要查看函数获得的数据类型,可以使用内置的 type()
函数、使用 isinstance()
函数、利用 Python 的 inspect
模块、使用类型注解(Type Hinting)等方法。 其中,最常用且简单的方法是使用 type()
函数,这个函数可以直接告诉你变量或表达式的数据类型。下面将详细介绍这些方法及其使用场景。
一、使用 type()
函数
type()
函数是 Python 中最常用的查看数据类型的方法。它返回对象的类型,可以直接应用于任何 Python 对象。下面是一个例子:
def example_function():
return 10
result = example_function()
print(type(result)) # <class 'int'>
在这个例子中,type()
函数返回 int
,表示 result
是一个整数。
二、使用 isinstance()
函数
isinstance()
函数用于检查一个对象是否是指定类型的实例。这个函数需要两个参数:第一个是要检查的对象,第二个是类型或类型的元组。它返回一个布尔值。下面是一个例子:
def example_function():
return 10
result = example_function()
if isinstance(result, int):
print("The result is an integer.")
else:
print("The result is not an integer.")
在这个例子中,isinstance()
函数检查 result
是否是 int
类型。如果是,则输出 "The result is an integer."。
三、利用 Python 的 inspect
模块
inspect
模块提供了几个有用的函数来获取有关实时对象的信息。inspect.signature()
可以获取函数的参数和返回类型。下面是一个例子:
import inspect
def example_function() -> int:
return 10
sig = inspect.signature(example_function)
return_annotation = sig.return_annotation
print(return_annotation) # <class 'int'>
在这个例子中,inspect.signature()
函数获取了 example_function
的签名,sig.return_annotation
返回了该函数的返回类型。
四、使用类型注解(Type Hinting)
Python 3.5 引入了类型注解,可以在函数定义时指定参数和返回值的类型。这不仅提高了代码的可读性,还可以帮助 IDE 和类型检查工具进行静态类型检查。下面是一个例子:
def example_function() -> int:
return 10
result = example_function()
print(type(result)) # <class 'int'>
在这个例子中,类型注解 -> int
表示 example_function
返回一个整数。尽管类型注解不会在运行时强制执行,但它是一个很好的文档工具。
五、结合使用 type()
和类型注解
在实际项目中,我们可以结合使用 type()
和类型注解来确保函数的返回值符合预期。下面是一个例子:
def example_function() -> int:
return 10
result = example_function()
assert type(result) is int, "The function did not return an integer"
print("The function returned an integer.")
在这个例子中,使用类型注解来声明预期的返回类型,并使用 type()
函数和 assert
语句来验证返回值是否符合预期。
六、动态类型检查
在某些情况下,函数可能返回多种类型的值。我们可以编写一个辅助函数来动态检查返回值的类型。下面是一个例子:
def example_function(condition: bool):
if condition:
return 10
else:
return "Hello"
def check_return_type(value):
if isinstance(value, int):
print("The return type is an integer.")
elif isinstance(value, str):
print("The return type is a string.")
else:
print("The return type is unknown.")
result = example_function(True)
check_return_type(result) # The return type is an integer.
result = example_function(False)
check_return_type(result) # The return type is a string.
在这个例子中,example_function
函数根据条件返回整数或字符串。check_return_type
函数动态检查返回值的类型并输出相应的消息。
七、使用第三方库
在某些复杂情况下,可以使用第三方库(如 pydantic
)来进行更严格的类型检查和验证。pydantic
提供了数据验证和解析的功能,特别适用于数据密集型应用。下面是一个例子:
from pydantic import BaseModel
class ExampleModel(BaseModel):
value: int
def example_function() -> int:
return 10
result = example_function()
model = ExampleModel(value=result)
print(model) # value=10
在这个例子中,ExampleModel
使用 pydantic
来验证返回值是否为整数。如果 example_function
返回的值不是整数,pydantic
会抛出验证错误。
八、使用自定义类型检查器
在某些高级场景中,可以编写自定义类型检查器来验证函数的返回值。这通常涉及定义一个基类和一个验证函数。下面是一个例子:
class TypeChecker:
def __init__(self, expected_type):
self.expected_type = expected_type
def check(self, value):
if not isinstance(value, self.expected_type):
raise TypeError(f"Expected type {self.expected_type}, but got {type(value)}")
print(f"The value is of type {self.expected_type}.")
def example_function() -> int:
return 10
result = example_function()
checker = TypeChecker(int)
checker.check(result) # The value is of type <class 'int'>.
在这个例子中,TypeChecker
类用于检查值的类型。如果值的类型不符合预期,TypeChecker
会抛出 TypeError
。
九、总结
在 Python 中,查看函数返回值的数据类型有多种方法,包括使用内置的 type()
和 isinstance()
函数、利用 inspect
模块、使用类型注解和第三方库等。选择哪种方法取决于具体的使用场景和需求。在日常开发中,结合多种方法可以提高代码的可读性和健壮性。希望通过本文的介绍,读者能更好地理解和掌握查看函数返回值数据类型的方法。
相关问答FAQs:
如何在Python中检查一个函数返回的数据类型?
在Python中,可以使用内置的type()
函数来检查一个函数的返回值的数据类型。首先,调用该函数并将返回值传递给type()
,例如:
result = my_function()
print(type(result))
这将输出返回值的类型,如<class 'int'>
或<class 'str'>
等。
有没有其他方法可以确定数据类型?
除了使用type()
,还可以使用isinstance()
函数来判断一个对象是否属于特定类型。例如:
if isinstance(result, list):
print("返回值是一个列表")
这种方法允许进行更精细的类型检查,例如判断一个对象是否属于多个类型中的任何一个。
如何处理函数返回值为None的情况?
当函数可能返回None
时,检查数据类型时应考虑这一点。可以在调用type()
之前,先检查返回值是否为None
。这样可以避免在处理返回值时遇到错误:
result = my_function()
if result is not None:
print(type(result))
else:
print("返回值为None")
这种方式确保了在处理数据时的安全性和可靠性。
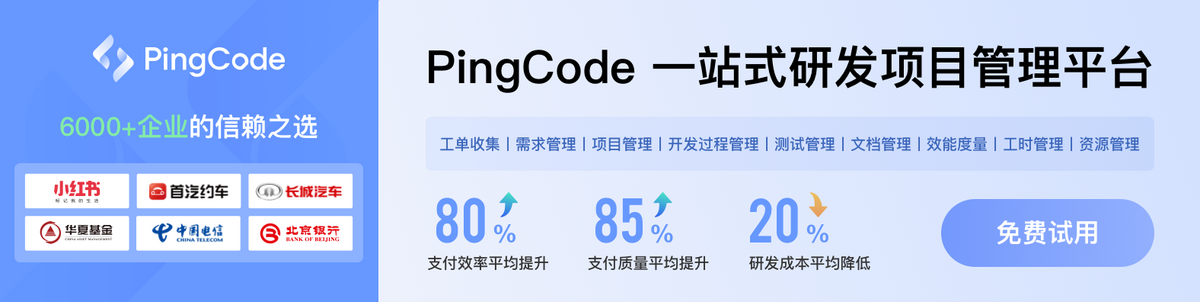