Python接收方法的返回值可以通过赋值给一个变量、直接使用返回值、或者作为参数传递给其他函数等多种方式。在编写Python代码时,函数或方法的返回值通常是通过return
语句来实现的。接收返回值后,可以立即对其进行处理、存储或传递给其他函数进行进一步操作。下面将详细介绍几种常见的接收和处理方法返回值的方式。
一、赋值给变量
最常见的接收方法返回值的方式是将其赋值给一个变量。这样可以方便地在后续代码中使用该变量。
def add(a, b):
return a + b
result = add(5, 3)
print(result) # 输出: 8
在上述示例中,add
方法的返回值被赋值给了变量result
,然后通过print
函数输出到控制台。
二、直接使用返回值
在某些情况下,可能不需要将返回值存储在变量中,而是直接在函数调用的位置使用返回值。
def multiply(a, b):
return a * b
print(multiply(4, 6)) # 输出: 24
在这个示例中,multiply
方法的返回值直接被传递给了print
函数,用于输出结果。
三、作为参数传递给其他函数
方法的返回值可以作为参数传递给其他函数,以实现更复杂的功能。
def square(x):
return x * x
def add(a, b):
return a + b
result = add(square(3), square(4))
print(result) # 输出: 25
在这个示例中,square
方法的返回值被传递给了add
方法,最终输出的结果是两个平方值的和。
四、处理多重返回值
Python函数可以返回多个值,接收时可以使用多个变量进行解包。
def divide(a, b):
quotient = a // b
remainder = a % b
return quotient, remainder
q, r = divide(10, 3)
print("Quotient:", q) # 输出: Quotient: 3
print("Remainder:", r) # 输出: Remainder: 1
在上述示例中,divide
方法返回两个值,接收时使用了两个变量q
和r
进行解包,从而分别接收商和余数。
五、使用返回值进行条件判断
函数的返回值可以用于条件判断,以决定接下来的操作。
def is_even(n):
return n % 2 == 0
if is_even(4):
print("4 is even")
else:
print("4 is odd")
在这个示例中,is_even
方法的返回值用于if
条件判断,根据返回值的布尔值决定执行哪个代码块。
六、存储返回值到数据结构中
返回值可以被存储到列表、字典等数据结构中,以便后续处理。
def generate_fibonacci(n):
fib_sequence = [0, 1]
while len(fib_sequence) < n:
fib_sequence.append(fib_sequence[-1] + fib_sequence[-2])
return fib_sequence
fib_list = generate_fibonacci(10)
print(fib_list) # 输出: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
在这个示例中,generate_fibonacci
方法生成的斐波那契数列被存储到列表fib_list
中,然后输出。
七、使用返回值进行错误处理
返回值可以用来指示函数执行的状态,从而进行相应的错误处理。
def safe_divide(a, b):
if b == 0:
return None
return a / b
result = safe_divide(10, 0)
if result is None:
print("Division by zero is not allowed")
else:
print("Result:", result)
在这个示例中,safe_divide
方法在检测到除数为零时返回None
,接收返回值后进行错误处理。
八、结合类和对象使用返回值
在面向对象编程中,方法返回值可以与类和对象结合使用,以实现更复杂的功能。
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
calc = Calculator()
sum_result = calc.add(10, 5)
difference_result = calc.subtract(10, 5)
print("Sum:", sum_result) # 输出: Sum: 15
print("Difference:", difference_result) # 输出: Difference: 5
在这个示例中,Calculator
类中的方法返回值被赋值给相应的变量,然后输出。
九、使用返回值进行迭代
返回值可以用于迭代操作,例如在for
循环中使用。
def get_numbers():
return [1, 2, 3, 4, 5]
for number in get_numbers():
print(number)
在这个示例中,get_numbers
方法返回一个列表,for
循环遍历该列表并输出每个元素。
十、结合函数式编程
返回值可以与函数式编程中的高阶函数结合使用,如map
、filter
和reduce
等。
def square(x):
return x * x
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(square, numbers))
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
在这个示例中,square
方法的返回值被map
函数用于生成新的列表-squared_numbers
。
十一、使用上下文管理器
函数的返回值可以用于上下文管理器,以确保资源的正确管理和释放。
class FileManager:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_value, traceback):
self.file.close()
with FileManager('test.txt', 'w') as f:
f.write('Hello, World!')
在这个示例中,FileManager
类的__enter__
方法返回文件对象,该返回值被用于with
语句块中进行文件操作。
十二、结合装饰器使用返回值
装饰器可以修改或增强函数的行为,返回值也可以在装饰器中进行处理。
def double_result(func):
def wrapper(*args, kwargs):
result = func(*args, kwargs)
return result * 2
return wrapper
@double_result
def add(a, b):
return a + b
print(add(5, 3)) # 输出: 16
在这个示例中,double_result
装饰器将被装饰函数的返回值加倍并返回,最终输出结果为16。
综上所述,Python接收方法的返回值有多种方式,具体使用哪种方式取决于实际需求和代码结构。通过灵活运用这些方法,可以编写出功能强大、易于维护的Python程序。
相关问答FAQs:
在Python中,如何定义一个返回值的方法?
在Python中,定义一个返回值的方法非常简单。使用def
关键字创建一个函数,并通过return
语句返回所需的值。例如:
def add(a, b):
return a + b
调用该函数时,可以接收返回值:
result = add(5, 3)
print(result) # 输出: 8
如何处理一个方法返回多个值?
Python的函数可以返回多个值,这些值会被打包成一个元组。可以通过逗号分隔多个返回值。例如:
def calculate(a, b):
return a + b, a - b
sum_result, diff_result = calculate(10, 5)
print(sum_result) # 输出: 15
print(diff_result) # 输出: 5
如果方法没有返回值,会发生什么?
如果一个方法没有使用return
语句,或者在return
后没有指定值,Python会默认返回None
。这意味着你可以调用该方法,但接收到的将是None
。例如:
def greet(name):
print(f"Hello, {name}")
result = greet("Alice")
print(result) # 输出: None
这种情况下,函数执行了预期的操作,但没有产生一个可用的返回值。
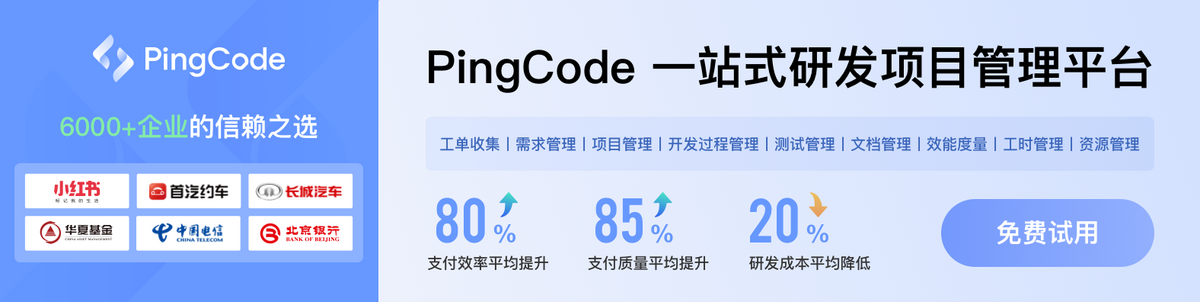