要判断Python栈中是否存在某元素,可以使用in
运算符、遍历栈、在特定方法中进行判断等方法,最常用的是直接使用in
运算符,因为它既简洁又高效。
直接使用in
运算符来判断栈中是否存在某元素是最常见的方法。这个方法非常简洁,只需一行代码即可完成判断。例如,对于一个栈stack
,可以使用element in stack
来检查element
是否在stack
中。如果栈中包含该元素,则返回True
,否则返回False
。这种方法不仅简洁,而且在Python内部进行了优化,效率较高。
下面将详细介绍多种方法来判断Python栈中是否存在某元素,并探讨每种方法的优缺点。
一、使用in
运算符
使用in
运算符是最简单的方法。假设我们有一个栈stack
,可以通过以下方式判断某元素是否在栈中:
stack = [1, 2, 3, 4, 5]
element = 3
if element in stack:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 简洁明了,只需一行代码。
- 内置优化,效率较高。
缺点:
- 对于非常大的栈,时间复杂度为O(n)。
二、遍历栈
遍历栈是一种较为基础的方法,通过遍历栈中的每一个元素来判断目标元素是否存在:
stack = [1, 2, 3, 4, 5]
element = 3
found = False
for item in stack:
if item == element:
found = True
break
if found:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 清晰易懂,适合初学者。
缺点:
- 代码较冗长。
- 时间复杂度为O(n),效率较低。
三、使用栈特定方法
如果栈是由某些特定的数据结构(如deque
)实现的,可以使用这些数据结构提供的特定方法来进行判断。例如,使用deque
实现栈:
from collections import deque
stack = deque([1, 2, 3, 4, 5])
element = 3
if element in stack:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 可以利用特定数据结构的优势。
缺点:
- 不同数据结构的方法可能不同,需要具体问题具体分析。
四、使用递归
递归是一种较为复杂但有趣的方法,通过递归调用函数来判断元素是否在栈中:
def is_in_stack(stack, element):
if not stack:
return False
if stack[-1] == element:
return True
return is_in_stack(stack[:-1], element)
stack = [1, 2, 3, 4, 5]
element = 3
if is_in_stack(stack, element):
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 递归思想优雅,代码简洁。
缺点:
- 递归深度受限,对于大栈可能会导致栈溢出。
- 性能较低,不适合大数据量。
五、使用集合(set)提高查找效率
如果栈中元素唯一,可以将栈转换为集合(set),利用集合的查找效率(O(1))来判断元素是否存在:
stack = [1, 2, 3, 4, 5]
element = 3
stack_set = set(stack)
if element in stack_set:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 查找效率高,时间复杂度为O(1)。
缺点:
- 需要额外的空间来存储集合。
- 只适用于元素唯一的情况。
六、使用二分查找(适用于有序栈)
如果栈是有序的,可以使用二分查找来提高查找效率,时间复杂度为O(log n):
import bisect
stack = [1, 2, 3, 4, 5]
element = 3
index = bisect.bisect_left(stack, element)
if index != len(stack) and stack[index] == element:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 查找效率高,时间复杂度为O(log n)。
缺点:
- 只适用于有序栈。
- 需要额外的库支持。
七、使用列表推导式
列表推导式是一种简洁的写法,可以在一行代码中完成判断:
stack = [1, 2, 3, 4, 5]
element = 3
found = any(item == element for item in stack)
if found:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 简洁明了,代码较短。
缺点:
- 对于非常大的栈,时间复杂度为O(n)。
八、使用生成器表达式
生成器表达式与列表推导式类似,但生成器表达式在大数据量时更节省内存:
stack = [1, 2, 3, 4, 5]
element = 3
found = any(item == element for item in stack)
if found:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 内存占用小,适合处理大数据量。
缺点:
- 时间复杂度为O(n)。
九、使用函数式编程
函数式编程风格可以利用filter
或map
函数来判断元素是否在栈中:
stack = [1, 2, 3, 4, 5]
element = 3
found = any(filter(lambda x: x == element, stack))
if found:
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 函数式编程风格简洁优雅。
缺点:
- 对于非常大的栈,时间复杂度为O(n)。
- 代码可读性较低。
十、使用自定义栈类
如果经常需要判断栈中是否存在某元素,可以自定义一个栈类,内部封装判断方法:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop() if self.items else None
def contains(self, element):
return element in self.items
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
element = 3
if stack.contains(element):
print(f"{element} is in the stack")
else:
print(f"{element} is not in the stack")
优点:
- 封装性好,代码可读性高。
缺点:
- 需要额外的类定义和维护。
总结
在Python中判断栈中是否存在某元素有多种方法,每种方法都有其优缺点。对于一般情况,直接使用in
运算符是最简洁高效的方法。但在特殊情况下,可以根据具体需求选择合适的方法,例如使用集合(set)提高查找效率、使用二分查找处理有序栈、使用生成器表达式节省内存等。总之,根据实际需求选择最合适的方法,才能在保证代码简洁的同时,提高程序的运行效率。
相关问答FAQs:
如何在Python中实现栈的基本操作以判断元素是否存在?
在Python中,可以使用列表来实现栈的基本操作,包括添加和删除元素。为了判断栈中是否存在某个元素,可以使用in
运算符。具体实现可以通过定义一个栈类,添加方法来检查元素的存在性。
使用Python的内置数据结构是否有更高效的查找方式?
如果你希望提高查找元素的效率,可以考虑使用集合(set)。集合在查找方面比列表更高效,因为它们的查找时间复杂度为O(1)。你可以将栈的元素存储在集合中,并利用集合的特性快速判断某个元素是否存在。
在多线程环境中,如何确保栈的安全性?
在多线程环境中,使用栈时需要注意线程安全问题。可以使用线程锁(如threading.Lock
)来确保在对栈进行操作时,其他线程无法同时访问。这样可以避免数据竞争和不一致的状态,确保栈操作的安全性。
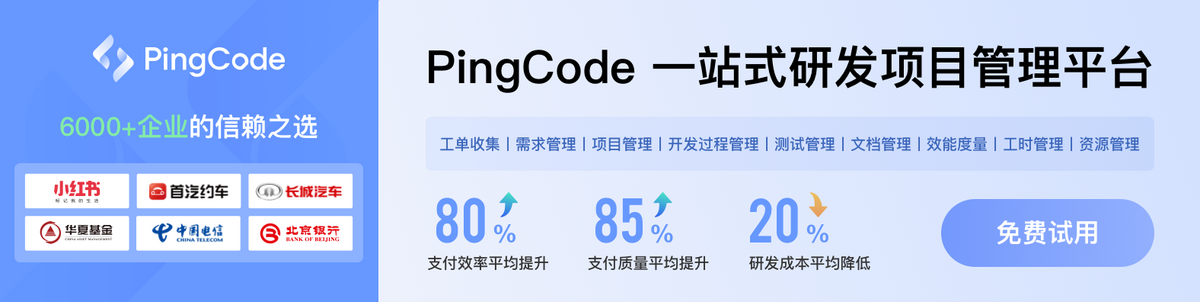