要用Python开发一个象棋游戏,关键步骤包括:定义棋盘和棋子、实现规则和移动、设计用户界面、处理用户输入、及增加人工智能对手。以下将详细描述每个步骤。
一、定义棋盘和棋子
象棋的棋盘是一个9×10的矩形,棋子包括将、士、象、车、马、炮和兵。可以用二维数组表示棋盘,用字典或类定义每个棋子。使用类定义棋子并实现其属性和方法,是一个良好的设计习惯。
棋盘与棋子类设计
首先,我们定义棋盘和棋子的类。棋盘用一个二维数组表示,棋子用类来实现。
class Piece:
def __init__(self, name, color, position):
self.name = name
self.color = color
self.position = position
class Board:
def __init__(self):
self.board = [['' for _ in range(9)] for _ in range(10)]
self.setup_pieces()
def setup_pieces(self):
# 初始化棋子的位置
self.board[0][0] = Piece('车', '红', (0, 0))
self.board[0][8] = Piece('车', '红', (0, 8))
# 按照象棋规则初始化其他棋子
二、实现规则和移动
象棋的每个棋子有不同的移动规则。需要为每种棋子实现单独的移动逻辑。实现棋子的移动规则时,可以借助函数或方法,确保代码的可读性和复用性。
棋子移动规则
我们可以为每种棋子实现一个can_move
方法,判断其移动是否合法。
def can_move(self, start_pos, end_pos):
if self.name == '车':
return self.rook_move(start_pos, end_pos)
elif self.name == '马':
return self.knight_move(start_pos, end_pos)
# 其他棋子的移动规则
def rook_move(self, start_pos, end_pos):
# 车的移动规则
if start_pos[0] == end_pos[0] or start_pos[1] == end_pos[1]:
return True
return False
def knight_move(self, start_pos, end_pos):
# 马的移动规则
if (abs(start_pos[0] - end_pos[0]), abs(start_pos[1] - end_pos[1])) in [(1, 2), (2, 1)]:
return True
return False
三、设计用户界面
用户界面可以用Pygame、Tkinter等库来实现。Pygame是一个流行的选择,因为它提供了丰富的图形和声音支持,适合开发游戏。
使用Pygame设计用户界面
首先,我们初始化Pygame并设置窗口大小。然后,我们需要绘制棋盘和棋子。
import pygame
from pygame.locals import *
pygame.init()
screen = pygame.display.set_mode((540, 600))
pygame.display.set_caption('象棋游戏')
def draw_board():
for row in range(10):
for col in range(9):
pygame.draw.rect(screen, (255, 255, 255), (col*60, row*60, 60, 60))
pygame.draw.rect(screen, (0, 0, 0), (col*60, row*60, 60, 60), 1)
def draw_pieces(board):
for row in range(10):
for col in range(9):
piece = board[row][col]
if piece:
pygame.draw.circle(screen, (255, 0, 0) if piece.color == '红' else (0, 0, 0), (col*60+30, row*60+30), 25)
font = pygame.font.Font(None, 36)
text = font.render(piece.name, True, (255, 255, 255))
screen.blit(text, (col*60+15, row*60+10))
主循环
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 128, 0))
draw_board()
draw_pieces(board)
pygame.display.update()
四、处理用户输入
用户输入包括点击棋盘、选择和移动棋子等。处理用户输入时,需要检测鼠标事件,并转换成棋盘上的位置坐标。
检测用户输入
我们可以在主循环中处理鼠标事件,获取用户点击的位置,并判断是否有效。
selected_piece = None
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == MOUSEBUTTONDOWN:
x, y = event.pos
col, row = x // 60, y // 60
if selected_piece:
if board.can_move(selected_piece.position, (row, col)):
board.move_piece(selected_piece.position, (row, col))
selected_piece = None
else:
selected_piece = board.get_piece((row, col))
screen.fill((0, 128, 0))
draw_board()
draw_pieces(board)
pygame.display.update()
五、增加人工智能对手
增加一个简单的AI对手,可以使游戏更加有趣。AI可以通过Minimax算法或其他策略来实现。
实现简单的AI对手
我们可以实现一个简单的AI,随机选择一个合法的移动。高级AI可以使用Minimax算法和Alpha-Beta剪枝。
import random
def ai_move(board):
possible_moves = board.get_all_possible_moves('黑')
if possible_moves:
move = random.choice(possible_moves)
board.move_piece(move[0], move[1])
在主循环中调用AI
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == MOUSEBUTTONDOWN:
x, y = event.pos
col, row = x // 60, y // 60
if selected_piece:
if board.can_move(selected_piece.position, (row, col)):
board.move_piece(selected_piece.position, (row, col))
selected_piece = None
ai_move(board)
else:
selected_piece = board.get_piece((row, col))
screen.fill((0, 128, 0))
draw_board()
draw_pieces(board)
pygame.display.update()
这样,一个简单的象棋游戏就实现了。通过不断优化棋子类、规则实现和AI算法,可以进一步提升游戏的体验和挑战性。
相关问答FAQs:
如何开始用Python编写象棋游戏?
要开始用Python编写象棋游戏,您需要首先熟悉Python的基本语法和面向对象编程的概念。建议您使用Pygame库来处理图形和用户输入,这样可以更容易地创建游戏界面和棋盘。可以从简单的棋盘绘制开始,然后逐步添加棋子和规则。
实现象棋游戏的主要功能有哪些?
在实现象棋游戏时,主要功能包括棋盘的初始化、棋子的移动规则、捕获对方棋子、悔棋功能和胜负判断等。您还可以考虑实现AI对手,使用简单的算法让电脑能够与玩家对弈。
如何处理象棋游戏中的用户输入?
处理用户输入的方式通常是监听键盘和鼠标事件。在Pygame中,可以通过事件循环来捕捉这些事件。用户可以通过点击棋盘上的棋子来选择,并通过移动鼠标来选择目标位置。确保在移动过程中检查是否合法的移动规则,以提升游戏的可玩性。
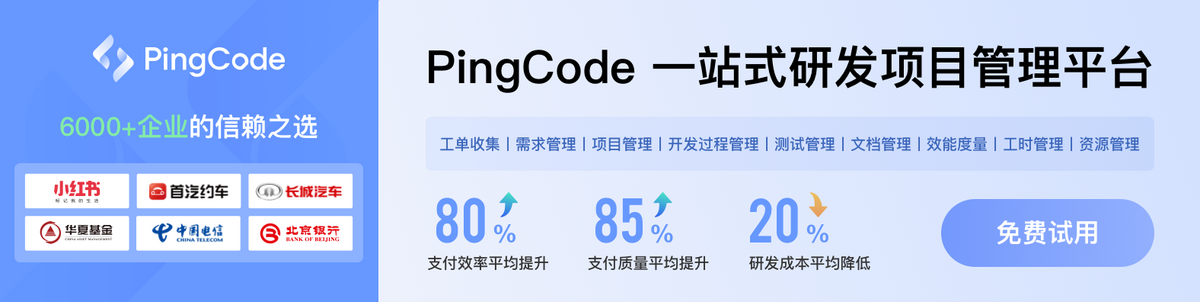