如何取字符串中的元素python
在Python中,取字符串中的元素非常简单,常见的方法包括索引、切片、字符串方法等。索引是最基础的方法,通过指定位置的索引值可以直接获取字符串中的某个字符。切片则可以用于获取字符串的一个子串,指定开始和结束位置。字符串方法(如find()
、split()
)提供了更多高级操作。本文将详细介绍这些方法并提供实际例子。
一、索引
1、正向索引
在Python中,字符串是一个字符序列,每个字符都有一个索引。正向索引从0开始。例如,对于字符串"hello"
,h
的索引是0,e
的索引是1,依此类推。
example_string = "hello"
print(example_string[0]) # 输出: h
print(example_string[1]) # 输出: e
2、负向索引
负向索引允许从字符串的末尾开始计数,最后一个字符的索引是-1
。对于"hello"
,o
的索引是-1
,l
的索引是-2
,依此类推。
example_string = "hello"
print(example_string[-1]) # 输出: o
print(example_string[-2]) # 输出: l
二、切片
1、基本切片
切片允许你通过指定开始和结束索引来获取字符串的子串。语法是string[start:end]
,其中start
是子串的起始位置,end
是结束位置,但不包括该位置的字符。
example_string = "hello"
print(example_string[1:4]) # 输出: ell
2、省略索引
切片的起始或结束索引可以省略,省略起始索引表示从头开始,省略结束索引表示到末尾结束。
example_string = "hello"
print(example_string[:4]) # 输出: hell
print(example_string[2:]) # 输出: llo
3、步长
切片还可以指定步长,默认步长是1。通过步长可以跳跃式地获取子串。
example_string = "hello"
print(example_string[::2]) # 输出: hlo
print(example_string[1:5:2]) # 输出: el
三、字符串方法
1、find()
find()
方法用于查找子字符串在字符串中的位置,返回第一个匹配的索引,如果没有找到则返回-1
。
example_string = "hello world"
print(example_string.find("world")) # 输出: 6
print(example_string.find("Python")) # 输出: -1
2、split()
split()
方法用于将字符串分割成多个子字符串,返回一个列表。默认情况下,分隔符是空格。
example_string = "hello world"
print(example_string.split()) # 输出: ['hello', 'world']
3、replace()
replace()
方法用于替换字符串中的子字符串,返回一个新的字符串。
example_string = "hello world"
print(example_string.replace("world", "Python")) # 输出: hello Python
四、正则表达式
正则表达式提供了更强大的字符串匹配和提取功能。在Python中,可以使用re
模块来进行正则表达式操作。
1、查找所有匹配项
re.findall()
方法用于查找所有匹配的子字符串,返回一个列表。
import re
example_string = "hello 123, hello 456"
matches = re.findall(r"\d+", example_string)
print(matches) # 输出: ['123', '456']
2、替换匹配项
re.sub()
方法用于替换匹配的子字符串。
import re
example_string = "hello 123, hello 456"
new_string = re.sub(r"\d+", "number", example_string)
print(new_string) # 输出: hello number, hello number
3、分组匹配
正则表达式还支持分组匹配,使用小括号()
可以将匹配的子字符串分组。
import re
example_string = "hello 123, hello 456"
pattern = r"(\w+) (\d+)"
matches = re.findall(pattern, example_string)
print(matches) # 输出: [('hello', '123'), ('hello', '456')]
五、总结
通过以上方法,Python提供了丰富的字符串操作功能,能够满足各种需求。索引和切片是最基础的方法,适用于简单的字符提取。字符串方法提供了更多高级操作,如查找、分割和替换。正则表达式则提供了强大的匹配和提取功能,适用于复杂的字符串操作。掌握这些方法可以大大提高你的Python编程效率。
相关问答FAQs:
如何在Python中提取字符串的特定字符?
在Python中,可以通过索引来提取字符串中的特定字符。字符串的索引是从0开始的,因此要提取第一个字符,可以使用string[0]
。如果需要提取多个字符,可以使用切片,例如string[1:4]
将提取从索引1到索引3的字符。
Python中如何使用循环遍历字符串的每个字符?
可以使用for
循环遍历字符串中的每个字符。示例代码如下:
string = "Hello"
for char in string:
print(char)
这段代码将逐个打印出字符串中的每个字符,方便进行更复杂的操作。
在Python中如何查找字符串中某个字符的索引位置?
要找到字符串中某个字符的索引,可以使用string.index('字符')
或string.find('字符')
方法。index()
方法在字符不存在时会引发异常,而find()
方法则返回-1。示例代码如下:
string = "Hello"
index = string.index('e') # 返回1
利用这些方法,可以轻松定位字符在字符串中的位置。
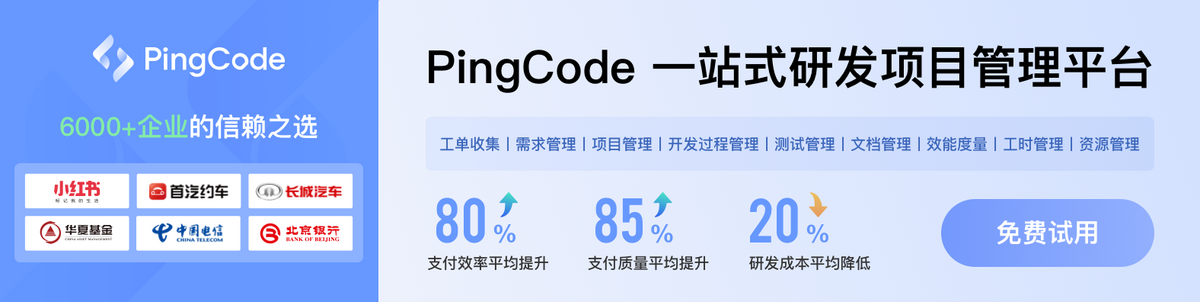