Python抓取网页数据处理超时的方法包括:设置合理的超时时间、使用重试机制、优化网络请求。其中,设置合理的超时时间是一个关键点,它可以防止程序因为等待响应而陷入无休止的阻塞状态。
为了更详细地描述这一点,设置合理的超时时间可以通过在发送请求时指定超时参数来实现。例如,在使用requests
库时,可以通过timeout
参数来设置超时时间。这样即使目标网站响应非常慢,程序也能在指定的时间内自动放弃请求并返回超时错误,从而避免程序卡住。以下是一个示例:
import requests
try:
response = requests.get('https://example.com', timeout=10) # 设置超时时间为10秒
response.raise_for_status()
except requests.exceptions.Timeout:
print('请求超时,请重试')
except requests.exceptions.RequestException as e:
print(f'请求发生错误: {e}')
一、设置合理的超时时间
合理设置超时时间可以有效防止程序因网络问题陷入长时间等待。通常情况下,超时时间不宜设置过短也不宜过长,应根据实际情况进行调整。对于大多数网络请求,5到10秒是一个比较合理的超时时间。
# 设置超时时间为5秒
response = requests.get('https://example.com', timeout=5)
这个超时时间是指连接(connect)和读取(read)的总时间,如果任何一个操作超过这个时间,都会引发Timeout
异常。
二、使用重试机制
在网络请求中,偶尔会遇到临时的网络问题或服务器负载过高导致的超时错误。对于这种情况,使用重试机制可以提高成功率。常见的重试库有retrying
和tenacity
,下面是使用tenacity
库的示例:
from tenacity import retry, stop_after_attempt, wait_fixed
import requests
@retry(stop=stop_after_attempt(3), wait=wait_fixed(2))
def fetch_data(url):
response = requests.get(url, timeout=10)
response.raise_for_status()
return response.text
try:
data = fetch_data('https://example.com')
except requests.exceptions.RequestException as e:
print(f'请求失败: {e}')
在这个例子中,fetch_data
函数会在失败后重试3次,每次重试之间等待2秒。如果在3次重试后仍然失败,才会抛出异常。
三、优化网络请求
优化网络请求可以提高抓取效率,减少超时发生的概率。以下是几种常见的优化方法:
- 使用连接池:使用连接池可以复用TCP连接,减少连接建立的开销,从而提高请求速度。
requests
库可以通过requests.Session
来实现连接池。
import requests
session = requests.Session()
response = session.get('https://example.com', timeout=10)
- 异步请求:使用异步请求可以同时发起多个请求,提高抓取效率。
aiohttp
是一个常用的异步HTTP客户端库,以下是一个示例:
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url, timeout=10) as response:
return await response.text()
async def main():
urls = ['https://example.com', 'https://example2.com']
tasks = [fetch(url) for url in urls]
responses = await asyncio.gather(*tasks)
for response in responses:
print(response)
asyncio.run(main())
- 处理代理和headers:使用代理可以避免因IP被封锁导致的超时问题,设置合适的headers可以模拟浏览器请求,提高成功率。
import requests
proxies = {
'http': 'http://10.10.10.10:8000',
'https': 'http://10.10.10.10:8000',
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get('https://example.com', proxies=proxies, headers=headers, timeout=10)
四、捕获和处理异常
在实际开发中,捕获和处理异常是确保程序健壮性的重要手段。除了Timeout
异常,还需要捕获其他可能的网络异常,并进行相应的处理。
import requests
try:
response = requests.get('https://example.com', timeout=10)
response.raise_for_status()
except requests.exceptions.Timeout:
print('请求超时,请重试')
except requests.exceptions.ConnectionError:
print('连接错误,请检查网络连接')
except requests.exceptions.HTTPError as err:
print(f'HTTP错误: {err.response.status_code}')
except requests.exceptions.RequestException as e:
print(f'请求发生错误: {e}')
通过这种方式,可以针对不同的异常类型进行不同的处理,提高程序的健壮性和容错能力。
五、日志记录和监控
在抓取网页数据时,记录日志和进行监控是非常重要的,可以帮助我们了解程序的运行状态,并在出现问题时及时发现和解决。logging
库是Python内置的日志库,可以方便地记录日志。
import logging
import requests
logging.basicConfig(level=logging.INFO)
def fetch_data(url):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
return response.text
except requests.exceptions.Timeout:
logging.error('请求超时: %s', url)
except requests.exceptions.RequestException as e:
logging.error('请求错误: %s', e)
data = fetch_data('https://example.com')
通过这种方式,可以将请求过程中的错误记录到日志中,方便后续排查和处理。
总之,处理Python抓取网页数据时的超时问题,需要综合运用设置合理的超时时间、使用重试机制、优化网络请求、捕获和处理异常、日志记录和监控等方法。这些方法可以有效提高抓取效率,减少超时发生的概率,提高程序的健壮性和稳定性。
相关问答FAQs:
如何判断在使用Python抓取网页数据时是否发生了超时?
在使用Python进行网页数据抓取时,可以通过捕获异常来判断是否发生了超时。例如,当使用requests库时,如果请求超时,可以捕获requests.exceptions.Timeout
异常。通过这种方式,您可以在程序中设置超时的时间限制,并在超时发生时进行相应的处理,例如重试请求或记录错误日志。
有哪些库可以帮助我处理Python网页抓取中的超时问题?
在Python中,除了requests库外,还可以使用其他库如Scrapy和Beautiful Soup。Scrapy本身就有内置的超时处理机制,允许您设置请求的超时时间。此外,使用aiohttp
库进行异步请求也可以更好地管理超时,通过设置timeout参数来控制请求的时间。
在抓取网页数据时,如何优化超时设置以提高成功率?
优化超时设置可以通过几个方法实现。首先,根据目标网站的响应时间合理设置超时值,通常可以在1-5秒之间进行调整。其次,结合重试机制,如果请求超时,可以设置一定次数的重试,以提高抓取成功的几率。同时,使用随机的时间间隔进行重试可以有效减少对目标网站的请求压力,从而降低被封禁的风险。
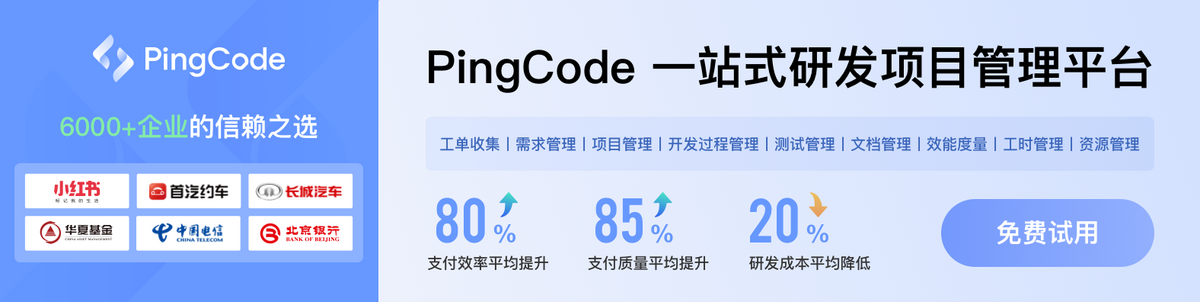