在Python中使用时间序列作为横坐标绘制图表,可以通过多种途径实现,主要工具包括Matplotlib、Pandas和Seaborn。这些库都提供了强大的功能来处理时间序列数据。以下是如何实现这一目标的几种方法:使用Pandas处理时间序列、使用Matplotlib绘制图表、结合Seaborn进行高级可视化。
详细描述:使用Pandas处理时间序列,Pandas是Python中处理数据的强大工具,特别适合处理时间序列数据。通过Pandas,你可以轻松地将时间数据转换为时间索引,并使用其内置功能进行数据操作和分析。以下是一个基本的示例,展示了如何使用Pandas处理时间序列数据,并结合Matplotlib绘制图表。
import pandas as pd
import matplotlib.pyplot as plt
创建示例数据
data = {'Date': pd.date_range(start='1/1/2020', periods=10, freq='D'),
'Value': range(10)}
创建DataFrame
df = pd.DataFrame(data)
将'Date'列设置为索引
df.set_index('Date', inplace=True)
绘制图表
df.plot()
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Time Series Plot')
plt.show()
一、使用Pandas处理时间序列
Pandas是一个功能强大的数据处理库,特别适用于时间序列数据的处理。通过Pandas,你可以轻松地将日期时间数据转换为时间索引,并进行各种操作和分析。以下是一些关键步骤和方法。
1.1 读取和处理时间序列数据
Pandas提供了多种方法来读取和处理时间序列数据。你可以从CSV文件、Excel文件或其他数据源中读取数据,然后将日期列转换为时间索引。
import pandas as pd
从CSV文件读取数据
df = pd.read_csv('data.csv', parse_dates=['Date'], index_col='Date')
查看数据类型
print(df.dtypes)
转换为时间索引
df.index = pd.to_datetime(df.index)
在上述代码中,我们使用parse_dates
参数将'Date'列解析为日期格式,并将其设置为索引。
1.2 时间序列数据的基本操作
Pandas提供了许多内置方法来操作时间序列数据。例如,你可以进行重采样、填充缺失值、滚动计算等操作。
# 重采样为月度数据
monthly_data = df.resample('M').mean()
填充缺失值
filled_data = df.fillna(method='ffill')
计算滚动平均值
rolling_mean = df.rolling(window=3).mean()
二、使用Matplotlib绘制图表
Matplotlib是Python中最常用的绘图库,适用于各种类型的图表绘制。通过将Pandas处理后的数据传递给Matplotlib,你可以轻松绘制时间序列图表。
2.1 基本绘图
你可以使用Pandas内置的绘图功能,或者直接使用Matplotlib绘制图表。
import matplotlib.pyplot as plt
使用Pandas内置的绘图功能
df.plot()
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Time Series Plot')
plt.show()
使用Matplotlib绘制图表
plt.figure(figsize=(10, 5))
plt.plot(df.index, df['Value'])
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Time Series Plot')
plt.show()
2.2 自定义图表
你可以通过Matplotlib的各种参数来自定义图表的外观,例如颜色、线型、标签等。
plt.figure(figsize=(10, 5))
plt.plot(df.index, df['Value'], color='blue', linestyle='--', marker='o')
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Customized Time Series Plot')
plt.grid(True)
plt.show()
三、结合Seaborn进行高级可视化
Seaborn是基于Matplotlib的高级可视化库,提供了更为美观和复杂的图表。通过Seaborn,你可以轻松实现高级可视化效果。
3.1 基本绘图
使用Seaborn绘制时间序列图表非常简单,只需传递Pandas DataFrame即可。
import seaborn as sns
使用Seaborn绘制基本图表
sns.lineplot(x=df.index, y='Value', data=df)
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Seaborn Time Series Plot')
plt.show()
3.2 高级可视化
Seaborn提供了许多高级绘图功能,例如添加置信区间、分面绘图等。
# 添加置信区间
sns.lineplot(x=df.index, y='Value', data=df, ci=95)
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Seaborn Time Series Plot with Confidence Interval')
plt.show()
分面绘图
g = sns.FacetGrid(df, col='Category', col_wrap=2)
g.map(sns.lineplot, 'Date', 'Value')
plt.show()
四、综合示例
以下是一个综合示例,展示了如何从数据读取、处理到可视化的完整流程。
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
读取数据
df = pd.read_csv('data.csv', parse_dates=['Date'], index_col='Date')
数据处理
monthly_data = df.resample('M').mean()
filled_data = df.fillna(method='ffill')
rolling_mean = df.rolling(window=3).mean()
基本绘图
plt.figure(figsize=(10, 5))
plt.plot(df.index, df['Value'], color='blue', linestyle='--', marker='o')
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Customized Time Series Plot')
plt.grid(True)
plt.show()
使用Seaborn进行高级可视化
sns.lineplot(x=df.index, y='Value', data=df, ci=95)
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Seaborn Time Series Plot with Confidence Interval')
plt.show()
通过以上方法,你可以在Python中轻松处理和可视化时间序列数据。无论是基本的绘图需求,还是高级的可视化效果,Pandas、Matplotlib和Seaborn都能满足你的需求。
相关问答FAQs:
在Python中,如何导入时间序列数据进行绘图?
要在Python中导入时间序列数据,通常可以使用Pandas库。首先,确保你有一个包含时间戳的CSV文件或其他数据格式。使用pd.read_csv()
读取数据,并将日期列解析为日期时间格式,使用pd.to_datetime()
函数。这样一来,你就可以轻松处理时间序列数据,并为绘图做好准备。
使用哪些库可以绘制时间序列图?
在Python中,最常用的绘图库包括Matplotlib和Seaborn。Matplotlib提供了灵活的绘图功能,适合创建基本的时间序列图,而Seaborn则提供了更高级的统计图形,能够快速生成更美观的时间序列图。此外,Plotly也是一个很好的选择,特别是用于交互式图表。
如何自定义时间序列图的格式和样式?
在Matplotlib中,可以通过多种方式自定义时间序列图的格式和样式。例如,你可以使用plt.xticks()
设置x轴的刻度,或使用plt.gca().xaxis.set_major_formatter()
自定义日期格式。同时,可以通过调整图形的颜色、线型和标签等属性,来增强图表的可读性和美观度,使其更符合你的需求。
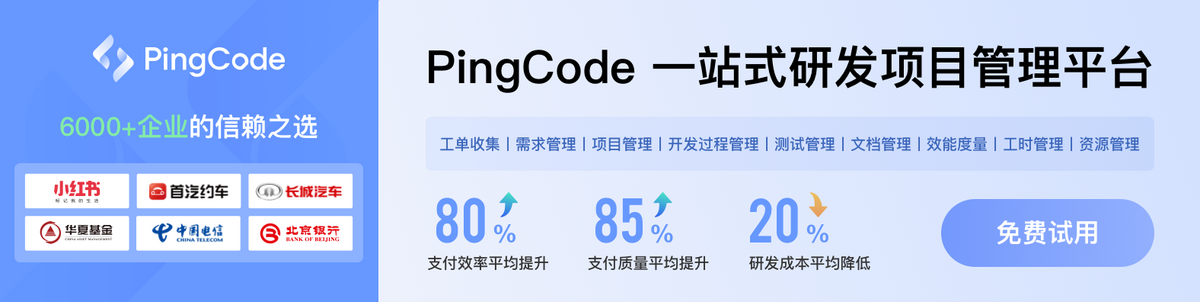