Python将浮点数写入文件的方法:使用内置的文件处理函数、格式化浮点数、使用json模块等方法。 本文将详细介绍这些方法,并给出相应的代码示例。
一、内置的文件处理函数
在Python中,最常用的方法之一是使用内置的文件处理函数来将浮点数写入文件。这个方法简单易用,可以满足大多数需求。以下是具体步骤:
- 打开文件
- 使用write()方法将浮点数写入文件
- 关闭文件
# 打开文件,使用'w'模式表示写入
with open('output.txt', 'w') as file:
# 要写入的浮点数
float_number = 3.14159
# 将浮点数转换为字符串并写入文件
file.write(str(float_number))
二、格式化浮点数
有时候,我们需要将浮点数格式化后再写入文件,比如限定小数点后的位数。Python提供了多种格式化浮点数的方法,如使用字符串的format()方法或f-strings。
# 使用format()方法
float_number = 3.14159
formatted_number = "{:.2f}".format(float_number)
with open('output.txt', 'w') as file:
file.write(formatted_number)
使用f-strings(Python 3.6及以上版本)
formatted_number = f"{float_number:.2f}"
with open('output.txt', 'w') as file:
file.write(formatted_number)
三、使用json模块
如果需要将多个浮点数或其他数据类型写入文件,json模块是一个不错的选择。它可以将Python对象序列化为JSON格式,并且提供了简单的文件读写操作。
import json
要写入的浮点数列表
float_numbers = [3.14159, 2.71828, 1.61803]
打开文件并写入浮点数列表
with open('output.json', 'w') as file:
json.dump(float_numbers, file)
四、使用pickle模块
pickle模块用于将Python对象序列化为二进制格式,并将其写入文件。它可以处理更多类型的数据,包括浮点数。
import pickle
要写入的浮点数列表
float_numbers = [3.14159, 2.71828, 1.61803]
打开文件并写入浮点数列表
with open('output.pkl', 'wb') as file:
pickle.dump(float_numbers, file)
五、使用pandas模块
如果你正在处理大量数据,尤其是数据分析相关的任务,pandas模块是一个强大的工具。它提供了多种文件读写功能,包括将浮点数写入CSV文件。
import pandas as pd
要写入的数据
data = {
'float_numbers': [3.14159, 2.71828, 1.61803]
}
创建DataFrame
df = pd.DataFrame(data)
将DataFrame写入CSV文件
df.to_csv('output.csv', index=False)
六、使用NumPy模块
NumPy是另一个用于科学计算的强大模块,常用于处理大规模数据。它提供了多种文件读写功能,包括将浮点数写入文本文件或二进制文件。
import numpy as np
要写入的浮点数数组
float_numbers = np.array([3.14159, 2.71828, 1.61803])
将浮点数数组写入文本文件
np.savetxt('output.txt', float_numbers)
将浮点数数组写入二进制文件
np.save('output.npy', float_numbers)
七、使用csv模块
csv模块提供了读写CSV文件的功能,可以方便地将浮点数写入CSV文件。
import csv
要写入的数据
float_numbers = [3.14159, 2.71828, 1.61803]
打开CSV文件并写入浮点数
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(float_numbers)
八、处理大文件和多线程
当处理非常大的文件时,可能需要优化文件写入速度或使用多线程。以下是一些建议:
- 批量写入:将浮点数批量写入文件,而不是每次写入一个数。这样可以减少磁盘I/O操作,提高写入速度。
- 多线程:使用多线程或多进程技术,同时写入多个文件或多个部分的数据,以提高效率。
以下是一个使用多线程的示例:
import threading
要写入的数据
float_numbers = [3.14159, 2.71828, 1.61803, 0.57721, 1.41421]
def write_to_file(file_name, data):
with open(file_name, 'w') as file:
for number in data:
file.write(f"{number}\n")
创建线程
thread1 = threading.Thread(target=write_to_file, args=('output1.txt', float_numbers[:3]))
thread2 = threading.Thread(target=write_to_file, args=('output2.txt', float_numbers[3:]))
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
九、处理科学记数法
在某些情况下,浮点数可能以科学记数法表示。Python提供了多种方法来处理科学记数法的浮点数。
# 使用科学记数法表示浮点数
float_number = 3.14159e2
将浮点数转换为字符串并写入文件
with open('output.txt', 'w') as file:
file.write(str(float_number))
十、错误处理
在文件写入过程中,可能会遇到各种错误,如文件权限问题、磁盘空间不足等。为了提高程序的健壮性,可以添加错误处理机制。
try:
# 打开文件
with open('output.txt', 'w') as file:
# 要写入的浮点数
float_number = 3.14159
# 将浮点数转换为字符串并写入文件
file.write(str(float_number))
except IOError as e:
print(f"文件写入失败:{e}")
总结
本文介绍了Python将浮点数写入文件的多种方法,包括使用内置的文件处理函数、格式化浮点数、使用json、pickle、pandas、NumPy、csv模块,以及处理大文件、多线程、科学记数法和错误处理等方面的内容。根据具体需求选择合适的方法,可以有效地将浮点数写入文件。
相关问答FAQs:
如何使用Python将浮点数保存为文本文件?
可以使用Python的内置函数open()
来创建或打开一个文件,并使用write()
方法将浮点数写入文件。确保将浮点数转换为字符串格式,例如使用str()
函数或格式化字符串。示例代码如下:
float_number = 3.14159
with open('output.txt', 'w') as file:
file.write(str(float_number))
这段代码会在当前目录下创建一个名为output.txt
的文件,并将浮点数写入其中。
如何将多个浮点数写入文件并保持格式?
要写入多个浮点数,可以使用循环遍历浮点数列表,并将每个数写入文件。可以使用格式化字符串来控制输出格式,例如保留小数点后两位。以下是一个示例:
float_numbers = [3.14, 2.71, 1.41]
with open('output.txt', 'w') as file:
for number in float_numbers:
file.write(f"{number:.2f}\n")
这将把每个浮点数写入新的一行,并保留两位小数。
如何在写入浮点数时处理可能的异常?
在写入浮点数时,可能会遇到文件无法打开或写入失败的情况。使用try...except
语句可以有效处理这些异常。例如:
float_number = 3.14159
try:
with open('output.txt', 'w') as file:
file.write(str(float_number))
except IOError as e:
print(f"写入文件时出错: {e}")
这种方法确保在出现错误时,程序不会崩溃,并能够输出有用的错误信息。
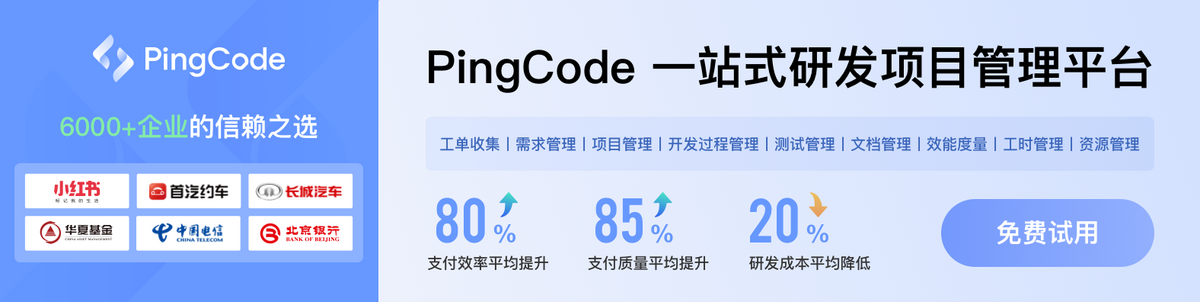