Python在Qt中画曲线图的方法有使用Qt自带的QPainter类、结合Matplotlib库、使用PyQtGraph库等。其中,使用PyQtGraph库是一种方便且高效的方法,因为它专门用于在Qt应用程序中进行实时数据绘制和快速图形绘制。接下来,我们将详细介绍如何使用PyQtGraph库在Qt应用程序中绘制曲线图。
PyQtGraph库的优势在于其高性能、易用性和与Qt的良好集成。接下来,将详细介绍如何在Python的Qt应用程序中使用PyQtGraph库绘制曲线图。
一、安装所需库
在开始之前,我们需要安装PyQt5和PyQtGraph库。可以使用以下命令进行安装:
pip install PyQt5 pyqtgraph
二、创建基本的Qt应用程序
首先,我们需要创建一个基本的Qt应用程序框架。下面是一个简单的示例代码:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQtGraph Plot Example")
self.setGeometry(100, 100, 800, 600)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
这段代码创建了一个基本的Qt应用程序窗口。
三、使用PyQtGraph绘制曲线图
接下来,我们将使用PyQtGraph库在Qt应用程序中绘制曲线图。
1、导入PyQtGraph库
首先,我们需要在代码中导入PyQtGraph库:
import pyqtgraph as pg
2、创建绘图窗口
在MainWindow
类中,我们将创建一个PyQtGraph的绘图窗口,并添加到Qt应用程序的主窗口中:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQtGraph Plot Example")
self.setGeometry(100, 100, 800, 600)
# 创建一个绘图窗口
self.plot_widget = pg.PlotWidget()
self.setCentralWidget(self.plot_widget)
3、绘制曲线图
接下来,我们将生成一些数据,并使用PyQtGraph绘制曲线图:
import numpy as np
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQtGraph Plot Example")
self.setGeometry(100, 100, 800, 600)
# 创建一个绘图窗口
self.plot_widget = pg.PlotWidget()
self.setCentralWidget(self.plot_widget)
# 生成数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
# 绘制曲线图
self.plot_widget.plot(x, y, pen=pg.mkPen(color='b', width=2))
在上述代码中,我们使用numpy
库生成了x和y数据,并使用plot_widget.plot
方法将数据绘制成曲线图。
四、添加更多的图形元素
除了基本的曲线图,我们还可以添加更多的图形元素,例如网格、标题、标签等。
1、添加网格
我们可以使用showGrid
方法添加网格:
self.plot_widget.showGrid(x=True, y=True)
2、添加标题和标签
我们可以使用setTitle
、setLabel
方法添加标题和标签:
self.plot_widget.setTitle("Sine Wave")
self.plot_widget.setLabel('left', 'Y-Axis')
self.plot_widget.setLabel('bottom', 'X-Axis')
完整的示例代码如下:
import sys
import numpy as np
from PyQt5.QtWidgets import QApplication, QMainWindow
import pyqtgraph as pg
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQtGraph Plot Example")
self.setGeometry(100, 100, 800, 600)
# 创建一个绘图窗口
self.plot_widget = pg.PlotWidget()
self.setCentralWidget(self.plot_widget)
# 生成数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
# 绘制曲线图
self.plot_widget.plot(x, y, pen=pg.mkPen(color='b', width=2))
# 添加网格
self.plot_widget.showGrid(x=True, y=True)
# 添加标题和标签
self.plot_widget.setTitle("Sine Wave")
self.plot_widget.setLabel('left', 'Y-Axis')
self.plot_widget.setLabel('bottom', 'X-Axis')
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
五、交互功能
PyQtGraph还支持多种交互功能,例如缩放、平移等。默认情况下,这些功能已经启用。用户可以使用鼠标滚轮进行缩放,点击并拖动进行平移。
1、添加图例
我们还可以为图形添加图例,以便更好地解释不同的曲线。可以使用addLegend
方法添加图例:
self.plot_widget.addLegend()
self.plot_widget.plot(x, y, pen=pg.mkPen(color='b', width=2), name="Sine Wave")
2、动态更新数据
我们还可以动态更新数据。例如,创建一个定时器,每隔一段时间更新一次数据:
from PyQt5.QtCore import QTimer
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQtGraph Plot Example")
self.setGeometry(100, 100, 800, 600)
# 创建一个绘图窗口
self.plot_widget = pg.PlotWidget()
self.setCentralWidget(self.plot_widget)
# 生成初始数据
self.x = np.linspace(0, 10, 100)
self.y = np.sin(self.x)
self.curve = self.plot_widget.plot(self.x, self.y, pen=pg.mkPen(color='b', width=2))
# 添加网格
self.plot_widget.showGrid(x=True, y=True)
# 添加标题和标签
self.plot_widget.setTitle("Sine Wave")
self.plot_widget.setLabel('left', 'Y-Axis')
self.plot_widget.setLabel('bottom', 'X-Axis')
# 创建定时器
self.timer = QTimer()
self.timer.timeout.connect(self.update_data)
self.timer.start(1000) # 每隔1000毫秒更新一次数据
def update_data(self):
# 更新数据
self.y = np.sin(self.x + np.random.rand())
self.curve.setData(self.x, self.y)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
在上述代码中,我们创建了一个定时器,每隔1000毫秒调用update_data
方法更新数据并重新绘制曲线。
六、总结
通过本文的介绍,我们学习了如何在Python的Qt应用程序中使用PyQtGraph库绘制曲线图。PyQtGraph库提供了高性能、易用且与Qt良好集成的绘图库,适用于实时数据绘制和快速图形绘制。我们还学习了如何添加网格、标题、标签、图例以及动态更新数据等功能。这些知识将帮助我们在实际项目中高效地进行数据可视化。
相关问答FAQs:
如何在Qt中使用Python绘制曲线图?
在Qt中绘制曲线图,可以使用PyQt或PySide结合Matplotlib库。首先,安装相关库(如PyQt5和Matplotlib),然后创建一个Qt窗口,并在窗口中嵌入Matplotlib的绘图部件。具体步骤包括初始化图形、设置数据点、绘制曲线以及将图形显示在Qt界面中。
哪些Python库适合在Qt中绘制曲线图?
在Qt中绘制曲线图时,最常用的库是Matplotlib和PyQtGraph。Matplotlib提供了灵活的绘图功能,适合复杂的图形绘制。PyQtGraph则是专为Qt设计的,提供高性能和实时更新的功能,适合需要频繁更新数据的应用。
如何在Qt应用程序中更新曲线图的数据?
要在Qt应用程序中动态更新曲线图,可以使用信号和槽机制。通过定时器或用户交互触发数据更新函数,重新绘制图形并更新显示。在Matplotlib中,可以使用set_data
方法来更新曲线数据,并调用draw
方法刷新图形。确保在更新过程中,界面保持响应,以提升用户体验。
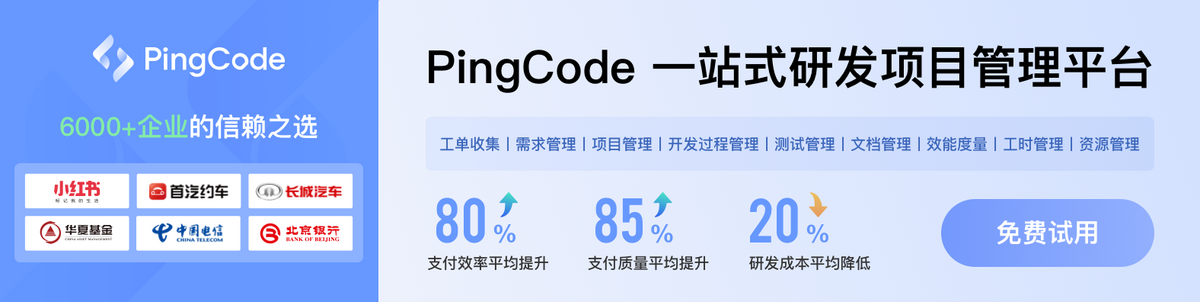