStemmer进行词干提取。
from nltk.stem import PorterStemmer
def stem_text(text):
stemmer = PorterStemmer()
words = text.split()
stemmed_text = [stemmer.stem(word) for word in words]
return ' '.join(stemmed_text)
标点符号处理
标点符号在文本相似度分析中通常被认为是噪音,因此需要将其去除。在Python中,可以使用正则表达式来去除标点符号。
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
三、文本向量化
文本向量化是将文本转换为数值向量的过程。常用的文本向量化方法有词袋模型(Bag of Words)和TF-IDF(词频-逆文档频率)等。TF-IDF是一种常用的文本向量化方法,它能够衡量单词在文档中的重要性。
from sklearn.feature_extraction.text import TfidfVectorizer
def vectorize_texts(texts):
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(texts)
return tfidf_matrix
四、计算余弦相似度
余弦相似度是一种常用的文本相似度计算方法,它通过计算两个向量之间的夹角余弦值来衡量文本的相似度。在Python中,可以使用sklearn库中的cosine_similarity函数来计算余弦相似度。
from sklearn.metrics.pairwise import cosine_similarity
def compute_cosine_similarity(tfidf_matrix):
cosine_sim = cosine_similarity(tfidf_matrix)
return cosine_sim
五、综合示例
下面是一个完整的示例,演示如何使用上述方法进行文本相似度分析:
import nltk
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
import re
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
nltk.download('stopwords')
def preprocess_text(text):
text = remove_punctuation(text)
text = remove_stopwords(text)
text = stem_text(text)
return text
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
words = text.split()
filtered_text = [word for word in words if word.lower() not in stop_words]
return ' '.join(filtered_text)
def stem_text(text):
stemmer = PorterStemmer()
words = text.split()
stemmed_text = [stemmer.stem(word) for word in words]
return ' '.join(stemmed_text)
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
def vectorize_texts(texts):
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(texts)
return tfidf_matrix
def compute_cosine_similarity(tfidf_matrix):
cosine_sim = cosine_similarity(tfidf_matrix)
return cosine_sim
texts = ["This is a sample text.", "This text is a different sample."]
preprocessed_texts = [preprocess_text(text) for text in texts]
tfidf_matrix = vectorize_texts(preprocessed_texts)
cosine_sim = compute_cosine_similarity(tfidf_matrix)
print("Cosine Similarity:\n", cosine_sim)
六、其他相似度计算方法
除了余弦相似度,还有其他一些常用的文本相似度计算方法,如Jaccard相似度和欧几里得距离等。
Jaccard相似度
Jaccard相似度是通过计算两个集合的交集与并集的比值来衡量相似度。在文本处理中,可以将文本表示为单词的集合,然后计算Jaccard相似度。
def jaccard_similarity(text1, text2):
words1 = set(text1.split())
words2 = set(text2.split())
intersection = words1.intersection(words2)
union = words1.union(words2)
return len(intersection) / len(union)
text1 = "This is a sample text."
text2 = "This text is a different sample."
print("Jaccard Similarity:", jaccard_similarity(text1, text2))
欧几里得距离
欧几里得距离是通过计算两个向量之间的距离来衡量相似度。距离越小,相似度越高。可以将文本表示为TF-IDF向量,然后计算欧几里得距离。
from sklearn.metrics.pairwise import euclidean_distances
def compute_euclidean_distance(tfidf_matrix):
euclidean_dist = euclidean_distances(tfidf_matrix)
return euclidean_dist
print("Euclidean Distance:\n", compute_euclidean_distance(tfidf_matrix))
七、文本相似度分析的应用
文本相似度分析在许多领域都有广泛的应用,如文本分类、聚类、推荐系统等。
文本分类
通过计算文本相似度,可以将文本分类到不同的类别中。例如,垃圾邮件检测可以通过计算邮件内容与垃圾邮件样本的相似度来实现。
文本聚类
文本聚类是将相似的文本聚集到同一个簇中。可以通过计算文本之间的相似度,然后使用聚类算法(如K-means)来实现文本聚类。
推荐系统
推荐系统可以根据用户的历史行为推荐相似的内容。例如,可以通过计算用户浏览过的文章与其他文章的相似度,推荐用户可能感兴趣的文章。
八、总结
文本相似度分析是自然语言处理中的一项重要技术。在Python中,可以使用多种方法进行文本相似度分析,如余弦相似度、Jaccard相似度和欧几里得距离等。通过对文本进行预处理、向量化,然后计算相似度,可以实现文本分类、聚类和推荐等功能。希望本文能够帮助你理解和掌握文本相似度分析的方法和应用。
相关问答FAQs:
如何在Python IDLE中使用文本相似度分析库?
在Python IDLE中,可以使用多种库来进行文本相似度分析,比如difflib
、sklearn
中的TfidfVectorizer
和cosine_similarity
等。首先,确保安装了相关库。接着,可以通过加载文本数据并使用这些库中的函数来计算文本之间的相似度。例如,使用difflib
的SequenceMatcher
类可以很方便地计算两个字符串之间的相似度评分。
文本相似度分析有什么实际应用场景?
文本相似度分析在多个领域有广泛应用。例如,在内容推荐系统中,可以根据用户的阅读历史推荐相似的文章;在抄袭检测中,可以通过比较文档之间的相似度来识别潜在的抄袭行为;在客户服务中,可以通过分析用户反馈与已知问题的相似度来提高响应效率。
如何提高文本相似度分析的准确性?
提高文本相似度分析的准确性可以通过多种方法实现。可以考虑使用更复杂的文本处理技术,比如自然语言处理(NLP)中的词嵌入(Word Embeddings)或深度学习模型。同时,数据预处理也至关重要,例如去除停用词、进行词干提取或使用句法分析来提取更有意义的特征,这些都能有效提高模型的性能。
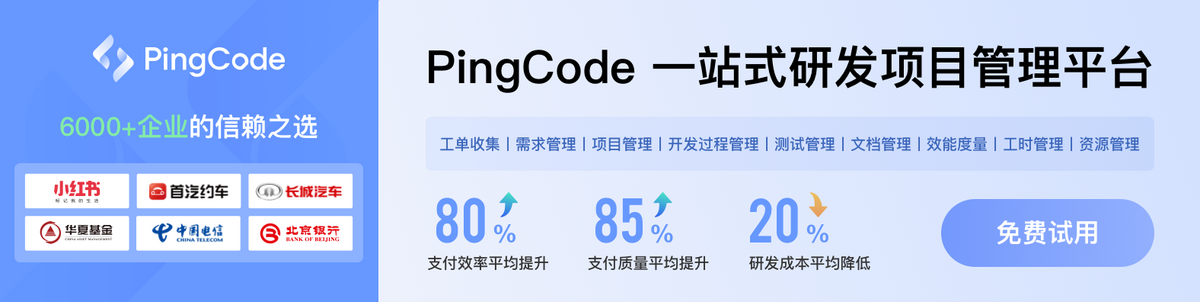